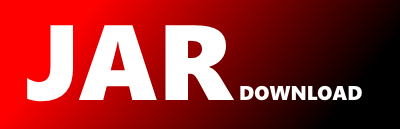
br.com.jarch.bpm.util.BpmUtils Maven / Gradle / Ivy
package br.com.jarch.bpm.util;
import br.com.jarch.exception.ValidationException;
import br.com.jarch.model.MultiTenant;
import br.com.jarch.model.ProcessInstanceBean;
import br.com.jarch.model.TaskBean;
import br.com.jarch.model.UserInformation;
import br.com.jarch.util.DateUtils;
import br.com.jarch.util.JsfUtils;
import br.com.jarch.util.LogUtils;
import org.camunda.bpm.engine.RuntimeService;
import org.camunda.bpm.engine.TaskService;
import javax.enterprise.inject.spi.CDI;
import javax.json.Json;
import javax.json.JsonArray;
import javax.json.JsonObject;
import javax.json.JsonObjectBuilder;
import javax.json.JsonReader;
import javax.json.bind.JsonbBuilder;
import javax.ws.rs.client.ClientBuilder;
import javax.ws.rs.client.WebTarget;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import java.io.StringReader;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.ZoneId;
import java.time.format.DateTimeFormatter;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class BpmUtils {
private static final String ASSIGNEE = "assignee";
private static final String URL_REST_BPM = System.getProperty("CAMUNDA.URL.SERVIDOR", "http://localhost:8080/engine-rest");
private static final String PROCESS_INSTANCE = "process-instance";
private static final String TASK = "task";
private static final String VALUE = "value";
private static final String VARIABLES = "variables";
private static final String CLAIM = "claim";
private static final String UNCLAIM = "unclaim";
private static final String IDENTITY_LINKS = "identity-links";
private static final String DELETE = "delete";
private static final String USER_ID = "userId";
private static final String TYPE = "type";
private static final String CANDIDATE = "candidate";
private static final String PROCESS_INSTANCE_BUSINESS_KEY = "processInstanceBusinessKey";
private static final String PROCESS_DEFINITION_KEY = "processDefinitionKey";
private static final String BUSINESS_KEY = "businessKey";
private static final String PROCESS_DEFINITION = "process-definition";
private static final String ACTIVE = "active";
private static final String LATEST_VERSION = "latestVersion";
private static final String LOCAL_VARIABLES = "localVariables";
private static final String EXECUTION = "execution";
private static final String CANDIDATE_GROUP = "candidateGroup";
private static final String PROCESS_VARIABLES = "processVariables";
private static final String CANDIDATE_GROUPS = "candidateGroups";
private static final String CANDIDATE_USER = "candidateUser";
private static final String INVOLVED_USER = "involvedUser";
private static final String PROCESS_DEFINITION_KEY_IN = "processDefinitionKeyIn";
private static final String SORT_BY = "sortBy";
private static final String SORT_ORDER = "sortOrder";
private static final String PRIORITY = "priority";
private static final String CREATED = "created";
private static final String ASC = "asc";
private static final String MODIFICATIONS = "modifications";
private static final String OBJECT_TYPE_NAME = "objectTypeName";
private static final String SERIALIZATION_DATA_FORMAT = "serializationDataFormat";
private static final String VALUE_INFO = "valueInfo";
// private static final String KEY = "key";
// private static final String START = "start";
private BpmUtils() {
}
public static void addCandidateUser(String taskId, String userId) {
// LogUtils.generate("Chamada REST Camunda Parametros: taskId = " + taskId + " userId = " + userId);
//
// Response response = getWebTarget()
// .path(TASK)
// .path(taskId)
// .path(IDENTITY_LINKS)
// .request(MediaType.APPLICATION_JSON_TYPE)
// .post(Entity.json(Json.createObjectBuilder().add(USER_ID, userId).add(TYPE, CANDIDATE).build()));
//
// validResponse(response);
CDI.current().select(TaskService.class).get().addCandidateUser(taskId, userId);
}
public static void assignee(String taskId, String assignee) {
// LogUtils.generate("Chamada REST Camunda Parametros: taskId = " + taskId + " assignee = " + assignee);
//
// Response response = getWebTarget()
// .path(TASK)
// .path(taskId)
// .path(ASSIGNEE)
// .request()
// .post(Entity.json(Json.createObjectBuilder().add(USER_ID, assignee).build()));
//
// validResponse(response);
CDI.current().select(TaskService.class).get().setAssignee(taskId, assignee);
}
public static void claim(String taskId) {
String user = CDI.current().select(UserInformation.class).get().get().getId().toString();
// LogUtils.generate("Chamada REST Camunda Parametros: taskId = " + taskId + " user = " + user);
//
// Response response = getWebTarget()
// .path(TASK)
// .path(taskId)
// .path(CLAIM)
// .request()
// .post(Entity.json(Json.createObjectBuilder().add(USER_ID, user).build()));
//
// validResponse(response);
CDI.current().select(TaskService.class).get().claim(taskId, user);
}
public static void unClaim(String taskId) {
// LogUtils.generate("Chamada REST Camunda Parametros: taskId = " + taskId);
//
// Response response = getWebTarget()
// .path(TASK)
// .path(taskId)
// .path(UNCLAIM)
// .request()
// .post(Entity.text(""));
//
// validResponse(response);
CDI.current().select(TaskService.class).get().setAssignee(taskId, null);
}
public static void complete(String taskId) {
complete(taskId, Map.of());
}
public static void complete(String taskId, Map variables) {
// LogUtils.generate("Chamada REST Camunda Parametros: taskId = " + taskId + " variables " + variables.entrySet().stream().map(es -> "Chave: " + es.getKey() + " Valor: " + es.getValue()).collect(Collectors.joining(", ")));
//
// TaskBean taskBpm = getTaskBpm(taskId);
//
// if (taskBpm == null) {
// return;
// }
//
// String jsonVariable = toVariableJson(variables).build().toString();
//
// LogUtils.generate(jsonVariable);
//
// Response response = getWebTarget()
// .path(TASK)
// .path(taskId)
// .path("complete")
// .request(MediaType.APPLICATION_JSON_TYPE)
// .post(Entity.json(jsonVariable));
//
// validResponse(response);
CDI.current().select(TaskService.class).get().complete(taskId, variables);
}
public static void deleteCandidateUser(String taskId, String userId) {
// LogUtils.generate("Chamada REST Camunda Parametros: taskId = " + taskId + " userId = " + userId);
//
// Response response = getWebTarget()
// .path(TASK)
// .path(taskId)
// .path(IDENTITY_LINKS)
// .path(DELETE)
// .request(MediaType.APPLICATION_JSON_TYPE)
// .post(Entity.json(Json.createObjectBuilder().add(USER_ID, userId).add(TYPE, CANDIDATE).build()));
//
// validResponse(response);
CDI.current().select(TaskService.class).get().deleteCandidateUser(taskId, userId);
}
public static void deleteProcessInstance(String processInstance, String deleteReason) {
// LogUtils.generate("Chamada REST Camunda Parametros: processInstance = " + processInstance);
//
// Response response = getWebTarget()
// .path(PROCESS_INSTANCE)
// .path(processInstance)
// .queryParam("cascade", true)
// .request(MediaType.APPLICATION_JSON_TYPE)
// .delete();
//
// validResponse(response);
CDI.current().select(RuntimeService.class).get().deleteProcessInstance(processInstance, deleteReason);
}
public static List filterTask(String processDefinitionKey, Map queryParam) {
return filterTask(List.of(processDefinitionKey), queryParam);
}
public static List filterTask(List processDefinitionKey, Map queryParam) {
LogUtils.generate("Chamada REST Camunda Parametros: processDefinitionKey = " + processDefinitionKey.stream().collect(Collectors.joining(", ")) + " queryParam = " + queryParam.entrySet().stream().map(es -> "Chave: " + es.getKey() + " Valor: " + es.getValue()).collect(Collectors.joining(", ")));
LogUtils.generate(URL_REST_BPM);
LogUtils.generate(TASK);
LogUtils.generate(PROCESS_DEFINITION_KEY_IN + " = " + processDefinitionKey.stream().collect(Collectors.joining(",")));
WebTarget webTarget = BpmUtils.getWebTarget().
path(TASK)
.queryParam(PROCESS_DEFINITION_KEY_IN, processDefinitionKey.stream().collect(Collectors.joining(",")))
.queryParam(ACTIVE, true)
.queryParam(SORT_BY, PRIORITY)
.queryParam(SORT_ORDER, ASC)
.queryParam(SORT_BY, CREATED)
.queryParam(SORT_ORDER, ASC);
for (Map.Entry es : queryParam.entrySet()) {
webTarget = webTarget.queryParam(es.getKey(), es.getValue());
}
if (queryParam.isEmpty() || !queryParam.containsKey(PROCESS_VARIABLES)) {
webTarget = webTarget.queryParam(PROCESS_VARIABLES, getFilterTenantVariable());
}
Response response = webTarget
.request(MediaType.APPLICATION_JSON_TYPE)
.get();
String respostaServico = response.readEntity(String.class);
LogUtils.generate(respostaServico);
if (!isStatusOk(response)) {
throw new ValidationException(respostaServico);
}
return JsonbBuilder.create().fromJson(respostaServico, new ArrayList() {}.getClass().getGenericSuperclass());
}
public static List filterTaskAssignee(String processDefinitionKey) {
return filterTaskAssignee(List.of(processDefinitionKey));
}
public static List filterTaskAssignee(List processDefinitionKey) {
return filterTask(processDefinitionKey,
Map.of(ASSIGNEE, CDI.current().select(UserInformation.class).get().get().getId().toString()));
}
public static List filterTaskBusinessKey(String processDefinitionKey, String businessKey) {
return filterTask(processDefinitionKey, Map.of(PROCESS_INSTANCE_BUSINESS_KEY, businessKey));
}
public static List filterTaskInvolvedUser(String processDefinitionKey) {
return filterTaskInvolvedUser(List.of(processDefinitionKey));
}
public static List filterTaskInvolvedUser(List processDefinitionKey) {
return filterTask(processDefinitionKey,
Map.of(INVOLVED_USER, CDI.current().select(UserInformation.class).get().get().getId().toString()));
}
public static List filterTaskCandidateUser(String processDefinitionKey) {
return filterTaskCandidateUser(List.of(processDefinitionKey));
}
public static List filterTaskCandidateUser(List processDefinitionKey) {
return filterTask(processDefinitionKey,
Map.of(CANDIDATE_USER, CDI.current().select(UserInformation.class).get().get().getId().toString()));
}
public static List filterTaskCandidateGroup(List processDefinitionKey, List candidateGroups) {
return filterTask(processDefinitionKey,
Map.of(CANDIDATE_GROUPS, candidateGroups.stream().collect(Collectors.joining(","))));
}
public static List filterTaskCandidateGroup(List processDefinitionKey, List candidateGroups, Map variablesEquals) {
String processVariables = getVariablesPathEquals(variablesEquals);
return filterTask(processDefinitionKey,
Map.of(
// ASSIGNEE, CDI.current().select(UserInformation.class).get().get().getId().toString(),
PROCESS_VARIABLES, processVariables,
CANDIDATE_GROUP, candidateGroups.stream().collect(Collectors.joining(","))
));
}
public static List filterTaskVariables(String processDefinitionKey, Map variablesEquals) {
String processVariables = getVariablesPathEquals(variablesEquals);
return filterTask(processDefinitionKey, Map.of(PROCESS_VARIABLES, processVariables));
}
public static List filterProcessInstance(String processDefinitionKey, Map variablesEquals) {
String processVariables = getVariablesPathEquals(variablesEquals);
return filterProcessInstanceBase(List.of(processDefinitionKey), Map.of(VARIABLES, processVariables));
}
public static List filterProcessInstance(List processDefinitionKey, Map variablesEquals) {
String processVariables = getVariablesPathEquals(variablesEquals);
return filterProcessInstanceBase(processDefinitionKey, Map.of(VARIABLES, processVariables));
}
public static TaskBean getTaskBpm(String taskId) {
LogUtils.generate("Chamada REST Camunda Parametros: taskId = " + taskId);
Response response = getWebTarget()
.path(TASK)
.path(taskId)
.request(MediaType.APPLICATION_JSON_TYPE)
.get();
if (isStatusOk(response)) {
String json = response.readEntity(String.class);
return JsonbBuilder.create().fromJson(json, TaskBean.class);
}
return null;
}
public static String getPropertyFromProcessInstance(String processDefinitionKey, String businessKey, String property) {
LogUtils.generate("Chamada REST Camunda Parametros: processDefinitionKey = " + processDefinitionKey + " businessKey = " + businessKey + " property = " + property);
Response response = getWebTarget()
.path(PROCESS_INSTANCE)
.queryParam(PROCESS_DEFINITION_KEY, processDefinitionKey)
.queryParam(BUSINESS_KEY, businessKey)
.request(MediaType.APPLICATION_JSON_TYPE)
.get();
return getProperty(property, response);
}
public static String getPropertyFromProcessInstance(String processDefinitionKey, Map variablesEquals, String property) {
LogUtils.generate("Chamada REST Camunda Parametros: processDefinitionKey = " + processDefinitionKey + " variablesEquals = " + variablesEquals.entrySet().stream().map(es -> "Chave: " + es.getKey() + " Valor: " + es.getValue()).collect(Collectors.joining(", ")) + " property = " + property);
Response response = getWebTarget()
.path(PROCESS_INSTANCE)
.queryParam(PROCESS_DEFINITION_KEY, processDefinitionKey)
.queryParam(VARIABLES, getVariablesPathEquals(variablesEquals))
.request(MediaType.APPLICATION_JSON_TYPE)
.get();
return getProperty(property, response);
}
public static Collection getProcessDefinitionActiveLatestVersion() {
LogUtils.generate();
return getWebTarget()
.path(PROCESS_DEFINITION)
.queryParam(ACTIVE, true)
.queryParam(LATEST_VERSION, true)
.request(MediaType.APPLICATION_JSON)
.get()
.readEntity(List.class);
}
public static void processDefinitionStartByKey(String key, String businessKey, Map variables) {
// LogUtils.generate("Chamada REST Camunda Parametros: key = " + key + " businessKey = " + businessKey + " variables = " + variables.entrySet().stream().map(es -> "Chave: " + es.getKey() + " Valor: " + es.getValue()).collect(Collectors.joining(", ")));
//
// String variablesJson = toVariableJson(variables).add(BUSINESS_KEY, businessKey).build().toString();
//
// Response response = getWebTarget()
// .path(PROCESS_DEFINITION)
// .path(KEY)
// .path(key)
// .path(START)
// .request(MediaType.APPLICATION_JSON)
// .post(Entity.json(variablesJson));
//
// validResponse(response);
CDI.current().select(RuntimeService.class).get().startProcessInstanceByKey(key, businessKey, variables);
}
public static void setVariablesProcessInstance(String processInstanceId, String varName, Object value) {
setVariablesProcessInstance(processInstanceId, Map.of(varName, value));
}
public static void setVariablesProcessInstance(String processInstanceId, Map variables) {
// LogUtils.generate("Chamada REST Camunda Parametros: processInstanceId = " + processInstanceId + " variables = " + variables.entrySet().stream().map(es -> "Chave: " + es.getKey() + " Valor: " + es.getValue()).collect(Collectors.joining(", ")));
//
// String jsonFinal = getVariablesModification(variables);
//
// Response response = getWebTarget()
// .path(PROCESS_INSTANCE)
// .path(processInstanceId)
// .path(VARIABLES)
// .request(MediaType.APPLICATION_JSON)
// .post(Entity.json(jsonFinal));
//
// validResponse(response);
CDI.current().select(RuntimeService.class).get().setVariables(processInstanceId, variables);
}
public static void removeVariableProcessInstance(String processInstanceId, String variable) {
removeVariablesProcessInstance(processInstanceId, List.of(variable));
}
public static void removeVariablesProcessInstance(String processInstanceId, Collection variables) {
// LogUtils.generate("Chamada REST Camunda Parametros: processInstanceId = " + processInstanceId + " variables = " + variables.entrySet().stream().map(es -> "Chave: " + es.getKey() + " Valor: " + es.getValue()).collect(Collectors.joining(", ")));
//
// String jsonFinal = getVariablesModification(variables);
//
// Response response = getWebTarget()
// .path(PROCESS_INSTANCE)
// .path(processInstanceId)
// .path(VARIABLES)
// .request(MediaType.APPLICATION_JSON)
// .post(Entity.json(jsonFinal));
//
// validResponse(response);
CDI.current().select(RuntimeService.class).get().removeVariables(processInstanceId, variables);
}
public static void removeVariable(String id, String variable) {
removeVariablesProcessInstance(id, List.of(variable));
}
public static void removeVariables(String id, Collection variables) {
// LogUtils.generate("Chamada REST Camunda Parametros: processInstanceId = " + processInstanceId + " variables = " + variables.entrySet().stream().map(es -> "Chave: " + es.getKey() + " Valor: " + es.getValue()).collect(Collectors.joining(", ")));
//
// String jsonFinal = getVariablesModification(variables);
//
// Response response = getWebTarget()
// .path(PROCESS_INSTANCE)
// .path(processInstanceId)
// .path(VARIABLES)
// .request(MediaType.APPLICATION_JSON)
// .post(Entity.json(jsonFinal));
//
// validResponse(response);
CDI.current().select(RuntimeService.class).get().removeVariablesLocal(id, variables);
}
public static void setVariablesTask(String taskId, String varName, Object value) {
setVariablesTask(taskId, Map.of(varName, value));
}
public static void setVariablesTask(String taskId, Map variables) {
// LogUtils.generate("Chamada REST Camunda Parametros: taskId = " + taskId + " variables = " + variables.entrySet().stream().map(es -> "Chave: " + es.getKey() + " Valor: " + es.getValue()).collect(Collectors.joining(", ")));
//
// String jsonFinal = getVariablesModification(variables);
//
// Response response = getWebTarget()
// .path(TASK)
// .path(taskId)
// .path(VARIABLES)
// .request(MediaType.APPLICATION_JSON)
// .post(Entity.json(jsonFinal));
//
// validResponse(response);
CDI.current().select(TaskService.class).get().setVariables(taskId, variables);
}
public static void setVariablesTaskLocal(String taskId, String varName, Object value) {
LogUtils.generate("Chamada REST Camunda Parametros: taskId = " + taskId + " varName = " + varName + " Value: " + value);
setVariablesTask(taskId, Map.of(varName, value));
}
public static void setVariablesTaskLocal(String taskId, Map variables) {
// LogUtils.generate("Chamada REST Camunda Parametros: taskId = " + taskId + " variables = " + variables.entrySet().stream().map(es -> "Chave: " + es.getKey() + " Valor: " + es.getValue()).collect(Collectors.joining(", ")));
//
// String jsonFinal = getVariablesModification(variables);
//
// Response response = getWebTarget()
// .path(TASK)
// .path(taskId)
// .path(LOCAL_VARIABLES)
// .request(MediaType.APPLICATION_JSON)
// .post(Entity.json(jsonFinal));
//
// validResponse(response);
CDI.current().select(TaskService.class).get().setVariablesLocal(taskId, variables);
}
public static void signal(String signalName) {
// LogUtils.generate("Chamada REST Camunda Parametros: signalName = " + signalName);
//
// Response response = getWebTarget()
// .path("signal")
// .request(MediaType.APPLICATION_JSON)
// .post(Entity.json(Json.createObjectBuilder().add("name", signalName).build()));
//
// validResponse(response);
CDI.current().select(RuntimeService.class).get().createSignalEvent(signalName).send();
}
public static String getVariableProcessInstance(String id, String varName) {
return getVariable(PROCESS_INSTANCE, VARIABLES, id, varName);
}
public static Long getVariableProcessInstanceLong(String id, String varName) {
return getVariableLong(PROCESS_INSTANCE, VARIABLES, id, varName);
}
public static Boolean getVariableProcessInstanceBoolean(String id, String varName) {
return getVariableBoolean(PROCESS_INSTANCE, VARIABLES, id, varName);
}
public static String getVariableExecutionLocal(String id, String varName) {
return getVariable(EXECUTION, LOCAL_VARIABLES, id, varName);
}
public static Map> getVariablesExecutionLocal(String id) {
return getVariables(EXECUTION, LOCAL_VARIABLES, id);
}
public static Map getVariables(String processInstanceId) {
return CDI.current().select(RuntimeService.class).get().getVariables(processInstanceId);
}
public static Long getVariableExecutionLocalLong(String id, String varName) {
return getVariableLong(EXECUTION, LOCAL_VARIABLES, id, varName);
}
public static String getVariableTask(String id, String varName) {
return getVariable(TASK, VARIABLES, id, varName);
}
public static Long getVariableTaskLong(String id, String varName) {
return getVariableLong(TASK, VARIABLES, id, varName);
}
public static String getVariableTaskLocal(String id, String varName) {
return getVariable(TASK, LOCAL_VARIABLES, id, varName);
}
public static Map> getVariablesTaskLocal(String id) {
return getVariables(TASK, LOCAL_VARIABLES, id);
}
public static Long getVariableTaskLocalLong(String id, String varName) {
return getVariableLong(TASK, LOCAL_VARIABLES, id, varName);
}
public static void showDiagram(String taskId) {
TaskBean taskBpm = getTaskBpm(taskId);
if (taskBpm == null) {
return;
}
String json = getWebTarget()
.path(PROCESS_DEFINITION)
.path(taskBpm.getProcessDefinitionId())
.path("xml")
.request(MediaType.APPLICATION_JSON)
.get()
.readEntity(String.class);
JsfUtils.setAttributeSession("json", json);
JsfUtils.setAttributeSession("taskDefinitionKey", taskBpm.getTaskDefinitionKey());
JsfUtils.redirect("../bpmn/diagram.jsf?taskId=" + taskId);
}
public static boolean isStatusOk(Response response) {
return response.getStatus() >= 200
&& response.getStatus() < 300;
}
public static WebTarget getWebTarget() {
return ClientBuilder
.newClient()
.target(URL_REST_BPM);
}
private static String formatValue(Map.Entry entry) {
if (entry.getValue() == null) {
return "null";
}
String value;
var dateTimeFormater = DateTimeFormatter.ofPattern("yyyy-MM-dd'T'HH:mm:ss.SSSZ");
if (entry.getValue().getClass() == Date.class) {
value = DateUtils.toLocalDateTime((Date) entry.getValue()).atZone(ZoneId.systemDefault()).toLocalDateTime().atZone(ZoneId.systemDefault()).format(dateTimeFormater);
} else if (entry.getValue().getClass() == LocalDate.class) {
value = LocalDateTime.of((LocalDate) entry.getValue(), LocalTime.now()).atZone(ZoneId.systemDefault()).format(dateTimeFormater);
} else if (entry.getValue().getClass() == LocalDateTime.class) {
value = ((LocalDateTime) entry.getValue()).atZone(ZoneId.systemDefault()).format(dateTimeFormater);
} else {
value = entry.getValue().toString();
}
String delimiter = entry.getValue().getClass() == String.class
// || entry.getValue().getClass() == Date.class
// || entry.getValue().getClass() == LocalDate.class
// || entry.getValue().getClass() == LocalDateTime.class
? "\""
: "";
return delimiter + value + delimiter;
}
private static String getType(Class> aClass) {
if (Number.class.isAssignableFrom(aClass)
|| String.class.isAssignableFrom(aClass)
|| Date.class.isAssignableFrom(aClass)
|| Boolean.class.isAssignableFrom(aClass))
return aClass.getSimpleName();
return Object.class.getSimpleName();
}
private static JsonObjectBuilder getVariableObjectBuilder(Map.Entry entrySet/*, boolean addValueInfo*/) {
JsonObjectBuilder jsonVariable =
Json
.createObjectBuilder();
if (entrySet.getValue() == null) {
jsonVariable.addNull(VALUE);
jsonVariable.add(TYPE, "String");
return jsonVariable;
}
boolean addValueInfo = entrySet.getValue() != null && getType(entrySet.getValue().getClass()).equals("Object");
if (Integer.class.isAssignableFrom(entrySet.getValue().getClass()))
jsonVariable.add(VALUE, (Integer) entrySet.getValue());
else if (Long.class.isAssignableFrom(entrySet.getValue().getClass()))
jsonVariable.add(VALUE, (Long) entrySet.getValue());
else if (Double.class.isAssignableFrom(entrySet.getValue().getClass()))
jsonVariable.add(VALUE, (Double) entrySet.getValue());
else if (Date.class.isAssignableFrom(entrySet.getValue().getClass()))
jsonVariable.add(VALUE, formatValue(entrySet));
else if (Collection.class.isAssignableFrom(entrySet.getValue().getClass()))
jsonVariable.add(VALUE, "[" + ((Collection) entrySet
.getValue())
.stream()
.map(v -> (String.class.isAssignableFrom(v.getClass()) ? "\"" : "") + v.toString() + (String.class.isAssignableFrom(v.getClass()) ? "\"" : ""))
.collect(Collectors.joining(",")).toString() + "]");
else
jsonVariable.add(VALUE, entrySet.getValue().toString());
jsonVariable.add(TYPE, getType(entrySet.getValue().getClass()));
if (addValueInfo) {
JsonObjectBuilder jsonValueInfo =
Json
.createObjectBuilder()
.add(OBJECT_TYPE_NAME, entrySet.getValue().getClass().getName())
.add(SERIALIZATION_DATA_FORMAT, MediaType.APPLICATION_JSON);
jsonVariable.add(VALUE_INFO, jsonValueInfo.build());
}
return jsonVariable;
}
private static String getFilterTenantVariable() {
return "idMultiTenant_eq_" + CDI.current().select(MultiTenant.class).get().get();
}
private static String getVariablesModification(Map variables) {
JsonObjectBuilder jsonVariables = Json.createObjectBuilder();
variables
.entrySet()
.stream()
.forEach(entrySet -> {
JsonObjectBuilder jsonVariable = getVariableObjectBuilder(entrySet);
jsonVariables.add(entrySet.getKey(), jsonVariable);
}
);
return Json.createObjectBuilder().add(MODIFICATIONS, jsonVariables).build().toString();
}
private static JsonObjectBuilder toVariableJson(Map variables) {
if (variables.isEmpty()) {
return Json.createObjectBuilder();
}
JsonObjectBuilder jsonVariables = Json.createObjectBuilder();
variables
.entrySet()
.forEach(es -> jsonVariables.add(es.getKey(), getVariableObjectBuilder(es)));
return Json
.createObjectBuilder()
.add(VARIABLES, jsonVariables);
}
private static List filterProcessInstanceBase(String processDefinitionKey, Map queryParam) {
return filterProcessInstanceBase(List.of(processDefinitionKey), queryParam);
}
private static List filterProcessInstanceBase(List processDefinitionKeys, Map queryParam) {
LogUtils.generate("Chamada REST Camunda Parametros: processDefinitionKey = " + processDefinitionKeys.stream().collect(Collectors.joining(", ")) + " queryParam = " + queryParam.entrySet().stream().map(es -> "Chave: " + es.getKey() + " Valor: " + es.getValue()).collect(Collectors.joining(", ")));
WebTarget webTarget = BpmUtils.getWebTarget().
path(PROCESS_INSTANCE)
.queryParam(PROCESS_DEFINITION_KEY_IN, processDefinitionKeys.stream().collect(Collectors.joining(",")))
.queryParam(ACTIVE, true);
for (Map.Entry es : queryParam.entrySet()) {
webTarget = webTarget.queryParam(es.getKey(), es.getValue());
}
Response response = webTarget
.request(MediaType.APPLICATION_JSON_TYPE)
.get();
String respostaServico = response.readEntity(String.class);
if (!isStatusOk(response)) {
throw new ValidationException(respostaServico);
}
return JsonbBuilder.create().fromJson(respostaServico, new ArrayList() {}.getClass().getGenericSuperclass());
}
private static String getVariable(String pathRoot, String pathType, String id, String varName) {
LogUtils.generate("Chamada REST Camunda Parametros: pathRoot = " + pathRoot + " pathType = " + pathType + " id = " + id + " varName = " + varName);
if (!getVariables(pathRoot, pathType, id).containsKey(varName)) {
return null;
}
Response response = getWebTarget()
.path(pathRoot)
.path(id)
.path(pathType)
.path(varName)
.request(MediaType.APPLICATION_JSON_TYPE)
.get();
if (isStatusOk(response)) {
String json = response.readEntity(String.class);
try (JsonReader reader = Json.createReader(new StringReader(json))) {
JsonObject jsonObject = reader.readObject();
return jsonObject.getString(VALUE);
}
}
return null;
}
private static Map> getVariables(String pathRoot, String pathType, String id) {
LogUtils.generate("Chamada REST Camunda Parametros: pathRoot = " + pathRoot + " pathType = " + pathType + " id = " + id);
Response response = getWebTarget()
.path(pathRoot)
.path(id)
.path(pathType)
.request(MediaType.APPLICATION_JSON_TYPE)
.get();
if (isStatusOk(response)) {
return response.readEntity(Map.class);
}
return null;
}
private static Long getVariableLong(String pathRoot, String pathType, String id, String varName) {
LogUtils.generate("Chamada REST Camunda Parametros: pathRoot = " + pathRoot + " pathType = " + pathType + " id = " + id + " varName = " + varName);
if (!getVariables(pathRoot, pathType, id).containsKey(varName)) {
return null;
}
Response response = getWebTarget()
.path(pathRoot)
.path(id)
.path(pathType)
.path(varName)
.request(MediaType.APPLICATION_JSON_TYPE)
.get();
if (isStatusOk(response)) {
String json = response.readEntity(String.class);
try (JsonReader reader = Json.createReader(new StringReader(json))) {
JsonObject jsonObject = reader.readObject();
return jsonObject.getJsonNumber(VALUE).longValue();
}
}
return null;
}
private static Boolean getVariableBoolean(String pathRoot, String pathType, String id, String varName) {
LogUtils.generate("Chamada REST Camunda Parametros: pathRoot = " + pathRoot + " pathType = " + pathType + " id = " + id + " varName = " + varName);
if (!getVariables(pathRoot, pathType, id).containsKey(varName)) {
return null;
}
Response response = getWebTarget()
.path(pathRoot)
.path(id)
.path(pathType)
.path(varName)
.request(MediaType.APPLICATION_JSON_TYPE)
.get();
if (isStatusOk(response)) {
String json = response.readEntity(String.class);
try (JsonReader reader = Json.createReader(new StringReader(json))) {
JsonObject jsonObject = reader.readObject();
return jsonObject.getBoolean(VALUE);
}
}
return null;
}
private static void validResponse(Response response) {
if (!isStatusOk(response)) {
String messageErro;
String responseStr = response.readEntity(String.class);
try {
JsonReader reader = Json.createReader(new StringReader(responseStr));
JsonObject jsonObject = reader.readObject();
messageErro = jsonObject.getString("message");
} catch (Exception ex) {
messageErro = responseStr;
}
messageErro = messageErro.replace("javax.validation.ValidationException: ", "");
throw new ValidationException(messageErro);
}
}
private static String getVariablesPathEquals(Map variablesEquals) {
return getFilterTenantVariable() +
(variablesEquals.isEmpty() ? "" : ",") +
variablesEquals
.entrySet()
.stream()
.map(es -> es.getKey() + "_eq_" + es.getValue().toString())
.collect(Collectors.joining(","));
}
private static String getProperty(String property, Response response) {
if (isStatusOk(response)) {
String json = response.readEntity(String.class);
try (JsonReader reader = Json.createReader(new StringReader(json))) {
JsonArray jsonArray = reader.readArray();
if (!jsonArray.isEmpty()) {
return jsonArray.getJsonObject(0).getString(property);
}
}
}
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy