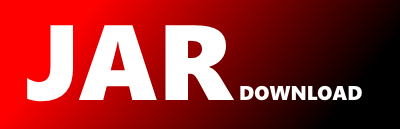
com.arch.util.LogUtils Maven / Gradle / Ivy
package com.arch.util;
import java.text.SimpleDateFormat;
import java.util.Date;
public final class LogUtils {
private static final String SEPARATOR = "########################################################################################\n";
private static final int STACKTRACE_INITIAL = 2;
private static final int STACKTRACE_FINAL = 3;
private static int posicao = STACKTRACE_INITIAL;
private LogUtils() {
super();
}
public static void start() {
posicao = STACKTRACE_FINAL;
generate("##### * * * I N I C I O * * * #####");
}
public static void end() {
posicao = STACKTRACE_FINAL;
generate("##### * * * F I M * * * #####");
}
public static void generate(String msg) {
String nomeClasseChamador = clazzCall();
String nomeMetodoChamador = methodCall();
int linhaChamador = lineCall();
generate(nomeClasseChamador, nomeMetodoChamador, linhaChamador, msg, true);
}
public static void generate() {
String nomeClasseChamador = clazzCall();
String nomeMetodoChamador = methodCall();
int linhaChamador = lineCall();
generate(nomeClasseChamador, nomeMetodoChamador, linhaChamador, "*** PONTO DE LOG ***", true);
}
public static void warning(String descricao) {
String nomeClasseChamador = clazzCall();
String nomeMetodoChamador = methodCall();
int linhaChamador = lineCall();
generate(nomeClasseChamador,
nomeMetodoChamador,
linhaChamador,
"\n" +
SEPARATOR +
SEPARATOR +
"####### ---> " + descricao + "\n" +
SEPARATOR +
SEPARATOR,
true);
}
public static void warning() {
String nomeClasseChamador = clazzCall();
String nomeMetodoChamador = methodCall();
int linhaChamador = lineCall();
generate(nomeClasseChamador,
nomeMetodoChamador,
linhaChamador,
"\n" +
SEPARATOR +
SEPARATOR +
"####### ---> PONTO DE LOG\n" +
SEPARATOR +
SEPARATOR,
true);
}
public static void generate(Exception ex) {
ex.printStackTrace();
}
public static void generateSilent(Exception ex) {
//
}
private static String methodCall() {
Throwable thr = new Throwable();
thr.fillInStackTrace();
StackTraceElement[] ste = thr.getStackTrace();
return ste[posicao].getMethodName();
}
private static String clazzCall() {
Throwable thr = new Throwable();
thr.fillInStackTrace();
StackTraceElement[] ste = thr.getStackTrace();
String retorno = ste[posicao].getClassName();
if (retorno.contains(".")) { // Pegar o nome da classe sem o pacote...
retorno = retorno.substring(retorno.lastIndexOf('.') + 1);
}
return retorno;
}
private static int lineCall() {
Throwable thr = new Throwable();
thr.fillInStackTrace();
StackTraceElement[] ste = thr.getStackTrace();
return ste[posicao].getLineNumber();
}
private static void generate(String clazz, String method, int row, String message, boolean showMessage) {
if (showMessage) {
System.out.println(timeLocal() + " - " + clazz + ":" + row + " - " + method + " - " + message);
}
posicao = STACKTRACE_INITIAL;
}
private static String timeLocal() {
return new SimpleDateFormat("HH:mm:ss").format(new Date());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy