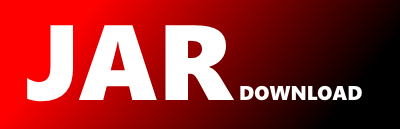
br.com.jarch.faces.ui.ButtonUI Maven / Gradle / Ivy
package br.com.jarch.faces.ui;
import br.com.jarch.core.exception.ValidationException;
import br.com.jarch.faces.util.JsfUtils;
import javax.el.MethodExpression;
import javax.faces.component.FacesComponent;
import javax.faces.component.UINamingContainer;
import javax.faces.context.FacesContext;
@FacesComponent("br.com.jarch.faces.ui.ButtonUI")
public class ButtonUI extends UINamingContainer {
public String methodAction() {
MethodExpression me = (MethodExpression) this.getAttributes().get("action");
if (me != null) {
try {
Object result = me.invoke(FacesContext.getCurrentInstance().getELContext(), null);
if (result instanceof String) {
return (String) result;
}
} catch (Exception ex) {
ex.printStackTrace();
if (ValidationException.class.isAssignableFrom(ex.getClass())
|| (ex.getCause() != null && ValidationException.class.isAssignableFrom(ex.getCause().getClass()))) {
throw ex;
}
Object result = JsfUtils.resolveExpressionLanguage(me.getExpressionString());
if (result instanceof String) {
return (String) result;
}
}
}
return null;
}
public void methodActionListener() {
MethodExpression me = (MethodExpression) this.getAttributes().get("actionListener");
if (me != null) {
me.invoke(FacesContext.getCurrentInstance().getELContext(), null);
}
}
public void methodListener() {
MethodExpression me = (MethodExpression) this.getAttributes().get("listener");
if (me != null) {
me.invoke(FacesContext.getCurrentInstance().getELContext(), null);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy