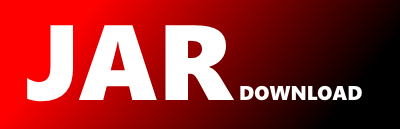
com.arch.util.ConexaoInternetUtils Maven / Gradle / Ivy
package com.arch.util;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.URL;
import java.util.Scanner;
public class ConexaoInternetUtils {
static public String ipInternet() {
BufferedReader buffer = null;
try {
URL url = new URL("http://www.meuenderecoip.com");
InputStreamReader in = new InputStreamReader(url.openStream());
buffer = new BufferedReader(in);
String line = buffer.readLine();
while (line != null) {
if (line.indexOf("Meu IP - Meu") > 0) {
line = buffer.readLine();
String ip = line.substring(line.indexOf(">") + 1, line.indexOf(""));
return ip;
}
line = buffer.readLine();
}
return "";
} catch (Exception ex) {
return "";
} finally {
try {
if (buffer != null) {
buffer.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
static public float tempoResposta(String ip, int tempoPerdaPacote) {
float retorno = 0f;
for (int i = 0; i < 5; i++) {
String resposta = "";
int fim = 0;
boolean kbo = false;
String comando;
if (System.getProperty("os.name").toLowerCase().indexOf("window") > -1) {
comando = new String("ping -n 1 " + ip);
} else {
comando = new String("ping -c 1 " + ip);
}
try (Scanner s = new Scanner(Runtime.getRuntime().exec(comando).getInputStream())) {
while (s.hasNextLine()) {
resposta = s.nextLine() + "\n";
fim = resposta.length() - 8;
for (int j = 0; j <= fim; j++) {
if (resposta.toLowerCase().indexOf("ttl=") > -1) {
kbo = true;
break;
}
}
if (kbo == true) {
break;
} else {
resposta = "HOST DOWN";
}
}
int posicaoInicio;
int posicaoFim;
if (resposta.indexOf("tempo") < 0) {
posicaoInicio = resposta.indexOf("time") + 5;
posicaoFim = resposta.indexOf(" ms");
} else {
posicaoInicio = resposta.indexOf("tempo") + 6;
posicaoFim = resposta.indexOf("ms TTL");
}
String tempo = resposta.substring(posicaoInicio, posicaoFim);
retorno += Float.parseFloat(tempo);
} catch (Exception e) {
retorno += tempoPerdaPacote;
}
}
return retorno / 5;
}
public enum Tipo {OTIMO, BOM, REGULAR, RUIM, PESSIMO, ANALISANDO}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy