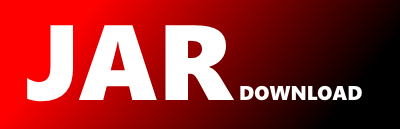
com.arch.util.MessagePropertiesUtils Maven / Gradle / Ivy
package com.arch.util;
import java.text.MessageFormat;
import java.util.MissingResourceException;
import java.util.ResourceBundle;
public class MessagePropertiesUtils {
private MessagePropertiesUtils() {
super();
}
public static String messageBundle(String baseName, String key) {
try {
return ResourceBundle.getBundle(baseName).getString(key);
} catch (RuntimeException ex) {
try {
return ResourceBundle.getBundle(baseName + "Jarch").getString(key);
} catch (MissingResourceException mse) {
// LogUtils.generate("NAO LOCALIZADO NO BUNDLE " + key + " LOCALE: " + Locale.getDefault());
} catch (RuntimeException ex2) {
// LogUtils.generate("NAO LOCALIZADO NO BUNDLE " + key + " LOCALE: " + getResourceBundle().getLocale().toString() + " VARIANT: " + getResourceBundle().getLocale().getVariant());
}
}
return "???" + key + "???";
}
public static String messageBundleParam(String baseName, String key, String parametro) {
return String.format(messageBundle(baseName, key), messageBundle(baseName, parametro));
}
public static String messageBundle(String baseName, String key, Object... parameters) {
String value = String.format(messageBundle(baseName, key), parameters);
Object[] params = messageBundleParameters(baseName, parameters);
try {
return MessageFormat.format(value, params);
} catch (MissingResourceException ex) {
// LogUtils.warning("NAO LOCALIZADO NO BUNDLE " + key);
}
return "???" + key + "???";
}
private static Object[] messageBundleParameters(String baseName, Object[] parametros) {
if (parametros == null || parametros.length < 1) {
return parametros;
}
for (int i = 0; i < parametros.length; i++) {
if (parametros[i] instanceof String) {
String parametro = (String) parametros[i];
if (parametro.startsWith("#")) {
parametros[i] = parametro.substring(1);
} else {
parametros[i] = messageBundle(baseName, parametro);
}
}
}
return parametros;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy