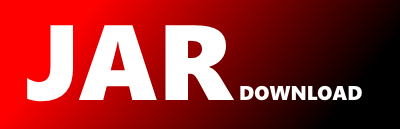
br.com.objectos.lang.AbstractShutdownHook Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of objectos-lang Show documentation
Show all versions of objectos-lang Show documentation
A set of utilities for classes of the java.lang and the java.util packages.
The newest version!
/*
* Copyright (C) 2011-2021 Objectos Software LTDA.
*
* This file is part of the ObjectosComuns project.
*
* ObjectosComuns is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* ObjectosComuns is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with ObjectosComuns. If not, see .
*/
package br.com.objectos.lang;
import static br.com.objectos.lang.Lang.checkNotNull;
import br.com.objectos.annotations.VisibleForTesting;
import br.com.objectos.logging.Event0;
import br.com.objectos.logging.Event1;
import br.com.objectos.logging.Logger;
import br.com.objectos.logging.Logging;
import br.com.objectos.multirelease.Concrete;
import java.util.ArrayList;
import java.util.List;
@Concrete(modifiers = "public", simpleName = "ShutdownHook")
abstract class AbstractShutdownHook {
private static final Event1 CAUGHT_EXCEPTION;
private static final Event1 FINISHED;
private static final Event0 STARTED;
static {
Class> s;
s = ShutdownHook.class;
CAUGHT_EXCEPTION = Logging.warn(s, "CAUGHT_EXCEPTION", Throwable.class);
FINISHED = Logging.info(s, "FINISHED", Long.class);
STARTED = Logging.info(s, "STARTED");
}
private List listeners;
private Logger logger = Logging.getNoopLogger();
private Thread thread;
private List threads;
public final void addListener(ShutdownHookListener listener) {
checkNotNull(listener, "listener == null");
if (listeners == null) {
synchronized (this) {
if (listeners == null) {
listeners = new ArrayList();
}
}
}
synchronized (listeners) {
listeners.add(listener);
}
}
public final void addThread(Thread thread) {
checkNotNull(thread, "thread == null");
if (threads == null) {
synchronized (this) {
if (threads == null) {
threads = new ArrayList();
}
}
}
synchronized (threads) {
threads.add(thread);
}
}
public synchronized final void setLogger(Logger logger) {
this.logger = checkNotNull(logger, "logger == null");
}
final void log(Throwable e) {
logger.log(CAUGHT_EXCEPTION, e);
}
final void register() {
thread = new Job();
thread.setDaemon(true);
Runtime runtime;
runtime = Runtime.getRuntime();
runtime.addShutdownHook(thread);
}
abstract void run0();
@VisibleForTesting
final Thread startAndJoinThread() throws InterruptedException {
thread.start();
thread.join();
return thread;
}
private class Job extends Thread {
Job() {
super("ShutdownHook");
}
@Override
public final void run() {
long startTime;
startTime = System.currentTimeMillis();
logger.log(STARTED);
if (listeners != null) {
doListeners();
}
if (threads != null) {
doThreads();
}
run0();
long totalTime;
totalTime = System.currentTimeMillis() - startTime;
logger.log(FINISHED, totalTime);
}
private void doListeners() {
for (int i = listeners.size() - 1; i >= 0; i--) {
ShutdownHookListener l;
l = listeners.get(i);
try {
l.onShutdownHook();
} catch (Exception e) {
log(e);
}
}
}
private void doThreads() {
for (int i = threads.size() - 1; i >= 0; i--) {
Thread t;
t = threads.get(i);
try {
t.interrupt();
} catch (Exception e) {
log(e);
}
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy