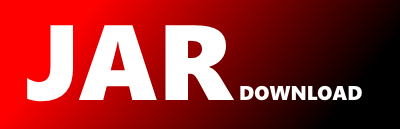
br.com.esec.icpm.util.StreamUtils Maven / Gradle / Ivy
package br.com.esec.icpm.util;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import org.apache.commons.lang.StringUtils;
public class StreamUtils {
public static InputStream load(String path) {
InputStream stream = null;
if (StringUtils.isEmpty(path))
return null;
if (stream == null || isEmpty(stream))
stream = tryGetOnClass(path);
if (stream == null || isEmpty(stream))
stream = tryGetOnClass("/" + path);
if (stream == null || isEmpty(stream))
stream = tryGetOnClassLoader(path);
if (stream == null || isEmpty(stream))
stream = tryGetOnClassLoader("/" + path);
if (stream == null || isEmpty(stream))
stream = tryGetByFilePath(path);
if (stream == null || isEmpty(stream))
stream = tryGetByFilePath("/" + path);
if (stream == null || isEmpty(stream))
stream = tryMountUrl(path);
if (stream == null || isEmpty(stream))
stream = tryMountUrl("/" + path);
if (stream == null || isEmpty(stream))
throw new IllegalStateException("The system can not found the path '" + path + "'.");
return stream;
}
private static boolean isEmpty(InputStream stream) {
try {
return stream.available() <= 0;
} catch (IOException e) {
return false;
}
}
private static InputStream tryGetOnClass(String path) {
try {
return UrlUtils.class.getResourceAsStream(path);
} catch (Exception e) {
return null;
}
}
private static InputStream tryGetOnClassLoader(String path) {
try {
ClassLoader loader = Thread.currentThread().getContextClassLoader();
return loader.getResourceAsStream(path);
} catch (Exception e) {
return null;
}
}
private static InputStream tryGetByFilePath(String path) {
try {
File file = new File(path);
if (!file.exists()) {
return null;
}
return new FileInputStream(file);
} catch (Exception e) {
return null;
}
}
private static InputStream tryMountUrl(String path) {
try {
InputStream stream = new URL(path).openStream();
if (stream.available() > 0)
return stream;
return null;
} catch (IOException e) {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy