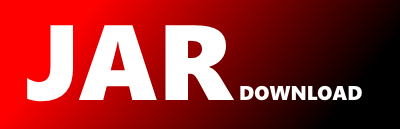
com.arch.util.JpaUtils Maven / Gradle / Ivy
package com.arch.util;
import org.hibernate.Hibernate;
import org.hibernate.annotations.Filter;
import org.hibernate.annotations.Where;
import javax.persistence.CascadeType;
import javax.persistence.EmbeddedId;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.OneToMany;
import javax.persistence.OneToOne;
import javax.persistence.metamodel.EntityType;
import java.lang.reflect.Field;
import java.util.Arrays;
import java.util.Collection;
public class JpaUtils {
public static final String LOGIC_EXCLUSION = "logicExclusion";
public static final String WHERE_LOGIC_EXCLUSION = "dh_exclusao IS NULL";
public static String nameEntity(Class> clazz) {
Entity anotacao = clazz.getAnnotation(Entity.class);
if (anotacao.name() == null || anotacao.name().isEmpty()) {
return clazz.getName();
}
return anotacao.name();
}
public static String aliasEntity(Class> clazz) {
Entity anotacao = clazz.getAnnotation(Entity.class);
return anotacao.name() == null || anotacao.name().isEmpty()
// ? CaracterUtils.firstCaracterLowerCase(clazz.getSimpleName())
? clazz.getSimpleName()
: anotacao.name();
}
public static boolean isCollectionOneToManyWithCascadeAllOrCascadeRemove(Object entity, Field field) {
return collectionOneToManyWithCascade(entity, field, CascadeType.REMOVE);
}
public static boolean collectionOneToManyWithCascadeAllOrCascadeMerge(Object entity, Field field) {
return collectionOneToManyWithCascade(entity, field, CascadeType.MERGE);
}
public static boolean collectioOneToManyWithLazy(Field field) {
if (!ReflectionUtils.isCollection(field)) {
return false;
}
if (!field.isAnnotationPresent(OneToMany.class)) {
return false;
}
OneToMany oneToMany = field.getAnnotation(OneToMany.class);
return oneToMany.fetch() == FetchType.LAZY;
}
public static boolean isExclusionLogic(Class> clazzEntity) {
Where whereAnnotation = clazzEntity.getAnnotation(Where.class);
if ((whereAnnotation != null && (whereAnnotation.clause().equals(WHERE_LOGIC_EXCLUSION)))) {
return true;
}
return Arrays
.stream(clazzEntity.getAnnotationsByType(Filter.class))
.anyMatch(a -> a.name().equals(LOGIC_EXCLUSION) && a.condition().equals(WHERE_LOGIC_EXCLUSION));
}
public static boolean deletePhysical(Class> clazzEntity) {
return !isExclusionLogic(clazzEntity);
}
public static String attributeId(Class> clazzEntity) {
return Arrays
.stream(clazzEntity.getDeclaredFields())
.filter(f -> f.isAnnotationPresent(EmbeddedId.class))
.map(f -> aliasEntity(clazzEntity) + "." + f.getName())
.findAny()
.orElse(aliasEntity(clazzEntity));
}
public static boolean isOneToManyAndCascadeRemove(Object entityMaster, EntityType> entiyType) {
for (Field field : entityMaster.getClass().getDeclaredFields()) {
if (!Collection.class.isAssignableFrom(field.getType())) {
continue;
}
if (!ReflectionUtils.getGenericClass(field).equals(entiyType.getJavaType())) {
continue;
}
OneToMany oneToMany = field.getAnnotation(OneToMany.class);
if (oneToMany == null) {
continue;
}
return Arrays
.stream(oneToMany.cascade())
.anyMatch(c -> CascadeType.ALL.equals(c) || CascadeType.REMOVE.equals(c));
}
return false;
}
public static boolean isOneToOneAndCascadeRemove(Object entityMaster, EntityType> entiyType) {
for (Field field : entityMaster.getClass().getDeclaredFields()) {
if (!ReflectionUtils.getGenericClass(field).equals(entiyType.getJavaType())) {
continue;
}
OneToOne oneToOne = field.getAnnotation(OneToOne.class);
if (oneToOne == null) {
continue;
}
return Arrays
.stream(oneToOne.cascade())
.anyMatch(c -> CascadeType.ALL.equals(c) || CascadeType.REMOVE.equals(c));
}
return false;
}
public static boolean isOneToOneAndMappedBy(Class> classMaster, EntityType> entiyType) {
for (Field field : entiyType.getJavaType().getDeclaredFields()) {
if (!ReflectionUtils.getGenericClass(field).equals(classMaster)) {
continue;
}
OneToOne oneToOne = field.getAnnotation(OneToOne.class);
if (oneToOne == null) {
continue;
}
LogUtils.warning("Field = " + field.getName() + " / " + field.getType() + " tem mappedBy " + !oneToOne.mappedBy().isEmpty());
return !oneToOne.mappedBy().isEmpty();
}
return false;
}
public static boolean isCollectionOneToMany(Object entity, Field field) {
boolean result = ReflectionUtils.isCollection(field);
result &= isObjectInitialize(entity, field);
result &= field.isAnnotationPresent(OneToMany.class);
return result;
}
public static boolean collectionOneToManyWithCascade(Object entity, Field field, CascadeType cascadeAcao) {
if (!isCollectionOneToMany(entity, field)) {
return false;
}
OneToMany oneToMany = field.getAnnotation(OneToMany.class);
boolean cascade = false;
for (CascadeType cascadeType : oneToMany.cascade()) {
if (cascadeType.equals(CascadeType.ALL) || cascadeType.equals(cascadeAcao)) {
cascade = true;
break;
}
}
return cascade;
}
public static boolean isObjectInitialize(Object entity, Field field) {
try {
field.setAccessible(true);
return Hibernate.isInitialized(field.get(entity));
} catch (SecurityException | IllegalAccessException ex) {
LogUtils.generate(ex);
return false;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy