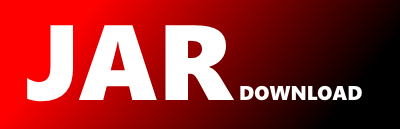
br.com.jarch.faces.ui.ListComponentUI Maven / Gradle / Ivy
package br.com.jarch.faces.ui;
import org.primefaces.event.CellEditEvent;
import javax.faces.application.FacesMessage;
import javax.faces.component.FacesComponent;
import javax.faces.component.NamingContainer;
import javax.faces.component.UIInput;
import javax.faces.component.UINamingContainer;
import javax.faces.context.FacesContext;
import java.util.ArrayList;
import java.util.Collection;
@FacesComponent("br.com.jarch.faces.ui.ListComponentUI")
public class ListComponentUI extends UIInput implements NamingContainer {
private String item = "";
private String itemRemove = "";
private Collection list = new ArrayList<>();
@Override
public String getFamily() {
return UINamingContainer.COMPONENT_FAMILY;
}
public void clear() {
item = "";
}
public void onCellEdit(CellEditEvent event) {
Object oldValue = event.getOldValue();
Object newValue = event.getNewValue();
if (newValue != null && !newValue.equals(oldValue)) {
FacesMessage msg = new FacesMessage(FacesMessage.SEVERITY_INFO, "Cell Changed", "Old: " + oldValue + ", New:" + newValue);
FacesContext.getCurrentInstance().addMessage(null, msg);
}
}
public String getItem() {
return item;
}
public void setItem(String item) {
this.item = item;
}
public String getItemRemove() {
return itemRemove;
}
public void setItemRemove(String itemRemove) {
this.itemRemove = itemRemove;
}
public Collection getList() {
return list;
}
public void setList(Collection list) {
this.list = list;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy