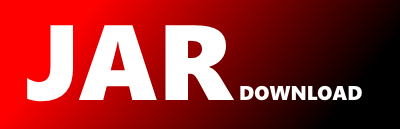
br.com.jarch.faces.util.MacAddressUtils Maven / Gradle / Ivy
package br.com.jarch.faces.util;
import br.com.jarch.util.LogUtils;
import javax.servlet.http.HttpServletRequest;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.LineNumberReader;
import java.util.Arrays;
import java.util.List;
import java.util.Locale;
import java.util.Scanner;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.stream.Collectors;
public final class MacAddressUtils {
public static final String ARP_GET_IP_HW = "arp -a";
private MacAddressUtils() {
}
public static boolean existsMacAdressEqual(String macAddress){
List listaMacAddress = getARPTable();
for (String item: listaMacAddress){
if ((item.toUpperCase(Locale.getDefault()).contains(macAddress.toUpperCase(Locale.getDefault()))
|| item.toUpperCase(Locale.getDefault()).contains(macAddress.toUpperCase(Locale.getDefault()).replaceAll("-", ":")))){
return true;
}
}
return false;
}
public static List getARPTable() {
String ipClient = getIpAddr();
String listaMacAddress;
try(Scanner s = new Scanner(Runtime.getRuntime().exec(ARP_GET_IP_HW).getInputStream()).useDelimiter("\\A")){
listaMacAddress = s.hasNext() ? s.next() : "";
} catch (IOException ex) {
LogUtils.generateSilent(ex);
listaMacAddress = "";
}
List lista = Arrays
.asList(listaMacAddress.split("\n"))
.stream()
.filter(line -> line.contains(ipClient))
.collect(Collectors.toList());
return lista;
}
public static String getIpAddr() {
String unknown = "unknown";
HttpServletRequest request = JsfUtils.getRequest();
String ip = request.getHeader("x-forwarded-for");
if(ip == null
|| ip.length() == 0
|| unknown.equalsIgnoreCase(ip)) {
ip = request.getHeader("Proxy-Client-IP");
}
if(ip == null
|| ip.length() == 0
|| unknown.equalsIgnoreCase(ip)) {
ip = request.getHeader("WL-Proxy-Client-IP");
}
if(ip == null
|| ip.length() == 0
|| unknown.equalsIgnoreCase(ip)) {
ip = request.getRemoteAddr();
}
return ip;
}
public static String getMacAddress(){
String ip = getIpAddr();
String macAddress = getMacAddress1(ip);
if (macAddress == null){
macAddress = getMacAddress2(ip);
}
if (macAddress == null){
macAddress = getMacAddress3();
}
if (macAddress == null){
macAddress = getMacAddress4(ip);
}
return macAddress;
}
private static String getMacAddress1(String ip){
// Find OS and set command according to OS
String OS = System.getProperty("os.name").toLowerCase();
String[] cmd;
Pattern macpt = null;
if (OS.contains("win")) {
// Windows
macpt = Pattern
.compile("[0-9a-f]+-[0-9a-f]+-[0-9a-f]+-[0-9a-f]+-[0-9a-f]+-[0-9a-f]+");
String[] a = { "arp", "-a", ip };
cmd = a;
} else {
// Mac OS X, Linux
macpt = Pattern
.compile("[0-9a-f]+:[0-9a-f]+:[0-9a-f]+:[0-9a-f]+:[0-9a-f]+:[0-9a-f]+");
String[] a = { "arp", ip };
cmd = a;
}
try {
// Run command
Process p = Runtime.getRuntime().exec(cmd);
p.waitFor();
// read output with BufferedReader
BufferedReader reader = new BufferedReader(new InputStreamReader(
p.getInputStream()));
String line = reader.readLine();
// Loop trough lines
while (line != null) {
Matcher m = macpt.matcher(line);
// when Matcher finds a Line then return it as result
if (m.find()) {
return m.group(0);
}
line = reader.readLine();
}
} catch (Exception ex) {
LogUtils.generate(ex);
}
return null;
}
private static String getMacAddress2(String ip){
String listaMacAddress;
try(Scanner s = new Scanner(Runtime.getRuntime().exec(ARP_GET_IP_HW).getInputStream()).useDelimiter("\\A")){
listaMacAddress = s.hasNext() ? s.next() : "";
} catch (IOException ex) {
LogUtils.generateSilent(ex);
listaMacAddress = "";
}
List lista = Arrays
.asList(listaMacAddress.split("\n"))
.stream()
.filter(line -> line.contains(ip))
.collect(Collectors.toList());
Pattern p = Pattern.compile("([\\w]{1,2}(-|:)){5}[\\w]{1,2}");
Matcher m = p.matcher(lista.stream().findAny().orElse(""));
if (m.find()) {
return m.group();
}
return null;
}
private static String getMacAddress3(){
try{
String macAddress = null;
String command = "ifconfig";
String osName = System.getProperty("os.name");
// System.out.println("Operating System is " + osName);
if (osName.startsWith("Windows")) {
command = "ipconfig /all";
} else if (osName.startsWith("Linux") || osName.startsWith("Mac") || osName.startsWith("HP-UX")
|| osName.startsWith("NeXTStep") || osName.startsWith("Solaris") || osName.startsWith("SunOS")
|| osName.startsWith("FreeBSD") || osName.startsWith("NetBSD")) {
command = "ifconfig -a";
} else if (osName.startsWith("OpenBSD")) {
command = "netstat -in";
} else if (osName.startsWith("IRIX") || osName.startsWith("AIX") || osName.startsWith("Tru64")) {
command = "netstat -ia";
} else if (osName.startsWith("Caldera") || osName.startsWith("UnixWare") || osName.startsWith("OpenUNIX")) {
command = "ndstat";
} else {// Note: Unsupported system.
// throw new Exception("The current operating system '" + osName + "' is not supported.");
}
Process pid = Runtime.getRuntime().exec(command);
BufferedReader in = new BufferedReader(new InputStreamReader(pid.getInputStream()));
Pattern p = Pattern.compile("([\\w]{1,2}(-|:)){5}[\\w]{1,2}");
while (true) {
String line = in.readLine();
// System.out.println("line " + line);
if (line == null)
break;
Matcher m = p.matcher(line);
if (m.find()) {
macAddress = m.group();
break;
}
}
in.close();
return macAddress;
} catch (Exception ex) {
LogUtils.generate(ex);
}
return null;
}
private static String getMacAddress4(String ip){
String str = "";
String macAddress = "";
try {
Process p = Runtime.getRuntime().exec("nbtstat -A " + ip);
InputStreamReader ir = new InputStreamReader(p.getInputStream());
LineNumberReader input = new LineNumberReader(ir);
for (int i = 1; i < 100; i++) {
str = input.readLine();
if (str != null) {
if (str.indexOf("MAC Address") > 1) {
macAddress = str.substring(str.indexOf("MAC Address") + 14, str.length());
break;
}
}
}
} catch (Exception ex) {
LogUtils.generate(ex);
}
return macAddress;
}
/*// exemplos
request.getRemoteAddr();
request.getRemoteHost();
request.getHeader("X-FORWARDED-FOR");
request.getHeader("x-real-ip");
Map result = new HashMap<>();
Enumeration headerNames = request.getHeaderNames();
while (headerNames.hasMoreElements()) {
String key = (String) headerNames.nextElement();
String value = request.getHeader(key);
result.put(key, value);
}
String ip = request.getHeader("X-Forwarded-For");
if (ip == null || ip.length() == 0 || ip.equalsIgnoreCase("unknown")) {
ip = request.getHeader("Proxy-Client-IP");
}
if (ip == null || ip.length() == 0 || ip.equalsIgnoreCase("unknown")) {
ip = request.getHeader("WL-Proxy-Client-IP");
}
if (ip == null || ip.length() == 0 || ip.equalsIgnoreCase("unknown")) {
ip = request.getHeader("HTTP_X_FORWARDED_FOR");
}
if (ip == null || ip.length() == 0 || ip.equalsIgnoreCase("unknown")) {
ip = request.getHeader("HTTP_X_FORWARDED");
}
if (ip == null || ip.length() == 0 || ip.equalsIgnoreCase("unknown")) {
ip = request.getHeader("HTTP_X_CLUSTER_CLIENT_IP");
}
if (ip == null || ip.length() == 0 || ip.equalsIgnoreCase("unknown")) {
ip = request.getHeader("HTTP_CLIENT_IP");
}
if (ip == null || ip.length() == 0 || ip.equalsIgnoreCase("unknown")) {
ip = request.getHeader("HTTP_FORWARDED_FOR");
}
if (ip == null || ip.length() == 0 || ip.equalsIgnoreCase("unknown")) {
ip = request.getHeader("HTTP_FORWARDED");
}
if (ip == null || ip.length() == 0 || ip.equalsIgnoreCase("unknown")) {
ip = request.getHeader("HTTP_VIA");
}
if (ip == null || ip.length() == 0 || ip.equalsIgnoreCase("unknown")) {
ip = request.getHeader("REMOTE_ADDR");
}
if (ip == null || ip.length() == 0 || ip.equalsIgnoreCase("unknown")) {
ip = request.getRemoteAddr();
}
*/
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy