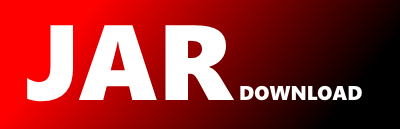
br.com.objectos.flat.RecordWriter Maven / Gradle / Ivy
/*
* Copyright 2015 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.flat;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
/**
* @author [email protected] (Marcio Endo)
*/
public class RecordWriter {
private final List fieldList = new ArrayList<>();
private final Appendable out;
RecordWriter(Appendable out) {
this.out = out;
}
@Override
public String toString() {
return out.toString();
}
public RecordWriter custom(T value, int length, CustomFormatter formatter) {
fieldList.add(CustomField.get(value, length, formatter));
return this;
}
public RecordWriter decimal(double value, int precision, int scale, DecimalOption... options) {
fieldList.add(DoubleField.get(value, precision, scale, options));
return this;
}
public RecordWriter fixed(String text) {
fieldList.add(FixedField.get(text));
return this;
}
public RecordWriter flatEnum(Enum> value, int length) {
fieldList.add(FlatEnumField.get(value, length));
return this;
}
public RecordWriter integer(int value, int length, IntegerOption... options) {
fieldList.add(IntegerField.get(value, length, options));
return this;
}
public RecordWriter localDate(LocalDate date, LocalDatePattern pattern) {
fieldList.add(LocalDateField.get(date, pattern));
return this;
}
public LocalDateWriter localDate(Optional maybe, LocalDatePattern pattern) {
return new LocalDateWriter(maybe, pattern);
}
public RecordWriter localDateTime(LocalDateTime dateTime, LocalDateTimePattern pattern) {
fieldList.add(LocalDateTimeField.get(dateTime, pattern));
return this;
}
public LocalDateTimeWriter localDateTime(Optional maybe, LocalDateTimePattern pattern) {
return new LocalDateTimeWriter(maybe, pattern);
}
public RecordWriter text(String text, int length, TextOption... options) {
fieldList.add(TextField.get(text, length, options));
return this;
}
public DoubleValueConditionWriter when(DoubleValueCondition condition, String text) {
return new DoubleValueConditionWriter(condition, text);
}
public IntValueConditionWriter when(IntValueCondition condition, String text) {
return new IntValueConditionWriter(condition, text);
}
public RecordWriter write() {
fieldList.add(NewLineField.get());
for (Field field : fieldList) {
field.writeTo(out);
}
return this;
}
public class DoubleValueConditionWriter {
private final DoubleValueCondition condition;
private final String text;
public DoubleValueConditionWriter(DoubleValueCondition condition, String text) {
this.condition = condition;
this.text = text;
}
public RecordWriter decimal(double value, int precision, int scale, DecimalOption... options) {
fieldList.add(DoubleField.get(value, precision, scale, condition, text, options));
return RecordWriter.this;
}
}
public class IntValueConditionWriter {
private final IntValueCondition condition;
private final String text;
public IntValueConditionWriter(IntValueCondition condition, String text) {
this.condition = condition;
this.text = text;
}
public RecordWriter integer(int value, int length, IntegerOption... options) {
fieldList.add(IntegerField.get(value, length, condition, text, options));
return RecordWriter.this;
}
}
public class LocalDateWriter {
private final Optional maybe;
private final LocalDatePattern pattern;
private LocalDateWriter(Optional maybe, LocalDatePattern pattern) {
this.maybe = maybe;
this.pattern = pattern;
}
public RecordWriter or(String defaultValue) {
fieldList.add(LocalDateField.get(maybe, pattern, defaultValue));
return RecordWriter.this;
}
}
public class LocalDateTimeWriter {
private final Optional maybe;
private final LocalDateTimePattern pattern;
private LocalDateTimeWriter(Optional maybe, LocalDateTimePattern pattern) {
this.maybe = maybe;
this.pattern = pattern;
}
public RecordWriter or(String defaultValue) {
fieldList.add(LocalDateTimeField.get(maybe, pattern, defaultValue));
return RecordWriter.this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy