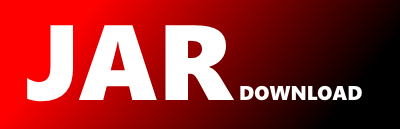
com.arch.util.CloneBeanUtils Maven / Gradle / Ivy
package com.arch.util;
import org.apache.commons.beanutils.BeanUtils;
import org.apache.commons.beanutils.PropertyUtils;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
public final class CloneBeanUtils {
private CloneBeanUtils() {
}
public static Object cloneBean(Class> classe, Object bean) {
try {
Object cloneBean = classe.cast(BeanUtils.cloneBean(bean));
return classe.cast(cloneBean(cloneBean, cloneBean, bean));
} catch (IllegalAccessException | InstantiationException | InvocationTargetException | NoSuchMethodException ex) {
throw new RuntimeException(ex);
}
}
private static Object cloneBean(Object object, Object cloneOriginalBean, Object originalBean) {
try {
Object cloneBean = object.getClass().newInstance();
Object bean = retornarObjeto(object);
Map, ?> describe = BeanUtils.describe(bean);
for (Map.Entry, ?> entry : describe.entrySet()) {
Object propertyValue = PropertyUtils.getProperty(bean, entry.getKey().toString());
if (propertyValue != null && !propertyValue.getClass().isAssignableFrom(Class.class)
&& PropertyUtils.isWriteable(cloneBean, entry.getKey().toString())) {
setProperty(cloneOriginalBean, originalBean, cloneBean, entry, propertyValue);
}
}
return cloneBean;
} catch (Exception ex) {
throw new RuntimeException(ex);
}
}
private static void setProperty(Object cloneOriginalBean, Object originalBean, Object cloneBean, Map.Entry, ?> entry, Object propertyValue) throws IllegalAccessException, InvocationTargetException, NoSuchMethodException {
if (isPrimitiveWrapper(propertyValue)
|| isByteArrayTimestampString(propertyValue)
|| propertyValue.getClass().isEnum()
|| propertyValue instanceof Collection>) {
PropertyUtils.setProperty(cloneBean, (String) entry.getKey(), propertyValue);
} else if (propertyValue.equals(originalBean)) {
PropertyUtils.setProperty(cloneBean, (String) entry.getKey(), cloneOriginalBean);
} else {
Object nestedCloneBean = cloneBean(propertyValue, cloneBean, originalBean);
PropertyUtils.setProperty(cloneBean, (String) entry.getKey(), nestedCloneBean);
}
}
private static boolean isByteArrayTimestampString(Object propertyValue) {
return propertyValue.getClass().isAssignableFrom(byte[].class)
|| propertyValue.getClass().isAssignableFrom(Date.class)
|| propertyValue.getClass().isAssignableFrom(String.class);
}
private static Object retornarObjeto(Object objeto) {
if (objeto == null) {
return null;
}
for (int i = 0; i < objeto.getClass().getDeclaredFields().length; i++) {
Field field = objeto.getClass().getDeclaredFields()[i];
if (field.getType().isAssignableFrom(List.class)) {
try {
Method metodo = metodoGet(objeto, field.getName());
Collection lista = (Collection) metodo.invoke(objeto);
} catch (NoSuchMethodException | IllegalAccessException | InvocationTargetException ex) {
boolean campoAcessivel = true;
if (!field.isAccessible()) {
field.setAccessible(true);
campoAcessivel = false;
}
setValueField(objeto, field);
if (!campoAcessivel) {
field.setAccessible(false);
}
}
}
}
return objeto;
}
private static void setValueField(Object objeto, Field field) {
try {
if (field.getType() == List.class) {
field.set(objeto, new ArrayList());
} else if (field.getType() == Set.class) {
field.set(objeto, new TreeSet<>());
}
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
private static Method metodoGet(Object objeto, String campo) throws NoSuchMethodException {
String methodName = "get" + Character.toUpperCase(campo.charAt(0)) + campo.substring(1);
return objeto.getClass().getMethod(methodName);
}
private static boolean isPrimitiveWrapper(Object propertyValue) {
return propertyValue.getClass().isAssignableFrom(Character.class)
|| propertyValue.getClass().isAssignableFrom(Number.class)
|| propertyValue.getClass().isAssignableFrom(byte[].class)
|| propertyValue.getClass().isAssignableFrom(Boolean.class);
}
public static String createToStringFor(Object obj) {
StringBuilder sb = new StringBuilder(obj.getClass().getSimpleName() + ": [");
Field[] fields = obj.getClass().getDeclaredFields();
for (Field field : fields) {
sb.append(field.getName()).append(": ");
field.setAccessible(true);
try {
sb.append(field.get(obj)).append(", ");
} catch (IllegalArgumentException | IllegalAccessException e) {
e.printStackTrace();
sb.append(" , ");
}
field.setAccessible(false);
}
String aRetornar = sb.substring(0, sb.length() - 2);
return aRetornar + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy