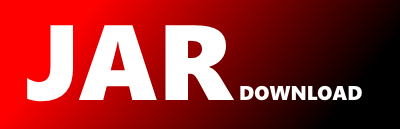
br.com.jarch.apt.generate.EntityCodeGenerate Maven / Gradle / Ivy
package br.com.jarch.apt.generate;
import br.com.jarch.annotation.*;
import br.com.jarch.form.FieldForm;
import br.com.jarch.util.FileUtils;
import br.com.jarch.util.LogUtils;
import br.com.jarch.util.ProcessorUtils;
import br.com.jarch.util.type.FieldType;
import javax.lang.model.element.Element;
import java.io.File;
import java.io.IOException;
import java.util.*;
import java.util.stream.Collectors;
import static br.com.jarch.util.ProcessorUtils.addCode;
import static br.com.jarch.util.ProcessorUtils.addLineBlank;
public final class EntityCodeGenerate {
private Element element;
private JArchGenerateLogicCrud generateLogicCrud;
private JArchGenerateLogicMasterDetail generateLogicMasterDetail;
private JArchGenerateLogicMasterSubDetail generateLogicMasterSubDetail;
private String nameSubPackage;
private boolean secret;
private boolean exclusionLogic;
// private File folder;
private File folderScript;
private String namePackage;
private Set imports = new HashSet<>();
private List columnsDataTable = new ArrayList<>();
private List fields = new ArrayList<>();
private List getSets = new ArrayList<>();
private List getSetEnums = new ArrayList<>();
public EntityCodeGenerate(Element element, JArchGenerateLogicCrud generateLogicCrud) {
this.element = element;
this.generateLogicCrud = generateLogicCrud;
this.nameSubPackage = generateLogicCrud.nameSubPackage();
this.secret = generateLogicCrud.secret();
this.exclusionLogic = generateLogicCrud.exclusionLogic();
configEnviroment();
}
public EntityCodeGenerate(Element element, JArchGenerateLogicMasterDetail generateLogicMasterDetail) {
this.element = element;
this.generateLogicMasterDetail = generateLogicMasterDetail;
this.nameSubPackage = generateLogicMasterDetail.nameSubPackage();
this.secret = generateLogicMasterDetail.secret();
this.exclusionLogic = generateLogicMasterDetail.exclusionLogic();
configEnviroment();
}
public EntityCodeGenerate(Element element, JArchGenerateLogicMasterSubDetail generateLogicMasterSubDetail) {
this.element = element;
this.generateLogicMasterSubDetail = generateLogicMasterSubDetail;
this.nameSubPackage = generateLogicMasterSubDetail.nameSubPackage();
this.secret = generateLogicMasterSubDetail.secret();
this.exclusionLogic = generateLogicMasterSubDetail.exclusionLogic();
configEnviroment();
}
public void create() {
createLogiCrud();
createLogicMasterDetail();
createLogicMasterSubDetail();
}
private void createLogiCrud() {
if (generateLogicCrud == null)
return;
List fieldForms = ProcessorUtils.getListFieldForm(generateLogicCrud.master().fields());
loadImportsFieldsGetsSetsEnums(fieldForms, imports, fields, columnsDataTable, getSets, getSetEnums);
String codeLookup = fieldForms.stream().filter(FieldForm::isCodeLookup).map(FieldForm::getAtributo).findAny().orElse(null);
String descriptionLookup = fieldForms.stream().filter(FieldForm::isDescriptionLookup).map(FieldForm::getAtributo).findAny().orElse(null);
String name = generateLogicCrud.master().name();
String tableName = generateLogicCrud.master().tableName();
saveEntity(name, tableName, imports, fields, getSets, getSetEnums, "", new ArrayList<>(), codeLookup,
descriptionLookup, fieldForms);
saveEnums(fieldForms);
saveEnderecos(fieldForms);
generateScriptDataBase(name, tableName, fieldForms, null);
}
private void createLogicMasterDetail() {
if (generateLogicMasterDetail == null)
return;
List fieldForms = ProcessorUtils.getListFieldForm(generateLogicMasterDetail.master().fields());
loadImportsFieldsGetsSetsEnums(fieldForms, imports, fields, columnsDataTable, getSets, getSetEnums);
String nameMaster = generateLogicMasterDetail.master().name();
String tableName = generateLogicMasterDetail.master().tableName();
saveEntity(nameMaster, tableName, imports, fields, getSets, getSetEnums, null,
Arrays.stream(generateLogicMasterDetail.details()).map(JArchGenerateDetail::name).collect(Collectors.toList()),
fieldForms.stream().filter(FieldForm::isCodeLookup).map(FieldForm::getAtributo).findAny().orElse(null),
fieldForms.stream().filter(FieldForm::isDescriptionLookup).map(FieldForm::getAtributo).findAny().orElse(null), fieldForms);
saveEnums(fieldForms);
saveEnderecos(fieldForms);
generateScriptDataBase(nameMaster, tableName, fieldForms, null);
generateDetailFromMasterDetail(nameMaster, tableName);
}
private void generateDetailFromMasterDetail(String nameMaster, String tableName) {
List fieldForms;
for (int i = 0; i < generateLogicMasterDetail.details().length; i++) {
fieldForms = ProcessorUtils.getListFieldForm(generateLogicMasterDetail.details()[i].fields());
loadImportsFieldsGetsSetsEnums(fieldForms, imports, fields, columnsDataTable, getSets, getSetEnums);
String nameDetail = generateLogicMasterDetail.details()[i].name();
String tableNameDetail = generateLogicMasterDetail.details()[i].tableName();
String codeLookup = fieldForms.stream().filter(FieldForm::isCodeLookup).map(FieldForm::getAtributo).findAny().orElse(null);
String descriptionLookup = fieldForms.stream().filter(FieldForm::isDescriptionLookup).map(FieldForm::getAtributo).findAny().orElse(null);
saveEntity(nameDetail, tableNameDetail, imports, fields, getSets, getSetEnums, nameMaster, new ArrayList<>(),
codeLookup, descriptionLookup, fieldForms);
saveEnums(fieldForms);
saveEnderecos(fieldForms);
generateScriptDataBase(nameDetail, tableNameDetail, fieldForms, tableName);
SearchCodeGenerate.generate(element, nameDetail, generateLogicMasterDetail.nameSubPackage());
JpqlBuilderCodeGenerate.generate(element, nameDetail, generateLogicMasterDetail.nameSubPackage());
ServiceCodeGenerate.generate(element, nameDetail, generateLogicMasterDetail.nameSubPackage());
RepositoryCodeGenerate.generate(element, nameDetail, generateLogicMasterDetail.nameSubPackage());
DaoCodeGenerate.generate(element, nameDetail, generateLogicMasterDetail.nameSubPackage());
}
}
private void createLogicMasterSubDetail() {
if (generateLogicMasterSubDetail == null)
return;
List fieldForms = ProcessorUtils.getListFieldForm(generateLogicMasterSubDetail.master().fields());
loadImportsFieldsGetsSetsEnums(fieldForms, imports, fields, columnsDataTable, getSets, getSetEnums);
String nameMaster = generateLogicMasterSubDetail.master().name();
String tableNameMaster = generateLogicMasterSubDetail.master().tableName();
List details = Arrays.stream(generateLogicMasterSubDetail.details()).map(JArchGenerateDetailSubDetail::name).collect(Collectors.toList());
String codeLookup = fieldForms.stream().filter(FieldForm::isCodeLookup).map(FieldForm::getAtributo).findAny().orElse(null);
String descriptionLookup = fieldForms.stream().filter(FieldForm::isDescriptionLookup).map(FieldForm::getAtributo).findAny().orElse(null);
saveEntity(nameMaster, tableNameMaster, imports, fields, getSets, getSetEnums, null, details, codeLookup,
descriptionLookup, fieldForms);
saveEnums(fieldForms);
saveEnderecos(fieldForms);
generateScriptDataBase(nameMaster, tableNameMaster, fieldForms, null);
generateDetailFromMasterDetailSubDetail(nameMaster, tableNameMaster);
}
private void generateDetailFromMasterDetailSubDetail(String nameMaster, String tableNameMaster) {
List fieldForms;
for (int i = 0; i < generateLogicMasterSubDetail.details().length; i++) {
fieldForms = ProcessorUtils.getListFieldForm(generateLogicMasterSubDetail.details()[i].fields());
loadImportsFieldsGetsSetsEnums(fieldForms, imports, fields, columnsDataTable, getSets, getSetEnums);
String nameDetail = generateLogicMasterSubDetail.details()[i].name();
String tableNameDetail = generateLogicMasterSubDetail.details()[i].tableName();
List details = Arrays.stream(generateLogicMasterSubDetail.details()[i].subDetails()).map(JArchGenerateSubDetail::name).collect(Collectors.toList());
String codeLookup = fieldForms.stream().filter(FieldForm::isCodeLookup).map(FieldForm::getAtributo).findAny().orElse(null);
String descriptionLookup = fieldForms.stream().filter(FieldForm::isDescriptionLookup).map(FieldForm::getAtributo).findAny().orElse(null);
saveEntity(nameDetail, tableNameDetail, imports, fields, getSets, getSetEnums, nameMaster, details,
codeLookup, descriptionLookup, fieldForms);
saveEnums(fieldForms);
saveEnderecos(fieldForms);
generateScriptDataBase(nameDetail, tableNameDetail, fieldForms, tableNameMaster);
SearchCodeGenerate.generate(element, nameDetail, generateLogicMasterSubDetail.nameSubPackage());
JpqlBuilderCodeGenerate.generate(element, nameDetail, generateLogicMasterSubDetail.nameSubPackage());
ServiceCodeGenerate.generate(element, nameDetail, generateLogicMasterSubDetail.nameSubPackage());
RepositoryCodeGenerate.generate(element, nameDetail, generateLogicMasterSubDetail.nameSubPackage());
DaoCodeGenerate.generate(element, nameDetail, generateLogicMasterSubDetail.nameSubPackage());
generateSubDetailFromMasterDetailSubDetail(i, nameDetail, tableNameDetail);
}
}
private void generateSubDetailFromMasterDetailSubDetail(int i, String nameDetail, String tableNameDetail) {
List fieldForms;
for (int j = 0; j < generateLogicMasterSubDetail.details()[i].subDetails().length; j++) {
fieldForms = ProcessorUtils.getListFieldForm(generateLogicMasterSubDetail.details()[i].subDetails()[j].fields());
loadImportsFieldsGetsSetsEnums(fieldForms, imports, fields, columnsDataTable, getSets, getSetEnums);
String nameSubDetail = generateLogicMasterSubDetail.details()[i].subDetails()[j].name();
String tableNameSubDetail = generateLogicMasterSubDetail.details()[i].subDetails()[j].tableName();
saveEntity(nameSubDetail, tableNameSubDetail, imports, fields, getSets, getSetEnums, nameDetail,
new ArrayList<>(),
fieldForms.stream().filter(FieldForm::isCodeLookup).map(FieldForm::getAtributo).findAny().orElse(null),
fieldForms.stream().filter(FieldForm::isDescriptionLookup).map(FieldForm::getAtributo).findAny().orElse(null),
fieldForms);
saveEnums(fieldForms);
saveEnderecos(fieldForms);
generateScriptDataBase(nameSubDetail, tableNameSubDetail, fieldForms, tableNameDetail);
SearchCodeGenerate.generate(element, nameSubDetail, generateLogicMasterSubDetail.nameSubPackage());
JpqlBuilderCodeGenerate.generate(element, nameSubDetail, generateLogicMasterSubDetail.nameSubPackage());
ServiceCodeGenerate.generate(element, nameSubDetail, generateLogicMasterSubDetail.nameSubPackage());
RepositoryCodeGenerate.generate(element, nameSubDetail, generateLogicMasterSubDetail.nameSubPackage());
DaoCodeGenerate.generate(element, nameSubDetail, generateLogicMasterSubDetail.nameSubPackage());
}
}
private void saveEntity(String name, String tableName, Set imports, List fields,
List getSets, List getSetEnums, String nameMaster, List details,
String codeLookup, String descriptionLookup, List fieldForms) {
String nomeSemCaracterEspecial = ProcessorUtils.getNameWithoutCharEspecialCapitalize(name);
String nomeSemCaracterEspecialMinusculo = ProcessorUtils.getNameWithoutCharEspecialStartLowerCase(name);
String nameMasterSemCaracterEspecial = ProcessorUtils.getNameWithoutCharEspecialCapitalize(nameMaster);
String nameMasterSemCaracterEspecialMinusculo = ProcessorUtils.getNameWithoutCharEspecialStartLowerCase(nameMaster);
String nomeSequenceGenerator = nomeSemCaracterEspecial + "IdSequence";
addImports(imports, nameMaster, details);
if (codeLookup != null && descriptionLookup != null && !codeLookup.isEmpty() && !descriptionLookup.isEmpty()) {
imports.add("import br.com.jarch.annotation.JArchLookup;");
}
StringBuilder sbEntity = new StringBuilder();
configurePackage(sbEntity);
configureImport(imports, sbEntity);
configureClass(tableName, codeLookup, descriptionLookup, nomeSemCaracterEspecial, nomeSemCaracterEspecialMinusculo, nomeSequenceGenerator, sbEntity, fieldForms);
configureFieldId(tableName, nomeSequenceGenerator, sbEntity);
configureRelationMaster(nameMaster, nameMasterSemCaracterEspecial, nameMasterSemCaracterEspecialMinusculo, sbEntity);
configureFields(fields, details, nomeSemCaracterEspecialMinusculo, sbEntity);
configureConstructors(fieldForms, nomeSemCaracterEspecial, sbEntity);
configureGetSet(getSets, getSetEnums, nameMaster, details, nameMasterSemCaracterEspecial, nameMasterSemCaracterEspecialMinusculo, sbEntity);
addCode(sbEntity, "}");
File fileEntity = new File(ProcessorUtils.getPathSource(element, nameSubPackage) + nomeSemCaracterEspecial + "Entity.java");
saveFile(fileEntity, sbEntity.toString());
}
private void configureConstructors(List fieldForms, String nomeSemCaracterEspecial, StringBuilder sbEntity) {
Optional fieldFormAddress = fieldForms
.stream()
.filter(f -> f.getTipo().isAddress())
.findAny();
addCode(sbEntity, "\tpublic " + nomeSemCaracterEspecial + "Entity() {");
if (fieldFormAddress.isPresent()) {
fieldForms
.stream()
.filter(f -> f.getTipo().isAddress())
.forEach(f -> addCode(sbEntity, "\t\tthis." + f.getAtributo() + " = new " + f.getAtributoPrimeiroLetraMaiusculo() + "Embeddable();"));
}
addCode(sbEntity, "\t}");
addLineBlank(sbEntity);
if (!columnsDataTable.isEmpty()) {
addCode(sbEntity, "\tpublic " + nomeSemCaracterEspecial + "Entity(Long id, " + columnsDataTable.stream().collect(Collectors.joining(", ")) + ") {");
addCode(sbEntity, "\t\tthis.id = id;");
columnsDataTable.forEach(c -> addCode(sbEntity, "\t\tthis." + c.substring(c.indexOf(" ") + 1).concat(" = ").concat(c.substring(c.indexOf(" ") + 1)).concat(";")));
addCode(sbEntity, "\t}");
addLineBlank(sbEntity);
}
}
private void configureGetSet(List getSets, List getSetEnums, String nameMaster, List details, String nameMasterSemCaracterEspecial, String nameMasterSemCaracterEspecialMinusculo, StringBuilder sbEntity) {
getSetId(sbEntity);
getSetMaster(nameMaster, nameMasterSemCaracterEspecial, nameMasterSemCaracterEspecialMinusculo, sbEntity);
getSets.forEach(getSet -> {
addCode(sbEntity, getSet);
addLineBlank(sbEntity);
});
details.forEach(detail -> {
String nameWithoutCharEspecialCapitalize = ProcessorUtils.getNameWithoutCharEspecialCapitalize(detail);
addCode(sbEntity, "" +
"\tpublic Set<" + nameWithoutCharEspecialCapitalize + "Entity> getLista" + nameWithoutCharEspecialCapitalize + "() {\n" +
"\t\treturn lista" + nameWithoutCharEspecialCapitalize + "; \n" +
"\t}\n" +
"\n" +
"\tpublic void setLista" + nameWithoutCharEspecialCapitalize + "(Set<" + nameWithoutCharEspecialCapitalize + "Entity> lista" + nameWithoutCharEspecialCapitalize + ") { \n" +
"\t\tthis.lista" + nameWithoutCharEspecialCapitalize + " = lista" + nameWithoutCharEspecialCapitalize + "; \n" +
"\t}\n" +
"\n" +
"\tpublic void addLista" + nameWithoutCharEspecialCapitalize + "(" + nameWithoutCharEspecialCapitalize + "Entity " + ProcessorUtils.getNameWithoutCharEspecialStartLowerCase(detail) + ") { \n" +
"\t\tif (lista" + nameWithoutCharEspecialCapitalize + " == null) {\n" +
"\t\t\tlista" + nameWithoutCharEspecialCapitalize + " = new HashSet<>();\n" +
"\t\t}\n" +
"\n" +
"\t\tlista" + nameWithoutCharEspecialCapitalize + ".add(" + ProcessorUtils.getNameWithoutCharEspecialStartLowerCase(detail) + ");\n" +
"\t}");
addLineBlank(sbEntity);
});
getSetEnums.forEach(getSetEnum -> {
addCode(sbEntity, getSetEnum);
addLineBlank(sbEntity);
});
}
private void getSetId(StringBuilder sbEntity) {
addCode(sbEntity, "\t@Override");
addCode(sbEntity, "\tpublic Long getId() {");
addCode(sbEntity, "\t\treturn id;");
addCode(sbEntity, "\t}");
addLineBlank(sbEntity);
addCode(sbEntity, "\t@Override");
addCode(sbEntity, "\tpublic void setId(Long id) {");
addCode(sbEntity, "\t\tthis.id = id;");
addCode(sbEntity, "\t}");
addLineBlank(sbEntity);
}
private void configureFields(List fields, List details, String nomeSemCaracterEspecialMinusculo, StringBuilder sbEntity) {
fields.forEach(f -> {
addCode(sbEntity, f);
addLineBlank(sbEntity);
});
details.forEach(d -> {
addCode(sbEntity, "\t@OneToMany(cascade = CascadeType.ALL, mappedBy = \"" + nomeSemCaracterEspecialMinusculo + "\", orphanRemoval = true)\n"
+ "\t@JArchValidRequired(\"label." + ProcessorUtils.getNameWithoutCharEspecialStartLowerCase(d) + "\") \n"
+ "\t@JArchNoCloneId\n"
+ "\t@Filter(name = Constant.TENANT)\n"
+ (exclusionLogic ? "\t@JArchExclusionLogic\n" : "")
+ "\tprivate Set<" + ProcessorUtils.getNameWithoutCharEspecialCapitalize(d) + "Entity> lista" + ProcessorUtils.getNameWithoutCharEspecialCapitalize(d) + ";");
addLineBlank(sbEntity);
}
);
}
private void configureFieldId(String tableName, String nomeSequenceGenerator, StringBuilder sbEntity) {
addCode(sbEntity, "\t@Id");
addCode(sbEntity, "\t@GeneratedValue(strategy = GenerationType.AUTO, generator = \"" + nomeSequenceGenerator + "\")");
addCode(sbEntity, "\t@Column(name = \"" + ProcessorUtils.nameIdColumn(tableName) + "\")");
addCode(sbEntity, "\tprivate Long id;");
addLineBlank(sbEntity);
}
private void configureClass(String tableName, String codeLookup, String descriptionLookup, String nomeSemCaracterEspecial, String nomeSemCaracterEspecialMinusculo, String nomeSequenceGenerator, StringBuilder sbEntity, List fieldForms) {
if (codeLookup != null && descriptionLookup != null && !codeLookup.isEmpty() && !descriptionLookup.isEmpty()) {
addCode(sbEntity, "@JArchLookup(codeAttribute = \"" + codeLookup + "\", descriptionAttribute = \"" + descriptionLookup + "\")");
}
if (secret) {
addCode(sbEntity, "@JArchConfidential");
}
if (exclusionLogic) {
addCode(sbEntity, "@JArchExclusionLogic");
}
addCode(sbEntity, "@Audited");
addCode(sbEntity, "@Table(name = \"" + tableName + "\", \n" +
"\t\tindexes = {\n" +
"\t\t\t\t" +
fieldForms
.stream()
.filter(f -> !f.getSearch().isEmpty())
.map(f -> "@Index(columnList = \"" + f.getCampoTabela() + "\", name = \"" + "dx_".concat(tableName.substring(3).replace("_", "").concat(f.getCampoTabela().substring(0, 6).replace("_", ""))) + "\")")
.collect(Collectors.joining(",\n\t\t\t\t")) +
"\n\t\t})");
addCode(sbEntity, "@Entity(name = \"" + nomeSemCaracterEspecialMinusculo + "\")");
addCode(sbEntity, "@SequenceGenerator(name = \"" + nomeSequenceGenerator + "\", sequenceName = \"" + ProcessorUtils.nameSequence(tableName) + "\", allocationSize = 1)");
addCode(sbEntity, "public class " + nomeSemCaracterEspecial + "Entity extends CrudMultiTenantEntity {");
addLineBlank(sbEntity);
}
private void configureImport(Set imports, StringBuilder sbEntity) {
imports.forEach(imp -> addCode(sbEntity, imp));
addLineBlank(sbEntity);
}
private void configurePackage(StringBuilder sbEntity) {
addCode(sbEntity, "package " + namePackage + ";");
addLineBlank(sbEntity);
}
private void getSetMaster(String nameMaster, String nameMasterSemCaracterEspecial, String nameMasterSemCaracterEspecialMinusculo, StringBuilder sbEntity) {
if (nameMaster != null && !nameMaster.isEmpty()) {
addCode(sbEntity, "\tpublic " + nameMasterSemCaracterEspecial + "Entity get" + nameMasterSemCaracterEspecial + "() {");
addCode(sbEntity, "\t\treturn " + nameMasterSemCaracterEspecialMinusculo + ";");
addCode(sbEntity, "\t}");
addLineBlank(sbEntity);
addCode(sbEntity, "\tpublic void set" + nameMasterSemCaracterEspecial + "(" + nameMasterSemCaracterEspecial + "Entity " + nameMasterSemCaracterEspecialMinusculo + ") {");
addCode(sbEntity, "\t\tthis." + nameMasterSemCaracterEspecialMinusculo + " = " + nameMasterSemCaracterEspecialMinusculo + ";");
addCode(sbEntity, "\t}");
addLineBlank(sbEntity);
}
}
private void configureRelationMaster(String nameMaster, String nameMasterSemCaracterEspecial, String nameMasterSemCaracterEspecialMinusculo, StringBuilder sbEntity) {
if (nameMaster != null && !nameMaster.isEmpty()) {
addCode(sbEntity, "\t@ManyToOne");
addCode(sbEntity, "\t@JoinColumn(name=\"id_" + nameMasterSemCaracterEspecialMinusculo + "\", nullable = false)");
addCode(sbEntity, "\t@Filter(name = Constant.TENANT)");
addCode(sbEntity, "\tprivate " + nameMasterSemCaracterEspecial + "Entity " + nameMasterSemCaracterEspecialMinusculo + ";");
addLineBlank(sbEntity);
}
}
private void addImports(Set imports, String nameMaster, List details) {
imports.add("import javax.persistence.Column;");
imports.add("import javax.persistence.Entity;");
imports.add("import javax.persistence.GeneratedValue;");
imports.add("import javax.persistence.GenerationType;");
imports.add("import javax.persistence.Id;");
imports.add("import javax.persistence.Index;");
imports.add("import javax.persistence.SequenceGenerator;");
imports.add("import javax.persistence.Table;");
imports.add("import org.hibernate.envers.Audited;");
imports.add("import br.com.jarch.annotation.JArchValidRequired;");
imports.add("import br.com.jarch.crud.entity.CrudMultiTenantEntity;");
if (!details.isEmpty()) {
imports.add("import javax.persistence.OneToMany;");
imports.add("import br.com.jarch.annotation.JArchNoCloneId;");
imports.add("import br.com.jarch.model.Constant;");
imports.add("import org.hibernate.annotations.Filter;");
imports.add("import javax.persistence.CascadeType;");
imports.add("import java.util.Set;");
imports.add("import java.util.HashSet;");
}
if (nameMaster != null && !nameMaster.isEmpty()) {
imports.add("import javax.persistence.JoinColumn;");
imports.add("import javax.persistence.ManyToOne;");
imports.add("import br.com.jarch.model.Constant;");
imports.add("import org.hibernate.annotations.Filter;");
}
if (exclusionLogic) {
imports.add("import br.com.jarch.model.Constant;");
imports.add("import br.com.jarch.annotation.JArchExclusionLogic;");
}
if (secret) {
imports.add("import br.com.jarch.annotation.JArchConfidential;");
}
}
private void loadImportsFieldsGetsSetsEnums(List fieldForms, Set imports, List fields, List columnsDataTable, List getSets, List getSetEnums) {
imports.clear();
fields.clear();
columnsDataTable.clear();
getSets.clear();
getSetEnums.clear();
for (FieldForm campoForm : fieldForms) {
String atributoPrimeiroMaisculo = campoForm.getAtributoPrimeiroLetraMaiusculo();
String definicaoAtributo = "";
if (!campoForm.getTipo().equals(FieldType.ENTITY) && !campoForm.getTipo().isAddress()) {
definicaoAtributo += "\t@Column(name = \"" + campoForm.getCampoTabela() + "\"";
if (campoForm.isObrigatorio()) {
definicaoAtributo += ", nullable = false";
}
if (campoForm.getTipo().getSizeColumnDatabase() > 0) {
definicaoAtributo += ", length = " + campoForm.getTipo().getSizeColumnDatabase();
}
if (campoForm.getTipo().isInteger()) {
definicaoAtributo += ", precision = 6";
} else if (campoForm.getTipo().isLong()) {
definicaoAtributo += ", precision = 8";
} else if (campoForm.getTipo().isDecimal()) {
definicaoAtributo += ", scale = 2, precision = 12";
}
definicaoAtributo += ")\n";
}
String tipo;
if (campoForm.getTipo().isEnum()) {
imports.add("import java.util.Collection;");
tipo = campoForm.getAtributo().substring(0, 1).toUpperCase()
+ campoForm.getAtributo().substring(1)
+ "Type";
} else if (campoForm.getTipo().isAddress()) {
imports.add("import javax.persistence.Embedded;");
tipo = campoForm.getAtributo().substring(0, 1).toUpperCase() +
campoForm.getAtributo().substring(1) +
"Embeddable";
definicaoAtributo += "\t@Embedded\n";
} else if (campoForm.getTipo().equals(FieldType.BINARY)) {
tipo = "byte[]";
definicaoAtributo += "\t@Lob\n";
definicaoAtributo += "\t@Basic(fetch = FetchType.LAZY)\n";
imports.add("import javax.persistence.Lob;");
imports.add("import javax.persistence.Basic;");
imports.add("import javax.persistence.FetchType;");
} else if (campoForm.getTipo().equals(FieldType.MONTH_YEAR)) {
tipo = "YearMonth";
imports.add("import br.com.jarch.jpa.converter.YearMonthJpaConverter;");
imports.add("import javax.persistence.Convert;");
imports.add("import java.time.YearMonth;");
definicaoAtributo += "\t@Convert(converter = YearMonthJpaConverter.class)\n";
} else if (campoForm.getTipo().equals(FieldType.HOUR)) {
tipo = "LocalTime";
imports.add("import br.com.jarch.jpa.converter.LocalTimeJpaConverter;");
imports.add("import javax.persistence.Convert;");
imports.add("import java.time.LocalTime;");
definicaoAtributo += "\t@Convert(converter = LocalTimeJpaConverter.class)\n";
} else if (campoForm.getTipo().equals(FieldType.DATE)) {
tipo = "LocalDate";
imports.add("import br.com.jarch.jpa.converter.LocalDateJpaConverter;");
imports.add("import javax.persistence.Convert;");
imports.add("import java.time.LocalDate;");
definicaoAtributo += "\t@Convert(converter = LocalDateJpaConverter.class)\n";
} else if (campoForm.getTipo().equals(FieldType.DATE_TIME)) {
tipo = "LocalDateTime";
imports.add("import br.com.jarch.jpa.converter.LocalDateTimeJpaConverter;");
imports.add("import javax.persistence.Convert;");
imports.add("import java.time.LocalDateTime;");
definicaoAtributo += "\t@Convert(converter = LocalDateTimeJpaConverter.class)\n";
} else if (campoForm.getTipo().equals(FieldType.MONEY)
|| campoForm.getTipo().equals(FieldType.RATE)
|| campoForm.getTipo().equals(FieldType.PERCENTAGE)) {
tipo = "BigDecimal";
imports.add("import java.math.BigDecimal;");
} else if (campoForm.getTipo().equals(FieldType.QUANTITY)) {
tipo = "Integer";
} else if (campoForm.getTipo().equals(FieldType.SEQUENCE)
|| campoForm.getTipo().equals(FieldType.NUMBER)) {
tipo = "Long";
} else if (campoForm.getTipo().equals(FieldType.CNPJ)) {
imports.add("import br.com.jarch.annotation.JArchValidCnpj;");
tipo = "String";
definicaoAtributo += "\t@JArchValidCnpj\n";
} else if (campoForm.getTipo().equals(FieldType.CNPJ_BASE)) {
imports.add("import br.com.jarch.annotation.JArchValidCnpjBase;");
tipo = "String";
definicaoAtributo += "\t@JArchValidCnpjBase\n";
} else if (campoForm.getTipo().equals(FieldType.CPF)) {
imports.add("import br.com.jarch.annotation.JArchValidCpf;");
tipo = "String";
definicaoAtributo += "\t@JArchValidCpf\n";
} else if (campoForm.getTipo().equals(FieldType.CEP)) {
imports.add("import br.com.jarch.annotation.JArchValidCep;");
tipo = "String";
definicaoAtributo += "\t@JArchValidCep\n";
} else if (campoForm.getTipo().equals(FieldType.CPFCNPJ)) {
imports.add("import br.com.jarch.annotation.JArchValidCpfCnpj;");
tipo = "String";
definicaoAtributo += "\t@JArchValidCpfCnpj\n";
} else if (campoForm.getTipo().equals(FieldType.TELEPHONE) || campoForm.getTipo().equals(FieldType.CELLPHONE)) {
imports.add("import br.com.jarch.annotation.JArchValidPhone;");
tipo = "String";
definicaoAtributo += "\t@JArchValidPhone\n";
} else if (campoForm.getTipo().equals(FieldType.BOOLEAN)) {
imports.add("import br.com.jarch.jpa.converter.br.BooleanSNJpaConverter;");
imports.add("import javax.persistence.Convert;");
tipo = "Boolean";
definicaoAtributo += "\t@Convert(converter = BooleanSNJpaConverter.class)\n";
} else if (campoForm.getTipo().equals(FieldType.EMAIL)) {
imports.add("import br.com.jarch.annotation.JArchValidEmail;");
tipo = "String";
definicaoAtributo += "\t@JArchValidEmail\n";
} else if (campoForm.getTipo().equals(FieldType.PASSWORD)) {
tipo = "String";
} else if (campoForm.getTipo().equals(FieldType.ENTITY)) {
imports.add("import javax.persistence.OneToOne;");
tipo = campoForm.getAtributo().substring(0, 1).toUpperCase() + campoForm.getAtributo().substring(1) + "Entity";
} else {
tipo = "String";
}
if (campoForm.isObrigatorio()) {
imports.add("import br.com.jarch.annotation.JArchValidRequired;");
definicaoAtributo += "\t@JArchValidRequired(\"" + campoForm.getBundleCodigoJava() + "\")\n";
}
if (campoForm.getTipo().getSizeColumnDatabase() > 0
&& tipo.equals("String")
&& !campoForm.getTipo().isCpfCnpj()) {
imports.add("import javax.validation.constraints.Size;");
definicaoAtributo += "\t@Size(max = " + campoForm.getTipo().getSizeColumnDatabase() + ", message = \"{message.maxSizeExceeded}\")\n";
}
if (campoForm.getTipo().equals(FieldType.ENTITY)) {
imports.add("import javax.persistence.JoinColumn;");
imports.add("import br.com.jarch.model.Constant;");
imports.add("import org.hibernate.annotations.Filter;");
if (ProcessorUtils.isExistsEntity(element, campoForm.getDescricao(), campoForm.getAtributo().toLowerCase())) {
String packageEntityField = ProcessorUtils.getPackage(element, campoForm.getAtributo().toLowerCase());
String nameEntityField = ProcessorUtils.getNomeEntity(campoForm.getDescricao());
imports.add("import " + packageEntityField.concat(".").concat(nameEntityField) + ";");
}
// if (ProcessorUtils.isExistsEntity(element, ProcessorUtils.getNomeEntity(campoForm.getAtributo()), campoForm.getAtributo())) {
//
// }
definicaoAtributo += "\t@OneToOne\n";
definicaoAtributo += "\t@JoinColumn(name = \"" + campoForm.getCampoTabela() + "\"" + (campoForm.isObrigatorio() ? ", nullable = false" : "") + ")\n";
definicaoAtributo += "\t@Filter(name = Constant.TENANT)\n";
definicaoAtributo += exclusionLogic ? "\t@JArchExclusionLogic\n" : "";
}
if (campoForm.isExclusive()) {
imports.add("import br.com.jarch.annotation.JArchValidExclusive;");
definicaoAtributo += "\t@JArchValidExclusive\n";
}
definicaoAtributo += "\tprivate " + tipo + " " + campoForm.getAtributo() + ";";
fields.add(definicaoAtributo);
if (!campoForm.getXhtml().isEmpty()) {
columnsDataTable.add(tipo + " " + campoForm.getAtributo());
}
String getIs = /*campoForm.getTipo().equals(FieldType.BOOLEAN) ? "is" : */"get";
String getSet = "\tpublic " + tipo + " " + getIs + atributoPrimeiroMaisculo + "() {\n"
+ "\t\treturn " + campoForm.getAtributo() + ";\n"
+ "\t}\n"
+ "\n"
+ "\tpublic void set" + atributoPrimeiroMaisculo + "(" + tipo + " " + campoForm.getAtributo() + ") {\n"
+ "\t\tthis." + campoForm.getAtributo() + " = " + campoForm.getAtributo() + ";\n"
+ "\t}";
getSets.add(getSet);
addGetSetEnum(getSetEnums, campoForm);
}
}
private void addGetSetEnum(List getSetEnums, FieldForm campoForm) {
if (!campoForm.getTipo().isEnum()) {
return;
}
String nomeEnum = campoForm.getAtributo().substring(0, 1).toUpperCase() +
campoForm.getAtributo().substring(1) +
"Type";
getSetEnums.add(
"\tpublic Collection<" + nomeEnum + "> get" + campoForm.getAtributoPrimeiroLetraMaiusculo() + "s() {\n"
+ "\t return " + nomeEnum + ".getCollection();\n"
+ "\t}\n"
+ "\n"
+ "\tpublic String get" + campoForm.getAtributoPrimeiroLetraMaiusculo() + "Description() {\n"
+ "\t return " + campoForm.getAtributo() + " == null ? \"\" : " + campoForm.getAtributo() + ".getDescription();\n"
+ "\t}");
}
private void saveEnums(List fieldForms) {
fieldForms
.stream()
.filter(f -> f.getTipo().isEnum())
.filter(f -> !FieldType.ENUMERATED.equals(f.getTipo()))
.forEach(fieldForm -> {
TypeCodeGenerate.generate(element, fieldForm.getAtributo(), nameSubPackage);
JpaConverterCodeGenerate.generate(element, fieldForm.getAtributo(), nameSubPackage);
});
}
private void saveEnderecos(List fieldForms) {
fieldForms
.stream()
.filter(f -> f.getTipo().isAddress())
.forEach(fieldForm -> EmbeddableAddressCodeGenerate.generate(element, fieldForm.getAtributo(), nameSubPackage, fieldForm.isObrigatorio()));
}
private void configEnviroment() {
namePackage = ProcessorUtils.getPackage(element, nameSubPackage);
File folder = new File(ProcessorUtils.getPathSource(element, nameSubPackage));
if (!folder.exists()) {
folder.mkdir();
}
}
private void generateScriptDataBase(String name, String tableName, List fieldForms, String tableNameOwner) {
folderScript = new File(ProcessorUtils.getPathSource(element, nameSubPackage) + File.separator + "sql");
if (!folderScript.exists())
folderScript.mkdir();
scriptOracle(name, tableName, fieldForms, tableNameOwner);
scriptPostgreSql(name, tableName, fieldForms, tableNameOwner);
scriptMySql(name, tableName, fieldForms, tableNameOwner);
scriptSqlServer(name, tableName, fieldForms, tableNameOwner);
scriptDb2(name, tableName, fieldForms, tableNameOwner);
scriptSybase(name, tableName, fieldForms, tableNameOwner);
}
private void scriptOracle(String name, String tableName, List fieldForms, String tableNameOwner) {
String nomeSemCaracterEspecial = ProcessorUtils.getNameWithoutCharEspecialCapitalize(name);
File fileScript = new File(folderScript.getAbsolutePath() + File.separator + nomeSemCaracterEspecial + "Oracle.sql");
StringBuilder sbScript = new StringBuilder();
addTableOracleSybase(sbScript, tableName, fieldForms, tableNameOwner);
addForeignKeysOracle(sbScript, tableName, fieldForms, tableNameOwner);
addIndexes(sbScript, tableName, fieldForms);
addSequenceDb2OracleSybase(sbScript, tableName);
addTableAudityOraclePostgreSql(sbScript, tableName, fieldForms, tableNameOwner);
saveFile(fileScript, sbScript.toString());
}
private void scriptPostgreSql(String name, String tableName, List fieldForms, String tableNameOwner) {
StringBuilder sbScript = new StringBuilder();
addTablePostgreSql(sbScript, tableName, fieldForms, tableNameOwner);
addForeignKeyPostgreSqlMySqlSqlServer(sbScript, tableName, fieldForms, tableNameOwner);
addIndexes(sbScript, tableName, fieldForms);
addSequencePostgreSql(sbScript, tableName);
addTableAudityOraclePostgreSql(sbScript, tableName, fieldForms, tableNameOwner);
String nomeSemCaracterEspecial = ProcessorUtils.getNameWithoutCharEspecialCapitalize(name);
File fileScript = new File(folderScript.getAbsolutePath() + File.separator + nomeSemCaracterEspecial + "PostgreSQL.sql");
saveFile(fileScript, sbScript.toString());
}
private void addTableAudityOraclePostgreSql(StringBuilder sbScript, String tableName, List fieldForms, String tableNameOwner) {
addComentTableAuditScript(sbScript, tableName);
addCode(sbScript, "CREATE TABLE ." + tableName.toUpperCase() + "_AUD (");
addCode(sbScript, "\tID_AUDITORIA NUMBER NOT NULL,");
addCode(sbScript, "\tTP_AUDITORIA NUMBER,");
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableName).toUpperCase() + " NUMBER,");
if (isHasTableOwner(tableNameOwner))
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableNameOwner).toUpperCase() + " NUMBER,");
addColumnDateExclusion(sbScript);
addCode(sbScript, "\tSQ_VERSAOREGISTRO NUMBER,");
addCode(sbScript, "\tID_MULTITENANT NUMBER,");
addCode(sbScript, getScriptColumnsNullable(fieldForms));
addCode(sbScript, "\tDH_ALTERACAO TIMESTAMP,");
addCode(sbScript, "\tID_USUARIOALTERACAO NUMBER");
addCode(sbScript, ");");
}
private void addColumnDateExclusion(StringBuilder sbScript) {
addCode(sbScript, "\tDH_EXCLUSAO TIMESTAMP,");
}
private void addSequencePostgreSql(StringBuilder sbScript, String tableName) {
addCode(sbScript, "-- CREATE SEQUENCE FROM ." + tableName.toUpperCase() + " TO ID");
addCode(sbScript, "CREATE SEQUENCE ." + ProcessorUtils.nameSequence(tableName).toUpperCase() + " START WITH 1 MINVALUE 1 MAXVALUE 999999999999;");
addLineBlank(sbScript);
}
private void addForeignKeyPostgreSqlMySqlSqlServer(StringBuilder sbScript, String tableName, List fieldForms, String tableNameOwner) {
List fieldFormEntitys = fieldForms.stream().filter(f -> f.getTipo().isEntity()).collect(Collectors.toList());
if (!fieldFormEntitys.isEmpty() || isHasTableOwner(tableNameOwner)) {
addCode(sbScript, "-- CREATE FOREIGN KEY'S FROM ." + tableName.toUpperCase());
if (isHasTableOwner(tableNameOwner)) {
String nameColumn = ProcessorUtils.nameIdColumn(tableNameOwner);
String nameForeignKey = "FK_".concat(tableName.substring(3).concat(nameColumn.substring(0, 6)).replace("_", "")).toUpperCase();
String nameTableReference = nameColumn.replace("ID_", "TB_");
addCode(sbScript, "ALTER TABLE ." + tableName.toUpperCase() + " ADD CONSTRAINT " + nameForeignKey + " FOREIGN KEY (" + nameColumn + ") REFERENCES ." + nameTableReference + "(" + nameColumn + ")");
}
String foreignKeys = fieldFormEntitys
.stream()
.map(f -> {
String nameColumn = f.getCampoTabela().toUpperCase();
String nameForeignKey = "FK_".concat(tableName.substring(3).concat(nameColumn.substring(0, 6)).replace("_", "")).toUpperCase();
String nameTableReference = nameColumn.replace("ID_", "TB_");
return "ALTER TABLE ." + tableName.toUpperCase() + " ADD CONSTRAINT " + nameForeignKey + " FOREIGN KEY (" + nameColumn + ") REFERENCES ." + nameTableReference + "(" + nameColumn + ")";
})
.collect(Collectors.joining(";\n"));
addCode(sbScript, foreignKeys);
addLineBlank(sbScript);
}
}
private void addTablePostgreSql(StringBuilder sbScript, String tableName, List fieldForms, String tableNameOwner) {
addCode(sbScript, "-- >>>>>>>>>>> SCRIPT DATABASE <<<<<<<<<<<<<");
addCode(sbScript, "-- CREATE TABLE ." + tableName.toUpperCase());
addCode(sbScript, "CREATE TABLE ." + tableName.toUpperCase() + " (");
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableName).toUpperCase() + " NUMBER NOT NULL,");
if (isHasTableOwner(tableNameOwner))
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableNameOwner).toUpperCase() + " NUMBER NOT NULL,");
addColumnDateExclusion(sbScript);
addCode(sbScript, "\tSQ_VERSAOREGISTRO NUMBER NOT NULL,");
addCode(sbScript, "\tID_MULTITENANT NUMBER NOT NULL,");
addCode(sbScript, getScriptColumnFromFieldForms(fieldForms).concat(","));
addCode(sbScript, "\tDH_ALTERACAO TIMESTAMP,");
addCode(sbScript, "\tID_USUARIOALTERACAO NUMBER");
String namePrimaryKey = "PK_".concat(ProcessorUtils.nameIdColumn(tableName).toUpperCase().substring(3));
addCode(sbScript, "\tCONSTRAINT " + namePrimaryKey + " PRIMARY KEY (" + ProcessorUtils.nameIdColumn(tableName).toUpperCase() + "));");
addLineBlank(sbScript);
}
private void scriptMySql(String name, String tableName, List fieldForms, String tableNameOwner) {
String nomeSemCaracterEspecial = ProcessorUtils.getNameWithoutCharEspecialCapitalize(name);
File fileScript = new File(folderScript.getAbsolutePath() + File.separator + nomeSemCaracterEspecial + "MySql.sql");
StringBuilder sbScript = new StringBuilder();
addTableMySql(sbScript, tableName, fieldForms, tableNameOwner);
addForeignKeyPostgreSqlMySqlSqlServer(sbScript, tableName, fieldForms, tableNameOwner);
addIndexes(sbScript, tableName, fieldForms);
addTableAuditMySql(sbScript, tableName, fieldForms, tableNameOwner);
saveFile(fileScript, sbScript.toString().replace("NUMBER", "BIGINT").replace("VARCHAR2", "VARCHAR"));
}
private void addTableAuditMySql(StringBuilder sbScript, String tableName, List fieldForms, String tableNameOwner) {
addComentTableAuditScript(sbScript, tableName);
addCode(sbScript, "CREATE TABLE ." + tableName.toUpperCase() + "_AUD (");
addCode(sbScript, "\tID_AUDITORIA NUMBER NOT NULL,");
addCode(sbScript, "\tTP_AUDITORIA NUMBER,");
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableName).toUpperCase() + " NUMBER,");
if (isHasTableOwner(tableNameOwner))
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableNameOwner).toUpperCase() + " NUMBER,");
addColumnDateExclusion(sbScript);
addCode(sbScript, "\tSQ_VERSAOREGISTRO NUMBER,");
addCode(sbScript, "\tID_MULTITENANT NUMBER,");
addCode(sbScript, getScriptColumnsNullable(fieldForms));
addCode(sbScript, "\tDH_ALTERACAO TIMESTAMP,");
addCode(sbScript, "\tID_USUARIOALTERACAO NUMBER");
String namePrimaryKey = "PK_".concat(ProcessorUtils.nameIdColumn(tableName).toUpperCase().substring(3));
addCode(sbScript, "\tCONSTRAINT " + namePrimaryKey + "AUD PRIMARY KEY (ID_AUDITORIA));");
}
private void addComentTableAuditScript(StringBuilder sbScript, String tableName) {
addCode(sbScript, "-- CREATE TABLE AUDIT FROM ." + tableName.toUpperCase());
}
private void addTableMySql(StringBuilder sbScript, String tableName, List fieldForms, String tableNameOwner) {
addCode(sbScript, "-- >>>>>>>>>>> SCRIPT DATABASE <<<<<<<<<<<<<");
addCode(sbScript, "-- CREATE TABLE ." + tableName.toUpperCase());
addCode(sbScript, "CREATE TABLE ." + tableName.toUpperCase() + " (");
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableName).toUpperCase() + " NUMBER AUTO_INCREMENT NOT NULL,");
if (isHasTableOwner(tableNameOwner))
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableNameOwner).toUpperCase() + " NUMBER NOT NULL,");
addColumnDateExclusion(sbScript);
addCode(sbScript, "\tSQ_VERSAOREGISTRO NUMBER NOT NULL,");
addCode(sbScript, "\tID_MULTITENANT NUMBER NOT NULL,");
addCode(sbScript, getScriptColumnFromFieldForms(fieldForms).concat(","));
addCode(sbScript, "\tDH_ALTERACAO TIMESTAMP,");
addCode(sbScript, "\tID_USUARIOALTERACAO NUMBER");
String namePrimaryKey = "PK_".concat(ProcessorUtils.nameIdColumn(tableName).toUpperCase().substring(3));
addCode(sbScript, "\tCONSTRAINT " + namePrimaryKey + " PRIMARY KEY (" + ProcessorUtils.nameIdColumn(tableName).toUpperCase() + "));");
addLineBlank(sbScript);
}
private void scriptSqlServer(String name, String tableName, List fieldForms, String tableNameOwner) {
String nomeSemCaracterEspecial = ProcessorUtils.getNameWithoutCharEspecialCapitalize(name);
File fileScript = new File(folderScript.getAbsolutePath() + File.separator + nomeSemCaracterEspecial + "SqlServer.sql");
StringBuilder sbScript = new StringBuilder();
addTableSqlServer(sbScript, tableName, fieldForms, tableNameOwner);
addForeignKeyPostgreSqlMySqlSqlServer(sbScript, tableName, fieldForms, tableNameOwner);
addIndexes(sbScript, tableName, fieldForms);
addTableAuditSqlServer(sbScript, tableName, fieldForms, tableNameOwner);
saveFile(fileScript, sbScript.toString().replace("NUMBER", "BIGINT").replace("VARCHAR2", "VARCHAR"));
}
private void addTableAuditSqlServer(StringBuilder sbScript, String tableName, List fieldForms, String tableNameOwner) {
addComentTableAuditScript(sbScript, tableName);
addCode(sbScript, "CREATE TABLE ." + tableName.toUpperCase() + "_AUD (");
addCode(sbScript, "\tID_AUDITORIA NUMBER NOT NULL,");
addCode(sbScript, "\tTP_AUDITORIA NUMBER,");
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableName).toUpperCase() + " NUMBER,");
if (isHasTableOwner(tableNameOwner))
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableNameOwner).toUpperCase() + " NUMBER,");
addColumnDateExclusion(sbScript);
addCode(sbScript, "\tSQ_VERSAOREGISTRO NUMBER,");
addCode(sbScript, "\tID_MULTITENANT NUMBER,");
addCode(sbScript, getScriptColumnsNullable(fieldForms));
addCode(sbScript, "\tDH_ALTERACAO TIMESTAMP,");
addCode(sbScript, "\tID_USUARIOALTERACAO NUMBER");
String namePrimaryKey = "PK_".concat(ProcessorUtils.nameIdColumn(tableName).toUpperCase().substring(3));
addCode(sbScript, "\tCONSTRAINT " + namePrimaryKey + "AUD PRIMARY KEY (ID_AUDITORIA));");
}
private void addTableSqlServer(StringBuilder sbScript, String tableName, List fieldForms, String tableNameOwner) {
addCode(sbScript, "-- >>>>>>>>>>> SCRIPT DATABASE <<<<<<<<<<<<<");
addCode(sbScript, "-- CREATE TABLE ." + tableName.toUpperCase());
addCode(sbScript, "CREATE TABLE ." + tableName.toUpperCase() + " (");
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableName).toUpperCase() + " NUMBER IDENTITY(1,1) NOT NULL,");
if (isHasTableOwner(tableNameOwner))
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableNameOwner).toUpperCase() + " NUMBER NOT NULL,");
addColumnDateExclusion(sbScript);
addCode(sbScript, "\tSQ_VERSAOREGISTRO NUMBER NOT NULL,");
addCode(sbScript, "\tID_MULTITENANT NUMBER NOT NULL,");
addCode(sbScript, getScriptColumnFromFieldForms(fieldForms).concat(","));
addCode(sbScript, "\tDH_ALTERACAO TIMESTAMP,");
addCode(sbScript, "\tID_USUARIOALTERACAO NUMBER");
String namePrimaryKey = "PK_".concat(ProcessorUtils.nameIdColumn(tableName).toUpperCase().substring(3));
addCode(sbScript, "\tCONSTRAINT " + namePrimaryKey + " PRIMARY KEY (" + ProcessorUtils.nameIdColumn(tableName).toUpperCase() + "));");
addLineBlank(sbScript);
}
private void scriptDb2(String name, String tableName, List fieldForms, String tableNameOwner) {
String nomeSemCaracterEspecial = ProcessorUtils.getNameWithoutCharEspecialCapitalize(name);
File fileScript = new File(folderScript.getAbsolutePath() + File.separator + nomeSemCaracterEspecial + "Db2.sql");
StringBuilder sbScript = new StringBuilder();
addTableDb2(sbScript, tableName, fieldForms, tableNameOwner);
addForeignKeys(sbScript, tableName, fieldForms, tableNameOwner);
addIndexes(sbScript, tableName, fieldForms);
addSequenceDb2OracleSybase(sbScript, tableName);
addTableAuditSybase(sbScript, tableName, fieldForms, tableNameOwner);
saveFile(fileScript, sbScript.toString());
}
private void addTableDb2(StringBuilder sbScript, String tableName, List fieldForms, String tableNameOwner) {
addCode(sbScript, "-- >>>>>>>>>>> SCRIPT DATABASE <<<<<<<<<<<<<");
addCode(sbScript, "-- CREATE TABLE ." + tableName.toUpperCase());
addCode(sbScript, "CREATE TABLE ." + tableName.toUpperCase() + " (");
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableName).toUpperCase() + " NUMBER NOT NULL CONSTRAINT " + ProcessorUtils.namePrimaryKey(tableName).toUpperCase() + " PRIMARY KEY,");
if (isHasTableOwner(tableNameOwner))
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableNameOwner).toUpperCase() + " NUMBER NOT NULL,");
addColumnDateExclusion(sbScript);
addCode(sbScript, "\tSQ_VERSAOREGISTRO NUMBER NOT NULL,");
addCode(sbScript, "\tID_MULTITENANT NUMBER NOT NULL,");
addCode(sbScript, getScriptColumnFromFieldForms(fieldForms));
addCode(sbScript, "\tDH_ALTERACAO TIMESTAMP,");
addCode(sbScript, "\tID_USUARIOALTERACAO NUMBER");
addCode(sbScript, ");");
addLineBlank(sbScript);
}
private void scriptSybase(String name, String tableName, List fieldForms, String tableNameOwner) {
String nomeSemCaracterEspecial = ProcessorUtils.getNameWithoutCharEspecialCapitalize(name);
File fileScript = new File(folderScript.getAbsolutePath() + File.separator + nomeSemCaracterEspecial + "Sybase.sql");
StringBuilder sbScript = new StringBuilder();
addTableOracleSybase(sbScript, tableName, fieldForms, tableNameOwner);
addForeignKeys(sbScript, tableName, fieldForms, tableNameOwner);
addIndexes(sbScript, tableName, fieldForms);
addSequenceDb2OracleSybase(sbScript, tableName);
addTableAuditSybase(sbScript, tableName, fieldForms, tableNameOwner);
saveFile(fileScript, sbScript.toString());
}
private void addTableAuditSybase(StringBuilder sbScript, String tableName, List fieldForms, String tableNameOwner) {
addComentTableAuditScript(sbScript, tableName);
addCode(sbScript, "CREATE TABLE ." + tableName.toUpperCase() + "_AUD (");
addCode(sbScript, "\tID_AUDITORIA NUMBER NOT NULL CONSTRAINT " + ProcessorUtils.namePrimaryKey(tableName).toUpperCase() + " PRIMARY KEY,");
addCode(sbScript, "\tTP_AUDITORIA NUMBER,");
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableName).toUpperCase() + " NUMBER,");
if (isHasTableOwner(tableNameOwner))
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableNameOwner).toUpperCase() + " NUMBER,");
addColumnDateExclusion(sbScript);
addCode(sbScript, "\tSQ_VERSAOREGISTRO NUMBER,");
addCode(sbScript, "\tID_MULTITENANT NUMBER,");
addCode(sbScript, getScriptColumnsNullable(fieldForms));
addCode(sbScript, "\tDH_ALTERACAO TIMESTAMP,");
addCode(sbScript, "\tID_USUARIOALTERACAO NUMBER");
addCode(sbScript, ");");
}
private void addForeignKeys(StringBuilder sbScript, String tableName, List fieldForms, String tableNameOwner) {
List fieldFormEntitys = fieldForms.stream().filter(f -> f.getTipo().isEntity()).collect(Collectors.toList());
if (!fieldFormEntitys.isEmpty() || isHasTableOwner(tableNameOwner)) {
addCode(sbScript, "-- CREATE FOREIGN KEYS FROM ." + tableName.toUpperCase());
if (isHasTableOwner(tableNameOwner)) {
String nameColumn = ProcessorUtils.nameIdColumn(tableNameOwner);
String nameForeignKey = "FK_".concat(tableName.substring(3).concat(nameColumn.substring(0, 6)).replace("_", "")).toUpperCase();
String nameTableReference = nameColumn.replace("ID_", "TB_");
addCode(sbScript, "ALTER TABLE ." + tableName.toUpperCase() + " ADD CONSTRAINT " + nameForeignKey + " FOREIGN KEY (" + nameColumn + ") REFERENCES ." + nameTableReference + "(" + nameColumn + ")");
}
for (FieldForm fieldForm : fieldFormEntitys) {
String nameColumn = fieldForm.getCampoTabela().toUpperCase();
String nameForeignKey = "FK_".concat(tableName.substring(3).concat(nameColumn.substring(0, 6)).replace("_", "")).toUpperCase();
String nameTableReference = nameColumn.replace("ID_", "TB_");
addCode(sbScript, "ALTER TABLE ." + tableName.toUpperCase() + " ADD CONSTRAINT " + nameForeignKey + " FOREIGN KEY (" + nameColumn + ") REFERENCES ." + nameTableReference + "(" + nameColumn + ");");
}
addLineBlank(sbScript);
}
}
private void addTableOracleSybase(StringBuilder sbScript, String tableName, List fieldForms, String tableNameOwner) {
addCode(sbScript, "-- >>>>>>>>>>> SCRIPT DATABASE <<<<<<<<<<<<<");
addCode(sbScript, "-- CREATE TABLE ." + tableName.toUpperCase());
addCode(sbScript, "CREATE TABLE ." + tableName.toUpperCase() + " (");
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableName).toUpperCase() + " NUMBER(19) NOT NULL CONSTRAINT " + ProcessorUtils.namePrimaryKey(tableName).toUpperCase() + " PRIMARY KEY,");
if (isHasTableOwner(tableNameOwner))
addCode(sbScript, "\t" + ProcessorUtils.nameIdColumn(tableNameOwner).toUpperCase() + " NUMBER(19) NOT NULL,");
addColumnDateExclusion(sbScript);
addCode(sbScript, "\tSQ_VERSAOREGISTRO NUMBER(6) NOT NULL,");
addCode(sbScript, "\tID_MULTITENANT NUMBER(5) NOT NULL,");
addCode(sbScript, getScriptColumnFromFieldForms(fieldForms));
addCode(sbScript, "\tDH_ALTERACAO TIMESTAMP,");
addCode(sbScript, "\tID_USUARIOALTERACAO NUMBER");
addCode(sbScript, ");");
addLineBlank(sbScript);
}
private boolean isHasTableOwner(String tableNameOwner) {
return tableNameOwner != null && !tableNameOwner.isBlank();
}
private void addSequenceDb2OracleSybase(StringBuilder sbScript, String tableName) {
addCode(sbScript, "-- CREATE SEQUENCE FROM ." + tableName.toUpperCase() + " TO ID");
addCode(sbScript, "CREATE SEQUENCE ." + ProcessorUtils.nameSequence(tableName).toUpperCase() + " NOCACHE START WITH 1 MINVALUE 1 MAXVALUE 999999999999;");
addLineBlank(sbScript);
}
private void addIndexes(StringBuilder sbScript, String tableName, List fieldForms) {
List fieldFormIndexes = fieldForms.stream().filter(f -> !f.getSearch().isEmpty()).collect(Collectors.toList());
if (!fieldFormIndexes.isEmpty()) {
addCode(sbScript, "-- CREATE INDEXES FROM ." + tableName.toUpperCase());
for (FieldForm fieldForm : fieldFormIndexes) {
String nameIndex = "DX_".concat(tableName.substring(3).concat(fieldForm.getCampoTabela().substring(0, 6)).replace("_", "")).toUpperCase();
addCode(sbScript, "CREATE INDEX " + nameIndex + " ON ." + tableName.toUpperCase() + "(" + fieldForm.getCampoTabela().toUpperCase() + ");");
}
addLineBlank(sbScript);
}
}
private void addForeignKeysOracle(StringBuilder sbScript, String tableName, List fieldForms, String tableNameOwner) {
boolean hasTableOnwer = isHasTableOwner(tableNameOwner);
List fieldFormEntitys = fieldForms.stream().filter(f -> f.getTipo().isEntity()).collect(Collectors.toList());
if (!fieldFormEntitys.isEmpty() || hasTableOnwer) {
addCode(sbScript, "-- CREATE FOREIGN KEYS FROM ." + tableName.toUpperCase());
if (hasTableOnwer) {
String nameColumn = ProcessorUtils.nameIdColumn(tableNameOwner).toUpperCase();
String nameForeignKey = "FK_".concat(tableName.substring(3).concat(nameColumn.substring(0, 6)).replace("_", "")).toUpperCase();
String nameTableReference = nameColumn.replace("ID_", "TB_");
addCode(sbScript, "ALTER TABLE ." + tableName.toUpperCase() + " ADD CONSTRAINT " + nameForeignKey + " FOREIGN KEY (" + nameColumn + ") REFERENCES ." + nameTableReference + "(" + nameColumn + ");");
}
for (FieldForm fieldForm : fieldFormEntitys) {
String nameColumn = fieldForm.getCampoTabela().toUpperCase();
String nameForeignKey = "FK_".concat(tableName.substring(3).concat(nameColumn.substring(0, 6)).replace("_", "")).toUpperCase();
String nameTableReference = nameColumn.replace("ID_", "TB_");
addCode(sbScript, "ALTER TABLE ." + tableName.toUpperCase() + " ADD CONSTRAINT " + nameForeignKey + " FOREIGN KEY (" + nameColumn + ") REFERENCES ." + nameTableReference + "(" + nameColumn + ");");
}
addLineBlank(sbScript);
}
}
private String getScriptColumnFromFieldForms(List fieldForms) {
return fieldForms
.stream()
.map(f -> "\t" + f.getTipo().getColumnSqlType(f.getCampoTabela(), f.isObrigatorio()))
.collect(Collectors.joining(",\n"))
.concat(",");
}
private String getScriptColumnsNullable(List fieldForms) {
return fieldForms
.stream()
.map(f -> "\t" + f.getTipo().getColumnSqlType(f.getCampoTabela(), false))
.collect(Collectors.joining(",\n"))
.concat(",");
}
private void saveFile(File file, String content) {
try {
if (ProcessorUtils.isFileExistsClientOrWebOrWs(file))
return;
FileUtils.save(file, content);
ProcessorUtils.messageNote("JARCH Created ==> " + file.getAbsoluteFile(), element);
} catch (IOException e) {
LogUtils.generate(e);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy