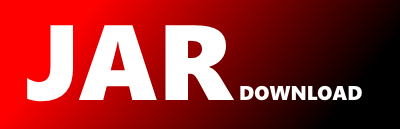
com.arch.util.RequestHttpUtils Maven / Gradle / Ivy
/*
package br.com.jarch.util;
import java.io.BufferedReader;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.StringWriter;
import java.io.Writer;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.Map;
import java.util.Set;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.codehaus.jackson.map.ObjectMapper;
public class RequestHttpUtils {
private static final String APPLICATION_JSON = "application/json";
private static final String CONTENT_TYPE = "Content-type";
public static final int CONTENT_LENGTH = 5_000;
public static final int CONNECT_TIMEOUT = 60_000;
public static void main(String[] args) {
try {
String retorno = getUrl("https://kanbanflow.com/api/v1/tasks?apiToken=e684cc66012011522f90eb9d41d93da8");
LogUtils.generate(retorno);
} catch (IOException ex) {
Logger.getLogger(RequestHttpUtils.class.getName()).log(Level.SEVERE, null, ex);
}
}
public static String getUrl(URL url) throws IOException {
return processaUrl(url, MetodoType.GET, null);
}
public static String getUrl(String value) throws IOException {
String url = value.replace(" ", "+");
return processaUrl(new URL(url), MetodoType.GET, null);
}
public static String getUrl(URL url, Map parametros) throws IOException {
return processaUrl(url, MetodoType.GET, parametros);
}
public static String getUrl(String value, Map parametros) throws IOException {
String url = value.replace(" ", "+");
return processaUrl(new URL(url), MetodoType.GET, parametros);
}
public static String postUrl(String value) throws IOException {
String url = value.replace(" ", "+");
return processaUrl(new URL(url), MetodoType.POST, null);
}
public static String postUrl(URL url) throws IOException {
return processaUrl(url, MetodoType.POST, null);
}
public static String postUrl(String value, Map parametros) throws IOException {
String url = value.replace(" ", "+");
return processaUrl(new URL(url), MetodoType.POST, parametros);
}
public static String postUrl(URL url, Map parametros) throws IOException {
return processaUrl(url, MetodoType.POST, parametros);
}
private static String processaUrl(URL url, MetodoType metodoType, Map parametros) throws IOException {
LogUtils.start();
LogUtils.generate(url.toString());
HttpURLConnection huc = (HttpURLConnection) url.openConnection();
HttpURLConnection.setFollowRedirects(false);
huc.setDoInput(true);
huc.setDoOutput(true);
huc.setUseCaches(false);
System.setProperty("http.keepAlive", "false");
huc.setRequestProperty("Content-length", String.valueOf(CONTENT_LENGTH));
huc.setConnectTimeout(CONNECT_TIMEOUT);
huc.setReadTimeout(CONNECT_TIMEOUT);
huc.setRequestMethod(metodoType.name());
if (parametros != null) {
Set chaves = parametros.keySet();
LogUtils.generate("Parametros");
for (String chave : chaves) {
Object conteudo = parametros.get(chave);
huc.addRequestProperty(chave, conteudo.toString());
LogUtils.generate(" Chave: " + chave + " Conteudo: " + conteudo);
}
}
LogUtils.generate("Conectando");
huc.connect();
LogUtils.generate("Conectado");
InputStream input = huc.getInputStream();
InputStreamReader inputReader = new InputStreamReader(input);
BufferedReader bufferedReader = new BufferedReader(inputReader);
LogUtils.generate("**** RETORNO DA PAGINA WEB ****");
StringBuilder sbRetorno = new StringBuilder();
String linha;
while ((linha = bufferedReader.readLine()) != null) {
LogUtils.generate(linha);
sbRetorno.append(linha);
}
LogUtils.generate("Fim");
return sbRetorno.toString();
}
public static T processaGet(URL url, Object objeto, Class classeRetorno) throws IOException {
return processaRequisicao(MetodoType.GET, url, objeto, classeRetorno);
}
public static T processaPost(URL url, Object objeto, Class classeRetorno) throws IOException {
return processaRequisicao(MetodoType.POST, url, objeto, classeRetorno);
}
private static T processaRequisicao(MetodoType metodoType, URL url, Object objeto, Class classeRetorno) throws IOException {
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setDoOutput(true);
connection.setDoInput(true);
connection.setInstanceFollowRedirects(false);
connection.setRequestMethod(metodoType.name());
connection.setRequestProperty(CONTENT_TYPE, APPLICATION_JSON);
connection.setUseCaches(false);
if (objeto != null) {
DataOutputStream wr = new DataOutputStream(connection.getOutputStream());
ObjectMapper mapper = new ObjectMapper();
Writer strWriter = new StringWriter();
mapper.writeValue(strWriter, objeto);
String stringJSON = strWriter.toString();
wr.writeBytes(stringJSON);
wr.flush();
wr.close();
}
BufferedReader rd = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String line;
StringBuilder stringBuilderJson = new StringBuilder();
while ((line = rd.readLine()) != null) {
stringBuilderJson.append(line);
}
rd.close();
connection.disconnect();
if (classeRetorno == String.class) {
return (T) stringBuilderJson.toString();
} else {
ObjectMapper objectMapper = new ObjectMapper();
return objectMapper.readValue(stringBuilderJson.toString(), classeRetorno);
}
}
enum MetodoType {
GET, POST
}
}
*/
© 2015 - 2025 Weber Informatics LLC | Privacy Policy