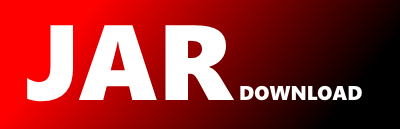
br.com.objectos.code.Configuration Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2016 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.code;
import java.lang.annotation.Annotation;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import javax.annotation.processing.ProcessingEnvironment;
import javax.lang.model.SourceVersion;
import br.com.objectos.core.util.ImmutableList;
import br.com.objectos.core.util.ImmutableSet;
/**
* @author [email protected] (Marcio Endo)
*/
public class Configuration {
private final Set annotationTypeSet;
private final List artifactGeneratorList;
private final ProcessorListener listener;
private final SourceVersion sourceVersion;
private Configuration(Set annotationTypeSet,
List artifactGeneratorList,
ProcessorListener listener,
SourceVersion sourceVersion) {
this.annotationTypeSet = annotationTypeSet;
this.artifactGeneratorList = artifactGeneratorList;
this.listener = listener;
this.sourceVersion = sourceVersion;
}
public static ConfigurationBuilder builder() {
return new Builder();
}
public AnnotationExecutor newExecutor(ProcessingEnvironment processingEnv) {
ProcessingEnvironmentWrapper wrapper = listener.wrap(processingEnv);
return new AnnotationExecutor(wrapper, artifactGeneratorList, listener);
}
Set annotationTypeSet() {
return annotationTypeSet;
}
List artifactGeneratorList() {
return artifactGeneratorList;
}
ProcessorListener listener() {
return listener;
}
SourceVersion sourceVersion() {
return sourceVersion;
}
private static class Builder
implements
ConfigurationBuilder,
ConfigurationBuilder.AddArtifactGenerator,
ConfigurationBuilder.AddAnnotationType,
ConfigurationBuilder.SetListener,
ConfigurationBuilder.SupportedSourceVersion {
private final ImmutableSet.Builder annotationTypeSet = ImmutableSet.builder();
private final ImmutableList.Builder artifactGeneratorList = ImmutableList.builder();
private ProcessorListener listener = EmptyProcessorListener.INSTANCE;
private SourceVersion sourceVersion = SourceVersion.latestSupported();
@Override
public AddAnnotationType addAllAnnotationTypesByName(Iterable qualifiedNames) {
annotationTypeSet.addAll(qualifiedNames);
return this;
}
@Override
public AddAnnotationType addAllAnnotationTypesByType(Iterable> annotationTypes) {
for (Class extends Annotation> annotationType : annotationTypes) {
addAnnotationType(annotationType);
}
return this;
}
@Override
public AddAnnotationType addAnnotationType(Class extends Annotation> annotationType) {
Objects.requireNonNull(annotationType);
annotationTypeSet.add(annotationType.getName());
return this;
}
@Override
public AddAnnotationType addAnnotationType(String qualifiedName) {
Objects.requireNonNull(qualifiedName);
annotationTypeSet.add(qualifiedName);
return this;
}
@Override
public AddArtifactGenerator addMethodInfoArtifactGenerator(MethodInfoArtifactGenerator generator) {
return addArtifactGenerator(generator);
}
@Override
public AddArtifactGenerator addPackageInfoArtifactGenerator(PackageInfoArtifactGenerator generator) {
return addArtifactGenerator(generator);
}
@Override
public AddArtifactGenerator addTypeInfoArtifactGenerator(TypeInfoArtifactGenerator generator) {
return addArtifactGenerator(generator);
}
@Override
public SetListener listener(ProcessorListener listener) {
Objects.requireNonNull(listener);
this.listener = listener;
return this;
}
@Override
public Configuration build() {
return new Configuration(
annotationTypeSet.build(),
artifactGeneratorList.build(),
listener,
sourceVersion);
}
@Override
public SupportedSourceVersion supportedSourceVersion(SourceVersion version) {
Objects.requireNonNull(version);
sourceVersion = version;
return this;
}
private AddArtifactGenerator addArtifactGenerator(ArtifactGenerator generator) {
Objects.requireNonNull(generator);
artifactGeneratorList.add(generator);
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy