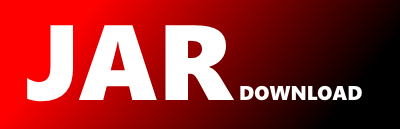
br.com.objectos.http.Channel Maven / Gradle / Ivy
/*
* Copyright 2016 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.http;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.ByteBuffer;
import java.nio.channels.SelectionKey;
import java.nio.channels.Selector;
import java.nio.channels.ServerSocketChannel;
import java.nio.channels.SocketChannel;
import java.util.Iterator;
import java.util.Set;
/**
* @author [email protected] (Marcio Endo)
*/
class Channel implements AutoCloseable {
private final ServerSocketChannel channel;
private final Selector selector;
private final ByteBuffer buffer = ByteBuffer.allocate(8192);
private Channel(ServerSocketChannel channel, Selector selector) {
this.channel = channel;
this.selector = selector;
}
public static Channel get(int port) throws IOException {
ServerSocketChannel channel = ServerSocketChannel.open();
channel.bind(new InetSocketAddress(port));
channel.configureBlocking(false);
Selector selector = Selector.open();
channel.register(selector, SelectionKey.OP_ACCEPT);
return new Channel(channel, selector);
}
public Socket accept() throws IOException {
Socket selected = NoopSocket.instance();
int select = selector.select();
if (select != 0) {
selected = select();
}
return selected;
}
@Override
public void close() throws IOException {
try {
channel.close();
} finally {
selector.close();
}
}
private Socket select() throws IOException {
Socket selected = NoopSocket.instance();
Set selectedKeys = selector.selectedKeys();
Iterator keyIterator = selectedKeys.iterator();
while (keyIterator.hasNext()) {
SelectionKey selectedKey = keyIterator.next();
keyIterator.remove();
if (!selectedKey.isValid()) {
continue;
}
if (selectedKey.isAcceptable()) {
SocketChannel accepted = channel.accept();
selected = HttpSocket.of(accepted, buffer);
break;
}
}
return selected;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy