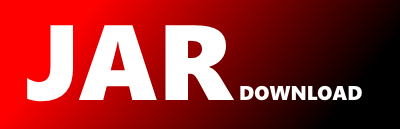
com.arch.util.DateUtils Maven / Gradle / Ivy
package com.arch.util;
import java.time.Instant;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.YearMonth;
import java.time.ZoneId;
import java.time.format.DateTimeFormatter;
import java.util.Date;
/**
* Created by wagner.araujo on 7/20/16.
*/
public final class DateUtils {
private DateUtils() {
//
}
public static Date toDate(LocalDate localDate) {
Instant instant = localDate.atStartOfDay().atZone(ZoneId.systemDefault()).toInstant();
return Date.from(instant);
}
public static Date toDateStartOfDay(LocalDate localDate) {
Instant instant = localDate.atStartOfDay().atZone(ZoneId.systemDefault()).toInstant();
return Date.from(instant);
}
public static Date toDateStartOfDay(Date data) {
LocalDateTime localDataHora = LocalDateTime.ofInstant(data.toInstant(), ZoneId.systemDefault()).withHour(0).withMinute(0).withSecond(0);
return Date.from(localDataHora.atZone(ZoneId.systemDefault()).toInstant());
}
public static Date toDateEndOfDay(LocalDate localDate) {
Instant instant = LocalDateTime.of(localDate, LocalTime.MAX).atZone(ZoneId.systemDefault()).toInstant();
return Date.from(instant);
}
public static Date toDateEndOfDay(Date data) {
LocalDateTime localDataHora = LocalDateTime.ofInstant(data.toInstant(), ZoneId.systemDefault()).withHour(23).withMinute(59).withSecond(59);
return Date.from(localDataHora.atZone(ZoneId.systemDefault()).toInstant());
}
public static Date toDate(LocalDateTime localDateTime) {
Instant instant = localDateTime.atZone(ZoneId.systemDefault()).toInstant();
return Date.from(instant);
}
public static LocalDate toLocalDate(Date date) {
Instant instant = Instant.ofEpochMilli(date.getTime());
return LocalDateTime.ofInstant(instant, ZoneId.systemDefault()).toLocalDate();
}
public static LocalDateTime toLocalDateTime(Date d) {
Instant instant = Instant.ofEpochMilli(d.getTime());
return LocalDateTime.ofInstant(instant, ZoneId.systemDefault());
}
public static LocalDateTime toLocalDateTimeStartOfDay(Date d) {
Instant instant = Instant.ofEpochMilli(d.getTime());
return LocalDateTime.ofInstant(instant, ZoneId.systemDefault()).toLocalDate().atStartOfDay();
}
public static LocalDateTime toLocalDateTimeEndOfDay(Date d) {
Instant instant = Instant.ofEpochMilli(d.getTime());
return LocalDateTime.ofInstant(instant, ZoneId.systemDefault()).toLocalDate().atTime(LocalTime.MAX);
}
public static Integer year() {
return LocalDate.now().getYear();
}
public static String formatMMyyyy(YearMonth yearMonth) {
return yearMonth.format(DateTimeFormatter.ofPattern("MM/yyyy"));
}
public static String formatddMMyyyy(LocalDate localDate) {
return localDate.format(DateTimeFormatter.ofPattern("dd/MM/yyyy"));
}
public static String formatddMMyyyyHHmm(LocalDateTime localDateTime) {
return localDateTime.format(DateTimeFormatter.ofPattern("dd/MM/yyyy HH:mm"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy