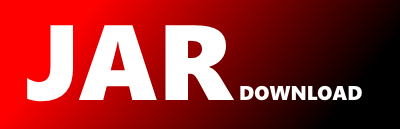
io.katharsis.resource.registry.RegistryEntry Maven / Gradle / Ivy
package io.katharsis.resource.registry;
import io.katharsis.repository.RepositoryMethodParameterProvider;
import io.katharsis.repository.exception.RelationshipRepositoryNotFoundException;
import io.katharsis.resource.information.ResourceInformation;
import io.katharsis.resource.registry.repository.AnnotatedRelationshipEntryBuilder;
import io.katharsis.resource.registry.repository.AnnotatedResourceEntryBuilder;
import io.katharsis.resource.registry.repository.DirectResponseRelationshipEntry;
import io.katharsis.resource.registry.repository.DirectResponseResourceEntry;
import io.katharsis.resource.registry.repository.ResourceEntry;
import io.katharsis.resource.registry.repository.ResponseRelationshipEntry;
import io.katharsis.resource.registry.responseRepository.RelationshipRepositoryAdapter;
import io.katharsis.resource.registry.responseRepository.ResourceRepositoryAdapter;
import java.util.LinkedList;
import java.util.List;
import java.util.Objects;
/**
* Holds information about a resource of type T and its repositories.
* It includes the following information:
* - ResourceInformation instance with information about the resource,
* - ResourceEntry instance,
* - List of all repositories for relationships defined in resource class.
* - Parent RegistryEntry if a resource inherits from another resource
*
* @param resource type
*/
public class RegistryEntry {
private final ResourceInformation resourceInformation;
private final ResourceEntry resourceEntry;
private final List> relationshipEntries;
private RegistryEntry parentRegistryEntry = null;
private ResourceRegistry resourceRegistry;
public RegistryEntry(ResourceInformation resourceInformation,
@SuppressWarnings("SameParameterValue") ResourceEntry resourceEntry) {
this(resourceInformation, resourceEntry, new LinkedList>());
}
public RegistryEntry(ResourceInformation resourceInformation,
ResourceEntry resourceEntry,
List> relationshipEntries) {
this.resourceInformation = resourceInformation;
this.resourceEntry = resourceEntry;
this.relationshipEntries = relationshipEntries;
}
protected void initialize(ResourceRegistry resourceRegistry){
this.resourceRegistry = resourceRegistry;
}
@SuppressWarnings("unchecked")
public ResourceRepositoryAdapter getResourceRepository(RepositoryMethodParameterProvider parameterProvider) {
Object repoInstance = null;
if (resourceEntry instanceof DirectResponseResourceEntry) {
repoInstance = ((DirectResponseResourceEntry) resourceEntry).getResourceRepository();
} else if (resourceEntry instanceof AnnotatedResourceEntryBuilder) {
repoInstance = ((AnnotatedResourceEntryBuilder) resourceEntry).build(parameterProvider);
}
if(repoInstance instanceof ResourceRegistryAware){
((ResourceRegistryAware)repoInstance).setResourceRegistry(resourceRegistry);
}
return new ResourceRepositoryAdapter(repoInstance);
}
public List> getRelationshipEntries() {
return relationshipEntries;
}
@SuppressWarnings("unchecked")
public RelationshipRepositoryAdapter getRelationshipRepositoryForClass(Class clazz,
RepositoryMethodParameterProvider parameterProvider) {
ResponseRelationshipEntry foundRelationshipEntry = null;
for (ResponseRelationshipEntry relationshipEntry : relationshipEntries) {
if (clazz == relationshipEntry.getTargetAffiliation()) {
foundRelationshipEntry = relationshipEntry;
break;
}
}
if (foundRelationshipEntry == null) {
throw new RelationshipRepositoryNotFoundException(resourceInformation.getResourceClass(), clazz);
}
Object repoInstance;
if (foundRelationshipEntry instanceof AnnotatedRelationshipEntryBuilder) {
repoInstance = ((AnnotatedRelationshipEntryBuilder) foundRelationshipEntry).build(parameterProvider);
} else {
repoInstance = ((DirectResponseRelationshipEntry) foundRelationshipEntry).getRepositoryInstanceBuilder();
}
if(repoInstance instanceof ResourceRegistryAware){
((ResourceRegistryAware)repoInstance).setResourceRegistry(resourceRegistry);
}
return new RelationshipRepositoryAdapter(repoInstance);
}
public ResourceInformation getResourceInformation() {
return resourceInformation;
}
public RegistryEntry getParentRegistryEntry() {
return parentRegistryEntry;
}
/**
* To be used only by ResourceRegistryBuilder
*
* @param parentRegistryEntry parent resource
*/
void setParentRegistryEntry(RegistryEntry parentRegistryEntry) {
this.parentRegistryEntry = parentRegistryEntry;
}
/**
* Check the parameter is a parent of this {@link RegistryEntry} instance
*
* @param registryEntry parent to check
* @return true if the parameter is a parent
*/
public boolean isParent(RegistryEntry registryEntry) {
RegistryEntry parentRegistryEntry = getParentRegistryEntry();
while (parentRegistryEntry != null) {
if (parentRegistryEntry.equals(registryEntry)) {
return true;
}
parentRegistryEntry = parentRegistryEntry.getParentRegistryEntry();
}
return false;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
RegistryEntry> that = (RegistryEntry>) o;
return Objects.equals(resourceInformation, that.resourceInformation) &&
Objects.equals(resourceEntry, that.resourceEntry) &&
Objects.equals(relationshipEntries, that.relationshipEntries) &&
Objects.equals(parentRegistryEntry, that.parentRegistryEntry);
}
@Override
public int hashCode() {
return Objects.hash(resourceInformation, resourceEntry, relationshipEntries, parentRegistryEntry);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy