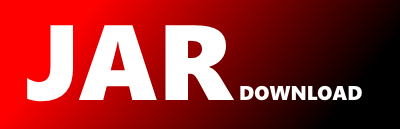
com.arch.util.br.CpfCnpjUtils Maven / Gradle / Ivy
package com.arch.util.br;
import com.arch.util.CaracterUtils;
import java.util.regex.Pattern;
public final class CpfCnpjUtils {
private static final int DOIS = 2;
private static final int TRES = 3;
private static final int QUATRO = 4;
private static final int CINCO = 5;
private static final int SEIS = 6;
private static final int SETE = 7;
private static final int OITO = 8;
private static final int NOVE = 9;
private static final int DEZ = 10;
private static final int ONZE = 11;
private static final int DOZE = 12;
private static final int TREZE = 13;
private static final int QUATORZE = 14;
private static final int QUARENTA_OITO = 48;
public static final int UM = 1;
public static final int ZERO = 0;
private CpfCnpjUtils() {
//
}
public static String formataCpf(String cpf) {
String value = CaracterUtils.alinharTextoDireita(cpf, ONZE, "0");
return formataMascara(value, "###.###.###-##");
}
public static String formataCnpj(String cnpj) {
String value = CaracterUtils.alinharTextoDireita(cnpj, QUATORZE, "0");
return formataMascara(value, "##.###.###/####-##");
}
public static String formata(String valor) {
if (valor.length() <= ONZE) {
return formataCpf(valor);
} else {
return formataCnpj(valor);
}
}
public static boolean valida(String cpfCnpj) {
String value = tiraFormatacao(cpfCnpj);
if (value.length() == ONZE) {
return validaCpf(value);
} else {
return validaCnpj(value);
}
}
public static String tiraFormatacao(String aDesformatar) {
return aDesformatar.replaceAll("[-\\./]", "");
}
public static boolean validaCpf(String cpf) {
String value = cpf.replace(".", "").replace("-", "");
if (validaTamanhoRepeticao(value)) {
return false;
}
int i;
int num;
int peso;
int sm = ZERO;
peso = DEZ;
for (i = ZERO; i < NOVE; i++) {
// converte o i-esimo caractere do value em um numero:
// por exemplo, transforma o caractere '0' no inteiro 0
// (48 eh a posicao de '0' na tabela ASCII)
num = value.charAt(i) - QUARENTA_OITO;
sm = sm + (num * peso);
peso = peso - UM;
}
int r = ONZE - (sm % ONZE);
char dig10;
if ((r == DEZ) || (r == ONZE)) {
dig10 = '0';
} else {
dig10 = (char) (r + QUARENTA_OITO);
}
// converte no respectivo caractere numerico
// Calculo do 2o. Digito Verificador
sm = ZERO;
peso = ONZE;
for (i = ZERO; i < DEZ; i++) {
num = value.charAt(i) - QUARENTA_OITO;
sm = sm + (num * peso);
peso = peso - UM;
}
r = ONZE - (sm % ONZE);
char dig11;
if ((r == DEZ) || (r == ONZE)) {
dig11 = '0';
} else {
dig11 = (char) (r + QUARENTA_OITO);
}
// Verifica se os digitos calculados conferem com os digitos
// informados.
return dig10 == value.charAt(NOVE) && dig11 == value.charAt(DEZ);
}
public static String formataCpfCnpj(String numero) {
if (numero == null) {
return "";
}
StringBuilder cpfCNPJ = new StringBuilder();
String retorno;
if (numero.length() <= ONZE) {
for (int i = ZERO; i < (ONZE - numero.length()); i++) {
cpfCNPJ.append("0");
}
cpfCNPJ.append(numero);
retorno = cpfCNPJ.substring(ZERO, TRES) + "." + cpfCNPJ.substring(TRES, SEIS) + "." + cpfCNPJ.substring(SEIS, NOVE) + "-" + cpfCNPJ.substring(NOVE, ONZE);
} else {
for (int i = ZERO; i < (QUATORZE - numero.length()); i++) {
cpfCNPJ.append("0");
}
cpfCNPJ.append(numero);
retorno = cpfCNPJ.substring(ZERO, DOIS) + "." + cpfCNPJ.substring(DOIS, CINCO) + "." + cpfCNPJ.substring(CINCO, OITO) + "/" + cpfCNPJ.substring(OITO, DOZE) + "-"
+ cpfCNPJ.substring(DOZE, QUATORZE);
}
return retorno;
}
public static String formataCnpjBase(String numero) {
if (numero == null) {
return "";
}
StringBuilder cpfCNPJ = new StringBuilder();
String retorno;
for (int i = ZERO; i < (OITO - numero.length()); i++) {
cpfCNPJ.append("0");
}
cpfCNPJ.append(numero);
retorno = cpfCNPJ.substring(ZERO, DOIS) + "." + cpfCNPJ.substring(DOIS, CINCO) + "." + cpfCNPJ.substring(CINCO, OITO);
return retorno;
}
private static int parseCharToInt(char c) {
return Integer.parseInt(Character.toString(c));
}
private static String formataMascara(String valor, String mascara) {
String dado = "";
// remove caracteres nao numericos
for (int i = ZERO; i < valor.length(); i++) {
char c = valor.charAt(i);
if (Character.isDigit(c)) {
dado += Character.toString(c);
}
}
int indMascara = mascara.length();
int indCampo = dado.length();
for (; indCampo > ZERO && indMascara > ZERO; ) {
indMascara--;
if (mascara.charAt(indMascara) == '#') {
indCampo--;
}
}
String saida = "";
for (; indMascara < mascara.length(); indMascara++) {
if (mascara.charAt(indMascara) == '#') {
saida += dado.charAt(indCampo);
indCampo++;
} else {
saida += mascara.charAt(indMascara);
}
}
return saida;
}
private static boolean validaTamanhoRepeticao(String value) {
for (int i = ZERO; i < DEZ; i++) {
if (CaracterUtils.replicate(Integer.toString(i), ONZE).equals(value)) {
return true;
}
}
return value.length() != ONZE;
}
public static boolean validaCnpj(String numeroCnpj) {
String cnpj = numeroCnpj;
cnpj = cnpj.replaceAll(Pattern.compile("\\s").toString(), "");
cnpj = cnpj.replaceAll(Pattern.compile("\\D").toString(), "");
if (cnpj.length() != QUATORZE) {
return false;
}
char[] aCnpj = cnpj.toCharArray();
int soma = parseCharToInt(aCnpj[ZERO]) * CINCO;
soma += (parseCharToInt(aCnpj[UM]) * QUATRO);
soma += (parseCharToInt(aCnpj[DOIS]) * TRES);
soma += (parseCharToInt(aCnpj[TRES]) * DOIS);
soma += (parseCharToInt(aCnpj[QUATRO]) * NOVE);
soma += (parseCharToInt(aCnpj[CINCO]) * OITO);
soma += (parseCharToInt(aCnpj[SEIS]) * SETE);
soma += (parseCharToInt(aCnpj[SETE]) * SEIS);
soma += (parseCharToInt(aCnpj[OITO]) * CINCO);
soma += (parseCharToInt(aCnpj[NOVE]) * QUATRO);
soma += (parseCharToInt(aCnpj[DEZ]) * TRES);
soma += (parseCharToInt(aCnpj[ONZE]) * DOIS);
int d1 = soma % ONZE;
d1 = d1 < DOIS ? ZERO : (ONZE - d1);
soma = ZERO;
soma += (parseCharToInt(aCnpj[ZERO]) * SEIS);
soma += (parseCharToInt(aCnpj[UM]) * CINCO);
soma += (parseCharToInt(aCnpj[DOIS]) * QUATRO);
soma += (parseCharToInt(aCnpj[TRES]) * TRES);
soma += (parseCharToInt(aCnpj[QUATRO]) * DOIS);
soma += (parseCharToInt(aCnpj[CINCO]) * NOVE);
soma += (parseCharToInt(aCnpj[SEIS]) * OITO);
soma += (parseCharToInt(aCnpj[SETE]) * SETE);
soma += (parseCharToInt(aCnpj[OITO]) * SEIS);
soma += (parseCharToInt(aCnpj[NOVE]) * CINCO);
soma += (parseCharToInt(aCnpj[DEZ]) * QUATRO);
soma += (parseCharToInt(aCnpj[ONZE]) * TRES);
soma += (parseCharToInt(aCnpj[DOZE]) * DOIS);
int d2 = soma % ONZE;
d2 = d2 < DOIS ? ZERO : (ONZE - d2);
return parseCharToInt(aCnpj[DOZE]) == d1 && parseCharToInt(aCnpj[TREZE]) == d2;
}
public static boolean isValidAndNotRequired(String cpfCnpj) {
if (cpfCnpj == null
|| cpfCnpj.isEmpty()) {
return true;
}
return CpfCnpjUtils.valida(cpfCnpj);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy