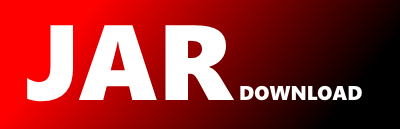
br.com.objectos.flat.FlatWriter Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2016 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.flat;
import static br.com.objectos.flat.TextTransformation.LEFT_ALIGN;
import static br.com.objectos.flat.TextTransformation.TRUNCATE;
import java.math.BigDecimal;
import java.math.RoundingMode;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.util.Arrays;
import java.util.EnumSet;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import br.com.objectos.core.lang.Preconditions;
import br.com.objectos.flat.DecimalOption;
import br.com.objectos.flat.FlatEnum;
import br.com.objectos.flat.IntegerOption;
import br.com.objectos.flat.LocalDatePattern;
import br.com.objectos.flat.LocalDateTimePattern;
import br.com.objectos.flat.TextOption;
/**
* @author [email protected] (Marcio Endo)
*/
public class FlatWriter implements AutoCloseable {
private static final Set ENUM_TRANSFORMS = EnumSet.of(TRUNCATE, LEFT_ALIGN);
private static final String LINE_SEPARATOR = System.getProperty("line.separator");
private final FlatAppendable out;
private FlatWriter(Appendable out) {
this.out = new FlatAppendable(out);
}
public static FlatWriter of(Appendable a) {
Objects.requireNonNull(a);
return new FlatWriter(a);
}
@Override
public void close() {
out.close();
}
public FlatWriter decimal(double value, int precision, int scale, DecimalOption... options) {
return Double.isNaN(value)
? fill(' ', precision)
: decimalStd(value, precision, scale, options);
}
public FlatWriter fill(char character, int length) {
Preconditions.checkArgument(length > 0);
for (int i = 0; i < length; i++) {
out.append(character);
}
return this;
}
public FlatWriter fixed(String fixed) {
out.append(fixed);
return this;
}
public & FlatEnum> FlatWriter flatEnum(E enumValue, int length) {
Objects.requireNonNull(enumValue);
String text = enumValue.flatValue();
for (TextTransformation transformation : ENUM_TRANSFORMS) {
text = transformation.apply(text, length);
}
out.append(text);
return this;
}
public FlatWriter integer(int value, int length, IntegerOption... options) {
StringBuilder format = new StringBuilder();
format.append('%');
for (IntegerOption option : options) {
option.formatString(format);
}
format.append(length);
format.append('d');
out.append(length, format.toString(), value);
return this;
}
public FlatWriter localDate(LocalDate date, LocalDatePattern pattern) {
Objects.requireNonNull(date);
Objects.requireNonNull(pattern);
out.append(pattern.toString(date));
return this;
}
public FlatWriter localDateTime(LocalDateTime dateTime, LocalDateTimePattern pattern) {
Objects.requireNonNull(dateTime);
Objects.requireNonNull(pattern);
out.append(pattern.toString(dateTime));
return this;
}
public FlatWriter text(String text, int length, TextOption... options) {
String value = Objects.requireNonNull(text);
List optionList = Arrays.asList(options);
for (TextTransformation transformation : TextTransformation.values()) {
if (transformation.matches(optionList)) {
value = transformation.apply(value, length);
}
}
out.append(value);
return this;
}
public FlatWriter write() {
out.append(LINE_SEPARATOR);
return this;
}
private FlatWriter decimalStd(double value, int precision, int scale, DecimalOption... options) {
BigDecimal multiplier = new BigDecimal((int) Math.pow(10, scale));
int normalized = new BigDecimal(value).setScale(scale, RoundingMode.HALF_EVEN).multiply(multiplier).intValue();
StringBuilder format = new StringBuilder();
format.append('%');
for (DecimalOption option : options) {
option.formatString(format);
}
format.append(precision);
format.append('d');
out.append(String.format(format.toString(), normalized));
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy