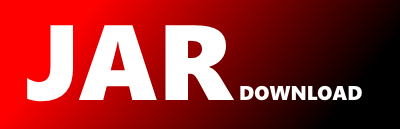
br.com.jarch.svn.BaseSvn Maven / Gradle / Ivy
The newest version!
package br.com.jarch.svn;
import org.tmatesoft.svn.core.SVNException;
import org.tmatesoft.svn.core.SVNNodeKind;
import org.tmatesoft.svn.core.SVNURL;
import org.tmatesoft.svn.core.auth.ISVNAuthenticationManager;
import org.tmatesoft.svn.core.internal.wc.DefaultSVNOptions;
import org.tmatesoft.svn.core.io.SVNRepository;
import org.tmatesoft.svn.core.io.SVNRepositoryFactory;
import org.tmatesoft.svn.core.wc.*;
import java.io.File;
import java.io.Serializable;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
abstract class BaseSvn implements Serializable {
private static final long serialVersionUID = -3496675246015308546L;
protected List log = new ArrayList<>();
protected String login;
protected String senha;
protected LogSvn logUtil = new LogSvn();
private SVNClientManager svnClientManager;
public BaseSvn() {
}
public BaseSvn(List log, String login, String senha) {
this.log = log;
this.login = login;
this.senha = senha;
}
private SVNClientManager getSvnClientManager() {
if (svnClientManager == null) {
DefaultSVNOptions defaultSVNOptions = new DefaultSVNOptions();
if (login == null || senha == null) {
svnClientManager = SVNClientManager.newInstance(defaultSVNOptions);
} else {
svnClientManager = SVNClientManager.newInstance(defaultSVNOptions, login, senha);
}
}
return svnClientManager;
}
public SVNWCClient getWCClient() {
return getSvnClientManager().getWCClient();
}
protected SVNStatusClient getStatusClient() {
return getSvnClientManager().getStatusClient();
}
protected SVNCommitClient getCommitClient() {
return getSvnClientManager().getCommitClient();
}
protected SVNUpdateClient getUpdateClient() {
return getSvnClientManager().getUpdateClient();
}
protected SVNCopyClient getCopyClient() {
return getSvnClientManager().getCopyClient();
}
protected SVNDiffClient getDiffClient() {
return getSvnClientManager().getDiffClient();
}
protected SVNLogClient getLogClient() {
return getSvnClientManager().getLogClient();
}
public boolean existeRepositorio(URL url) throws SVNException {
SVNRepository svnRepository = SVNRepositoryFactory.create(SVNURL.parseURIEncoded(url.toString()));
ISVNAuthenticationManager isvnAuthenticationManager = SVNWCUtil.createDefaultAuthenticationManager(login, senha.toCharArray());
svnRepository.setAuthenticationManager(isvnAuthenticationManager);
SVNNodeKind nodeKind = svnRepository.checkPath("", -1);
return nodeKind != SVNNodeKind.NONE;
}
public URL getRepositorio(File pastaWorkinkCopy) throws SVNException, MalformedURLException {
SVNInfo svnInfo = getWCClient().doInfo(pastaWorkinkCopy, SVNRevision.WORKING);
String url = svnInfo.getURL().toString();
if (!url.endsWith("/")) {
url += "/";
}
return new URL(url);
}
public List getLog() {
return log;
}
public void setLog(List log) {
this.log = log;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy