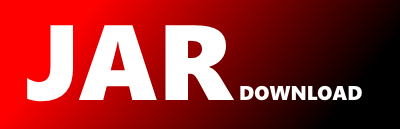
com.arch.util.ExcelUtils Maven / Gradle / Ivy
package com.arch.util;
import com.arch.util.annotation.ArchColumnExcel;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.IOException;
import java.io.OutputStream;
import java.lang.reflect.Field;
import java.util.Arrays;
import java.util.Collection;
import java.util.Comparator;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class ExcelUtils {
public static void generate(String namePlain, String title, Collection> listData, OutputStream outputStream) {
try {
if (listData.isEmpty()) {
return;
}
XSSFWorkbook wb = createWorkbook();
XSSFSheet sheet = createSheet(wb, namePlain);
createLineHeader(title, sheet);
createLineColumnHeader(listData, sheet);
createLineColumnDetail(listData, sheet);
saveWorkbook(wb, outputStream);
// wb.close()
// saida.flush()
} catch (IOException ex) {
LogUtils.generate(ex);
}
}
private static XSSFWorkbook createWorkbook() {
return new XSSFWorkbook();
}
private static XSSFSheet createSheet(XSSFWorkbook wb, String namePlain) {
return wb.createSheet(namePlain);
}
private static void createLineHeader(String title, XSSFSheet sheet) {
sheet.createRow(0);
addValueCell(sheet, title);
}
private static void createLineColumnHeader(Collection> listData, XSSFSheet sheet) {
sheet.createRow(sheet.getLastRowNum() + 1);
Object data = listData
.stream()
.findAny()
.orElse(null);
Collection> collection = dataCollection(BandLocation.HEADER, data);
collection
.forEach(column -> {
if (Map.Entry.class.isAssignableFrom(column.getClass())) {
addValueCell(sheet, ((Map.Entry, ?>) column).getKey());
} else {
String title = ((ArchColumnExcel) column).title();
addValueCell(sheet, title);
}
}
);
}
private static void createLineColumnDetail(Collection> listData, XSSFSheet sheet) {
listData
.forEach(
itemLinha -> {
sheet.createRow(sheet.getLastRowNum() + 1);
Collection> collection = dataCollection(BandLocation.DETAIL, itemLinha);
collection
.forEach(
column -> {
if (Map.Entry.class.isAssignableFrom(column.getClass())) {
addValueCell(sheet, ((Map.Entry, ?>) column).getValue());
} else {
Object conteudo = ReflectionUtils.getValueByField(itemLinha, (Field) column);
if (conteudo != null) {
addValueCell(sheet, conteudo);
}
}
}
);
}
);
}
private static void saveWorkbook(XSSFWorkbook wb, OutputStream outputStream) throws IOException {
wb.write(outputStream);
}
private static void addValueCell(XSSFSheet sheet, Object value) {
short lastCell = sheet
.getRow(sheet.getLastRowNum())
.getLastCellNum();
lastCell = lastCell < 0 ? 0 : lastCell;
sheet
.getRow(sheet.getLastRowNum())
.createCell(lastCell)
.setCellValue("" + value);
}
private static Collection> dataCollection(BandLocation bandLocation, Object data) {
Collection> collection;
if (Map.class.isAssignableFrom(data.getClass())) {
collection = ((Map, ?>) data)
.entrySet()
.stream()
.collect(Collectors.toList());
} else {
Stream> stream = Arrays
.stream(data
.getClass()
.getDeclaredFields())
.filter(field -> field.isAnnotationPresent(ArchColumnExcel.class));
if (bandLocation == BandLocation.HEADER) {
stream = stream
.map(field -> ((Field) field).getAnnotation(ArchColumnExcel.class))
.sorted(Comparator.comparingInt(ArchColumnExcel::order));
} else {
stream = stream
.sorted((field1, field2) ->
{
ArchColumnExcel column1 = ((Field) field1).getAnnotation(ArchColumnExcel.class);
ArchColumnExcel column2 = ((Field) field2).getAnnotation(ArchColumnExcel.class);
return Integer.compare(column1.order(), column2.order());
}
);
}
collection = stream.collect(Collectors.toList());
}
return collection;
}
private enum BandLocation {
HEADER, DETAIL
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy