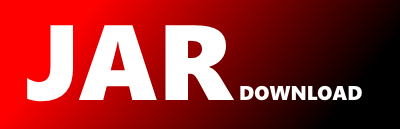
br.com.jarch.apt.generate.DataXhtmlCodeGenerate Maven / Gradle / Ivy
package br.com.jarch.apt.generate;
import br.com.jarch.annotation.JArchGenerateLogicCrud;
import br.com.jarch.annotation.JArchGenerateLogicMasterDetail;
import br.com.jarch.annotation.JArchGenerateLogicMasterSubDetail;
import br.com.jarch.form.FieldForm;
import br.com.jarch.util.FileUtils;
import br.com.jarch.util.LogUtils;
import br.com.jarch.util.ProcessorUtils;
import br.com.jarch.util.type.FieldType;
import javax.lang.model.element.Element;
import java.io.File;
import java.io.IOException;
import java.util.Arrays;
import java.util.Comparator;
import java.util.List;
import java.util.stream.Collectors;
import static br.com.jarch.util.ProcessorUtils.addCode;
public final class DataXhtmlCodeGenerate {
final String ENTITY = "entity";
final String DATA_DETAIL = "dataDetail";
final String DOT_ENTITY = ".entity";
private Element element;
private JArchGenerateLogicCrud generateLogicCrud;
private JArchGenerateLogicMasterDetail generateLogicMasterDetail;
private JArchGenerateLogicMasterSubDetail generateLogicMasterSubDetail;
private File fileDataXhtml;
private File fileDadosXhtml;
private String nameMaster;
private String nameMasterSemCaracterEspecial;
private String nameMasterSemCaracterEspecialMinusculo;
private String nameController;
public DataXhtmlCodeGenerate(Element element, JArchGenerateLogicCrud generateLogicCrud) {
this.element = element;
this.generateLogicCrud = generateLogicCrud;
configure(element, generateLogicCrud.master().name(), generateLogicCrud.nameSubPackage());
}
public DataXhtmlCodeGenerate(Element element, JArchGenerateLogicMasterDetail generateLogicMasterDetail) {
this.element = element;
this.generateLogicMasterDetail = generateLogicMasterDetail;
configure(element, generateLogicMasterDetail.master().name(), generateLogicMasterDetail.nameSubPackage());
}
public DataXhtmlCodeGenerate(Element element, JArchGenerateLogicMasterSubDetail generateLogicMasterSubDetail) {
this.element = element;
this.generateLogicMasterSubDetail = generateLogicMasterSubDetail;
configure(element, generateLogicMasterSubDetail.master().name(), generateLogicMasterSubDetail.nameSubPackage());
}
public void create() {
if (ProcessorUtils.isFileExistsClientOrWebOrWs(fileDataXhtml) || ProcessorUtils.isFileExistsClientOrWebOrWs(fileDadosXhtml)) {
return;
}
StringBuilder dataXhtml = new StringBuilder();
addCode(dataXhtml, "");
addCode(dataXhtml, "");
addCode(dataXhtml, "\t");
addCode(dataXhtml, "\t\t ");
addCode(dataXhtml, "\t ");
addCode(dataXhtml, "");
addCode(dataXhtml, "\t");
addCode(dataXhtml, "\t\t");
addCode(dataXhtml, "\t\t\t ");
addCode(dataXhtml, "\t\t\t");
if (generateLogicCrud != null) {
addCode(dataXhtml, generateXhtmlFromFieldsMaster(nameMaster, ProcessorUtils.getListFieldForm(generateLogicCrud.master().fields()), ENTITY, ""));
}
if (generateLogicMasterDetail != null) {
addCode(dataXhtml, generateXhtmlFromFieldsMaster(nameMaster, ProcessorUtils.getListFieldForm(generateLogicMasterDetail.master().fields()), ENTITY, ""));
}
if (generateLogicMasterSubDetail != null) {
addCode(dataXhtml, generateXhtmlFromFieldsMaster(nameMaster, ProcessorUtils.getListFieldForm(generateLogicMasterSubDetail.master().fields()), ENTITY, ""));
}
addCode(dataXhtml, "\t\t\t ");
if (generateLogicMasterDetail != null) {
addDivDataDetail(dataXhtml);
addCode(dataXhtml, "\t\t\t\t\t");
Arrays
.stream(generateLogicMasterDetail.details())
.forEach(d -> {
String nameSemCaracterEspecial = ProcessorUtils.getNameWithoutCharEspecialCapitalize(d.name());
String nameSemCaracterEspecialMinusculo = ProcessorUtils.getNameWithoutCharEspecialStartLowerCase(d.name());
String entity = DATA_DETAIL + nameSemCaracterEspecial + DOT_ENTITY;
String dataDetail = DATA_DETAIL + nameSemCaracterEspecial;
String actionDataDetail = nameController + "." + dataDetail;
addCode(dataXhtml, "" +
"\t\t\t\t\t\t\n" +
"\t\t\t\t\t\t\t\n" +
"\t\t\t\t\t\t\t\t \n" +
"\t\t\t\t\t\t\t \n" +
"\t\t\t\t\t\t\t");
addCode(dataXhtml, generateXhtmlFromFieldsDetail(d.name(), ProcessorUtils.getListFieldForm(d.fields()), entity, dataDetail));
addCode(dataXhtml, "\t\t\t\t\t\t\t ");
addCode(dataXhtml, "\t\t\t\t\t\t ");
});
addCode(dataXhtml, "\t\t\t\t\t ");
addCloseDivDataDetail(dataXhtml);
}
if (generateLogicMasterSubDetail != null) {
addDivDataDetail(dataXhtml);
addCode(dataXhtml, "\t\t\t\t\t");
Arrays
.stream(generateLogicMasterSubDetail.details())
.forEach(detail -> {
String nameSemCaracterEspecial = ProcessorUtils.getNameWithoutCharEspecialCapitalize(detail.name());
String nameSemCaracterEspecialMinusculo = ProcessorUtils.getNameWithoutCharEspecialStartLowerCase(detail.name());
String entity = DATA_DETAIL + nameSemCaracterEspecial + DOT_ENTITY;
String dataDetail = DATA_DETAIL + nameSemCaracterEspecial;
String actionDataDetail = nameController + "." + dataDetail;
addCode(dataXhtml, "" +
"\t\t\t\t\t\t\n" +
"\t\t\t\t\t\t\t\n" +
"\t\t\t\t\t\t\t\t \n" +
"\t\t\t\t\t\t\t \n" +
"\t\t\t\t\t\t\t");
addCode(dataXhtml, generateXhtmlFromFieldsDetail(detail.name(), ProcessorUtils.getListFieldForm(detail.fields()), entity, dataDetail));
Arrays
.stream(detail.subDetails())
.forEach(subDetail -> {
String nameSubDetalheSemCaracterEspecial = ProcessorUtils.getNameWithoutCharEspecialCapitalize(subDetail.name());
String nameSubDetalheSemCaracterEspecialMinusculo = ProcessorUtils.getNameWithoutCharEspecialStartLowerCase(subDetail.name());
String entitySubDetalhe = DATA_DETAIL + nameSubDetalheSemCaracterEspecial + DOT_ENTITY;
String dataDetailSubDetalhe = DATA_DETAIL + nameSubDetalheSemCaracterEspecial;
String actionDataDetailSubDetalhe = nameController + "." + dataDetailSubDetalhe;
addCode(dataXhtml, "" +
"\t\t\t\t\t\t\t\t\n" +
"\t\t\t\t\t\t\t\t\t\n" +
"\t\t\t\t\t\t\t\t\t\t\n" +
"\t\t\t\t\t\t\t\t\t\t\t\n" +
"\t\t\t\t\t\t\t\t\t\t\t\t \n" +
"\t\t\t\t\t\t\t\t\t\t\t \n" +
"\t\t\t\t\t\t\t\t\t\t\t");
addCode(dataXhtml, generateXhtmlFromFieldsSubDetail(subDetail.name(), ProcessorUtils.getListFieldForm(subDetail.fields()), entitySubDetalhe, dataDetailSubDetalhe));
addCode(dataXhtml, "\t\t\t\t\t\t\t\t\t\t\t ");
addCode(dataXhtml, "\t\t\t\t\t\t\t\t\t\t ");
addCode(dataXhtml, "\t\t\t\t\t\t\t\t\t ");
addCode(dataXhtml, "\t\t\t\t\t\t\t\t ");
}
);
addCode(dataXhtml, "\t\t\t\t\t\t\t ");
addCode(dataXhtml, "\t\t\t\t\t\t ");
});
addCode(dataXhtml, "\t\t\t\t\t ");
addCloseDivDataDetail(dataXhtml);
}
addCode(dataXhtml, "\t\t ");
addCode(dataXhtml, "\t ");
addCode(dataXhtml, " ");
addCode(dataXhtml, "");
try {
FileUtils.save(fileDataXhtml, dataXhtml.toString());
ProcessorUtils.messageNote("JARCH Created ==> " + fileDataXhtml.getAbsoluteFile(), element);
} catch (IOException e) {
LogUtils.generate(e);
}
}
private void addCloseDivDataDetail(StringBuilder sbListaXhtml) {
addCode(sbListaXhtml, "\t\t\t\t");
addCode(sbListaXhtml, "\t\t\t");
}
private void addDivDataDetail(StringBuilder sbListaXhtml) {
addCode(sbListaXhtml, "\t\t\t");
addCode(sbListaXhtml, "\t\t\t\t");
}
private String generateXhtmlFromFieldsMaster(String name, List formFields, String entity, String dataDetail) {
return generateXhtmlFromFields(TabXhtmlType.MASTER, name, formFields, entity, dataDetail);
}
private String generateXhtmlFromFieldsDetail(String name, List formFields, String entity, String dataDetail) {
return generateXhtmlFromFields(TabXhtmlType.DETAIL, name, formFields, entity, dataDetail);
}
private String generateXhtmlFromFieldsSubDetail(String name, List formFields, String entity, String dataDetail) {
return generateXhtmlFromFields(TabXhtmlType.SUBDETAIL, name, formFields, entity, dataDetail);
}
private String generateXhtmlFromFields(TabXhtmlType tabXhtmlType, String name, List formFields, String entity, String dataDetail) {
boolean master = name == nameMaster;
StringBuilder result = new StringBuilder();
int maiorLinha = formFields
.stream()
.mapToInt(f -> f.getXhtml().stream().mapToInt(l -> l.getRowDataXhtml()).max().orElse(0))
.max()
.orElse(0);
for (int linha = 1; linha <= maiorLinha; linha++) {
int linhaComparacao = linha;
List formFieldsOrderByRowColumn = formFields
.stream()
.filter(f -> !f.getXhtml().isEmpty())
.filter(f -> f.getXhtml().stream().allMatch(l -> l.getRowDataXhtml() == linhaComparacao))
.sorted(Comparator.comparing((FieldForm f) -> f.getXhtml().get(0).getColumnDataXhtml()))
.collect(Collectors.toList());
if (formFieldsOrderByRowColumn.isEmpty()) {
continue;
}
String tabsDentro = tabXhtmlType.tabs();
String stylePanelGrid = "";
if (formFieldsOrderByRowColumn.stream().anyMatch(f -> f.getTipo() == FieldType.ADDRESS)) {
stylePanelGrid = " style=\"width: 100%\"";
}
result.append(tabsDentro + "\n");
String fechandoTagPanelGrid = tabsDentro + " ";
tabsDentro += "\t";
for (FieldForm campoForm : formFieldsOrderByRowColumn) {
result.append(tabsDentro);
result.append("\n");
if (!FieldType.ENTITY.equals(campoForm.getTipo())
&& !FieldType.MONEY.equals(campoForm.getTipo())
&& !campoForm.getTipo().isAddress()) {
result.append(tabsDentro + "\t \n");
result.append(tabsDentro + "\t
\n");
}
result.append(tabsDentro + "\t");
result.append(campoForm.getTagXhtml(master, nameController, nameMasterSemCaracterEspecial, dataDetail, entity, tabsDentro));
result.append(tabsDentro + " \n");
}
result.append(fechandoTagPanelGrid + "\n");
}
return result.toString();
}
private void configure(Element element, String nameMaster, String nameSubPackage) {
this.nameMaster = nameMaster;
nameMasterSemCaracterEspecial = ProcessorUtils.getNameWithoutCharEspecialCapitalize(nameMaster);
nameMasterSemCaracterEspecialMinusculo = ProcessorUtils.getNameWithoutCharEspecialStartLowerCase(nameMaster);
nameController = nameMasterSemCaracterEspecialMinusculo + "DataController";
File folder = new File(ProcessorUtils.getPathPage(element, nameSubPackage));
if (!folder.exists()) {
folder.mkdir();
}
fileDataXhtml = new File(ProcessorUtils.getPathPage(element, nameSubPackage) + nameMasterSemCaracterEspecialMinusculo + "Data.xhtml");
fileDadosXhtml = new File(ProcessorUtils.getPathPage(element, nameSubPackage) + "dados" + nameMasterSemCaracterEspecial + ".xhtml");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy