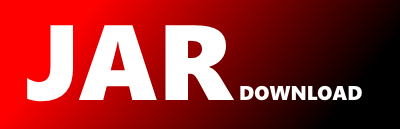
br.com.jarch.faces.ui.InputTextImUI Maven / Gradle / Ivy
package br.com.jarch.faces.ui;
import br.com.jarch.util.CharacterUtils;
import br.com.jarch.util.NumberUtils;
import br.com.jarch.util.StringUtils;
import org.primefaces.component.inputmask.InputMask;
import javax.faces.component.FacesComponent;
import javax.faces.component.NamingContainer;
import javax.faces.component.UIInput;
import javax.faces.context.FacesContext;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
@FacesComponent("br.com.jarch.faces.ui.InputTextImUI")
public class InputTextImUI extends UIInput implements NamingContainer {
private InputMask im;
@Override
public String getFamily() {
return "javax.faces.NamingContainer";
}
@Override
public void encodeBegin(FacesContext context) throws IOException {
applyMask();
super.encodeBegin(context);
}
public void applyMask() {
String value = getAttributeValue();
if (!StringUtils.isNullOrEmpty(value) && quantityDigitMask() > 0)
value = configMask();
im.setValue(value);
}
private String configZeroLeft() {
String value = getAttributeValue();
value = value.trim();
value = NumberUtils.onlyNumber(value);
value = CharacterUtils.alignTextRigth(value, quantityDigitMask(), "0");
return value;
}
private String configMask() {
String mask = getAttributeMask();
String value = configZeroLeft();
String digitsOnlyNumber = value.replaceAll("\\D", "");
int digitIndex = 0;
List formattedNumbers = new ArrayList<>();
for(int i = 0; i < mask.length(); ++i) {
char currentCharacter = mask.charAt(i);
if (currentCharacter == '9' && digitIndex < digitsOnlyNumber.length()) {
formattedNumbers.add(String.valueOf(digitsOnlyNumber.charAt(digitIndex++)));
} else if (currentCharacter != ']' && currentCharacter != '[') {
formattedNumbers.add(String.valueOf(currentCharacter));
}
}
return String.join("", formattedNumbers);
}
private String getAttributeMask() {
return getAttributes().getOrDefault("mask", "").toString();
}
private String getAttributeValue() {
return getAttributes().getOrDefault("value", "").toString();
}
private Integer quantityDigitMask() {
return NumberUtils.onlyNumber(getAttributeMask()).length();
}
@Override
public void setSubmittedValue(Object submittedValue) {
getAttributes().put("value", submittedValue);
im.setSubmittedValue(submittedValue);
}
public InputMask getIm() {
return im;
}
public void setIm(InputMask im) {
this.im = im;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy