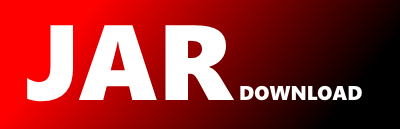
com.arch.util.LineCommandUtils Maven / Gradle / Ivy
package com.arch.util;
import java.io.BufferedReader;
import java.io.Closeable;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
public final class LineCommandUtils {
private LineCommandUtils() {
}
public static int executaCommando(final String command) throws InterruptedException, IOException {
return executaCommando(command, null);
}
public static int executaCommando(final String command, StringBuilder log) throws InterruptedException, IOException {
final ArrayList commands = new ArrayList<>();
if (OperationSystemUtils.windows()) {
commands.add("cmd");
commands.add("/C");
} else {
commands.add("/bin/bash");
commands.add("-c");
}
commands.add(command);
BufferedReader br = null;
final ProcessBuilder p = new ProcessBuilder(commands);
final Process process = p.start();
try (final InputStream is = process.getInputStream();
final InputStreamReader isr = new InputStreamReader(is)) {
br = new BufferedReader(isr);
String line;
String ultimaLinha = "1";
while ((line = br.readLine()) != null) {
LogUtils.generate(line);
if (log != null) {
if (!"".equals(log.toString())) {
log.append("\n");
}
log.append(line);
}
ultimaLinha = line;
}
LogUtils.warning(ultimaLinha);
process.waitFor();
return process.exitValue();
} finally {
secureClose(br);
}
}
private static void secureClose(final Closeable resource) {
try {
if (resource != null) {
resource.close();
}
} catch (IOException ex) {
LogUtils.generate(ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy