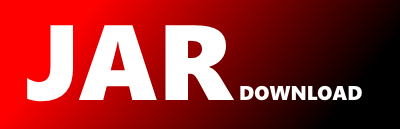
com.arch.util.XmlUtils Maven / Gradle / Ivy
package com.arch.util;
import org.apache.xml.serialize.OutputFormat;
import org.apache.xml.serialize.XMLSerializer;
import org.w3c.dom.Document;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import java.io.IOException;
import java.io.StringReader;
import java.io.StringWriter;
import java.io.Writer;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public final class XmlUtils {
public static final int LINE_WIDTH = 65;
public static final int INDENT = 2;
private XmlUtils() {
}
public static String getValue(String xml, String atributo) {
if (xml == null) {
return "";
}
Pattern regexValue = Pattern.compile("<" + atributo + ">([^<]+)" + atributo + ">");
Matcher match = regexValue.matcher(xml);
if (match.find()) {
return match.group(1);
} else {
return "";
}
}
public static String format(String unformattedXml) throws IOException, ParserConfigurationException, SAXException {
final Document document = parseXmlFile(unformattedXml);
OutputFormat format = new OutputFormat(document);
format.setLineWidth(LINE_WIDTH);
format.setIndenting(true);
format.setIndent(INDENT);
Writer out = new StringWriter();
XMLSerializer serializer = new XMLSerializer(out, format);
serializer.serialize(document);
return out.toString();
}
private static Document parseXmlFile(String in) throws ParserConfigurationException, IOException, SAXException {
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = dbf.newDocumentBuilder();
InputSource is = new InputSource(new StringReader(in));
return db.parse(is);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy