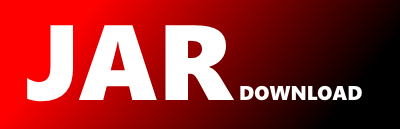
br.com.objectos.way.io.TableHeader Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2013 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.io;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Function;
import br.com.objectos.core.collections.MoreCollectors;
/**
* @author marcio.endo@objectos.com.br (Marcio Endo)
*/
class TableHeader {
final int maxSize;
final List columns;
private TableHeader(int maxSize, List columns) {
this.maxSize = maxSize;
this.columns = columns;
}
public static TableHeader headerOf(List cols) {
ToHeader func = new ToHeader();
List columns = cols.stream()
.map(func)
.collect(MoreCollectors.toImmutableList());
return new TableHeader(func.maxSize, columns);
}
public void apachePOI(POISheet sheet) {
List rows = new ArrayList<>(maxSize);
for (int i = 0; i < maxSize; i++) {
POIRow row = sheet.createRow(i);
rows.add(row);
}
for (TableColumnHeader header : columns) {
header.apachePOI(rows);
}
}
private static class ToHeader implements Function {
int maxSize = 0;
@Override
public TableColumnHeader apply(TableColumn input) {
TableColumnHeader header = input.getHeader();
maxSize = Math.max(maxSize, header.size());
return header;
}
}
}