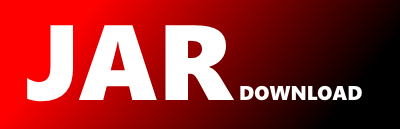
br.com.objectos.lang.Lang Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of objectos-lang Show documentation
Show all versions of objectos-lang Show documentation
A set of utilities for classes of the java.lang and the java.util packages.
The newest version!
/*
* Copyright (C) 2011-2021 Objectos Software LTDA.
*
* This file is part of the ObjectosComuns project.
*
* ObjectosComuns is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* ObjectosComuns is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with ObjectosComuns. If not, see .
*/
package br.com.objectos.lang;
import java.io.Closeable;
import java.io.IOException;
import java.lang.reflect.InvocationTargetException;
import java.nio.charset.Charset;
import java.util.NoSuchElementException;
import java.util.UUID;
import java.util.zip.ZipFile;
public final class Lang {
static final char[] HEX_CHARS = "0123456789abcdef".toCharArray();
private Lang() {}
public static Exception addSuppressed(Exception exception, Exception suppressed) {
return ThrowablesSingleton.INSTANCE.addSuppressed(exception, suppressed);
}
public static char[] append(char[] array, int offset, char[] value) {
return MoreArrays.append(array, offset, value);
}
public static char[] append(char[] array, int offset, String value) {
return MoreArrays.append(array, offset, value);
}
public static void checkArgument(boolean expression) {
Preconditions.checkArgument(expression);
}
public static void checkArgument(boolean expression, String message) {
Preconditions.checkArgument(expression, message);
}
public static void checkArgument(boolean expression, String format, Object... args) {
Preconditions.checkArgument(expression, format, args);
}
public static T checkNotNull(T reference) {
return Preconditions.checkNotNull(reference);
}
public static T checkNotNull(T reference, String message) {
return Preconditions.checkNotNull(reference, message);
}
public static T checkNotNull(T reference, String format, Object... args) {
return Preconditions.checkNotNull(reference, format, args);
}
public static void checkState(boolean state) {
Preconditions.checkState(state);
}
public static void checkState(boolean state, String message) {
Preconditions.checkState(state, message);
}
public static IOException close(IOException rethrow, Closeable closeable) {
return CloserSingleton.INSTANCE.close(rethrow, closeable);
}
public static IOException close(IOException rethrow, ZipFile file) {
return CloserSingleton.INSTANCE.close(rethrow, file);
}
public static StackTraceElement createStackTraceElement(
String classLoaderName,
String moduleName, String moduleVersion,
String declaringClass, String methodName,
String fileName, int lineNumber) {
return ThrowablesSingleton.INSTANCE.createStackTraceElement(
classLoaderName,
moduleName, moduleVersion,
declaringClass, methodName,
fileName, lineNumber
);
}
public static ThreadMode daemonThread() {
return ThreadModeImpl.DAEMON;
}
public static byte[] emptyByteArray() {
return MoreArrays.EMPTY_BYTE_ARRAY;
}
public static char[] emptyCharArray() {
return MoreArrays.EMPTY_CHAR_ARRAY;
}
public static int[] emptyIntArray() {
return MoreArrays.EMPTY_INT_ARRAY;
}
public static Object[] emptyObjectArray() {
return MoreArrays.EMPTY_OBJECT_ARRAY;
}
public static boolean equals(Object first, Object second) {
return MoreObjects.equals(first, second);
}
public static String format(long bytes, ByteUnit unit) {
return unit.format(bytes);
}
public static void formatToString(
StringBuilder sb, int depth, Object source) {
ToString.formatToString(
sb, depth, source
);
}
public static void formatToString(
StringBuilder sb, int depth, Object source,
String name, Object value) {
ToString.formatToString(
sb, depth, source,
name, value
);
}
public static void formatToString(
StringBuilder sb, int depth, Object source,
String name1, Object value1,
String name2, Object value2) {
ToString.formatToString(
sb, depth, source,
name1, value1,
name2, value2
);
}
public static void formatToString(
StringBuilder sb, int depth, Object source,
String name1, Object value1,
String name2, Object value2,
String name3, Object value3) {
ToString.formatToString(
sb, depth, source,
name1, value1,
name2, value2,
name3, value3
);
}
public static void formatToString(
StringBuilder sb, int depth, Object source,
String name1, Object value1,
String name2, Object value2,
String name3, Object value3,
String name4, Object value4) {
ToString.formatToString(
sb, depth, source,
name1, value1,
name2, value2,
name3, value3,
name4, value4
);
}
public static void formatToString(
StringBuilder sb, int depth, Object source,
String name1, Object value1,
String name2, Object value2,
String name3, Object value3,
String name4, Object value4,
String name5, Object value5) {
ToString.formatToString(
sb, depth, source,
name1, value1,
name2, value2,
name3, value3,
name4, value4,
name5, value5
);
}
public static void formatToStringList(
StringBuilder sb, int depth, Object source, ToStringList list) {
ToString.formatToStringList(
sb, depth, source, list
);
}
public static void formatToStringMap(
StringBuilder sb, int depth, Object source, ToStringMap map) {
ToString.formatToStringMap(
sb, depth, source, map
);
}
public static String getClassLoaderName(StackTraceElement element) {
return ThrowablesSingleton.INSTANCE.getClassLoaderName(element);
}
public static JavaProfile getJavaProfile() {
return JavaProfileProviderSingleton.INSTANCE.get();
}
public static String getModuleName(StackTraceElement element) {
return ThrowablesSingleton.INSTANCE.getModuleName(element);
}
public static String getModuleVersion(StackTraceElement element) {
return ThrowablesSingleton.INSTANCE.getModuleVersion(element);
}
public static OperatingSystem getOperatingSystem() {
return OperatingSystem.getInstance();
}
public static ShutdownHook getShutdownHook() {
return ShutdownHookLazy.INSTANCE;
}
public static String getStackTraceAsString(Throwable throwable) {
return Throwables.getStackTraceAsString(throwable);
}
public static Throwable[] getSuppressed(Throwable throwable) {
return ThrowablesSingleton.INSTANCE.getSuppressed(throwable);
}
public static String getSystemProperty(String propertyName) {
String property;
property = System.getProperty(propertyName);
if (property == null) {
throw new NoSuchElementException(propertyName);
}
return property;
}
public static int growArrayLength(int currentLength, int requiredIndex) {
return MoreArrays.growArrayLength(currentLength, requiredIndex);
}
public static byte[] growIfNecessary(byte[] array, int requiredIndex) {
return MoreArrays.growIfNecessary(array, requiredIndex);
}
public static char[] growIfNecessary(char[] array, int requiredIndex) {
return MoreArrays.growIfNecessary(array, requiredIndex);
}
public static double[] growIfNecessary(double[] array, int requiredIndex) {
return MoreArrays.growIfNecessary(array, requiredIndex);
}
public static E[] growIfNecessary(E[] array, int requiredIndex) {
return MoreArrays.growIfNecessary(array, requiredIndex);
}
public static int[] growIfNecessary(int[] array, int requiredIndex) {
return MoreArrays.growIfNecessary(array, requiredIndex);
}
public static int[][] growIfNecessary(int[][] array, int requiredIndex) {
return MoreArrays.growIfNecessary(array, requiredIndex);
}
public static int hashCode(int hc1, int hc2) {
return MoreObjects.hashCode(hc1, hc2);
}
public static int hashCode(int hc1, int hc2, int hc3) {
return MoreObjects.hashCode(hc1, hc2, hc3);
}
public static int hashCode(int hc1, int hc2, int hc3, int hc4) {
return MoreObjects.hashCode(hc1, hc2, hc3, hc4);
}
public static int hashCode(int hc1, int hc2, int hc3, int hc4, int hc5) {
return MoreObjects.hashCode(hc1, hc2, hc3, hc4, hc5);
}
public static int hashCode(int hc1, int hc2, int hc3, int hc4, int hc5, int... rest) {
return MoreObjects.hashCode(hc1, hc2, hc3, hc4, hc5, rest);
}
public static int hashCode(Object o) {
return MoreObjects.hashCode(o);
}
public static int hashCode(Object o1, Object o2) {
return MoreObjects.hashCode(o1, o2);
}
public static int hashCode(Object o1, Object o2, Object o3) {
return MoreObjects.hashCode(o1, o2, o3);
}
public static int hashCode(Object o1, Object o2, Object o3, Object o4) {
return MoreObjects.hashCode(o1, o2, o3, o4);
}
public static int hashCode(Object o1, Object o2, Object o3, Object o4, Object o5) {
return MoreObjects.hashCode(o1, o2, o3, o4, o5);
}
public static int hashCode(
Object o1, Object o2, Object o3, Object o4, Object o5, Object... rest) {
return MoreObjects.hashCode(o1, o2, o3, o4, o5, rest);
}
public static int hashCodeStart() {
return MoreObjects.HASH_CODE_START;
}
public static int hashCodeUpdate(int partial, int hashCode) {
return MoreObjects.hashCode0(partial, hashCode);
}
public static int hashCodeUpdate(int partial, Object o) {
return MoreObjects.hashCode0(partial, o);
}
public static boolean isDigit(byte utf8) {
return Bytes.isDigit(utf8);
}
public static boolean isHexDigit(char c) {
return Characters.isHexDigit(c);
}
public static boolean isNullOrEmpty(String string) {
return Strings.isNullOrEmpty(string);
}
public static Charset isoLatin1() {
return CharsetIsoLatin1.INSTANCE;
}
public static String lineSeparator() {
return StandardSystemProperties.LINE_SEPARATOR;
}
public static T newInstance(Class type)
throws IllegalAccessException,
InvocationTargetException,
InstantiationException,
NoSuchMethodException,
SecurityException {
checkNotNull(type, "type == null");
return ClassesSingleton.INSTANCE.newInstance(type);
}
public static String nullToEmpty(String string) {
return Strings.nullToEmpty(string);
}
public static int parseInt(byte utf8) {
return Ints.parseInt(utf8);
}
public static int parseInt(byte[] utf8) {
return parseInt(utf8, 0, utf8.length);
}
public static int parseInt(byte[] utf8, int offset, int length) {
return Ints.parseInt(utf8, offset, length);
}
public static int parseInt(char c) {
return Ints.parseInt(c);
}
public static byte[] randomByteArray(int length) {
return RandomImpl.INSTANCE.randomByteArray(length);
}
public static void randomBytes(byte[] bytes) {
RandomImpl.INSTANCE.randomBytes(bytes);
}
public static double randomDouble() {
return RandomImpl.INSTANCE.randomDouble();
}
public static int randomInt() {
return RandomImpl.INSTANCE.randomInt();
}
public static int randomInt(int bound) {
return RandomImpl.INSTANCE.randomInt(bound);
}
public static String randomString(int length) {
return RandomImpl.INSTANCE.randomString(length);
}
public static void rethrowIfNecessary(E rethrow) throws E {
if (rethrow != null) {
throw rethrow;
}
}
public static void startServices(Service... services) throws Exception {
checkNotNull(services, "services == null");
ShutdownHook shutdownHook;
shutdownHook = getShutdownHook();
for (int i = 0, length = services.length; i < length; i++) {
Service s;
s = services[i];
if (s == null) {
throw new NullPointerException("services[" + i + "] == null");
}
shutdownHook.addListener(s);
s.startService();
}
}
public static String stripDiacritics(String string) {
checkNotNull(string, "string == null");
return Strings.stripDiacritics(string);
}
public static byte[] toByteArray(UUID uuid) {
return UUIDs.toByteArray(uuid);
}
public static String toCamelCase(String string) {
checkNotNull(string, "string == null");
return Strings.toCamelCase(string);
}
public static int[] toCodePoints(String string) {
checkNotNull(string, "string == null");
return Strings.toCodePoints(string);
}
public static char toHexChar(int digit) {
return HEX_CHARS[digit];
}
public static String toHexString(byte[] bytes) {
checkNotNull(bytes, "bytes == null");
int length;
length = bytes.length;
switch (length) {
case 0:
return "";
default:
return MoreArrays.toHexStringNonEmpty(bytes, 0, length);
}
}
public static String toHexString(byte[] bytes, int offset, int length) {
checkNotNull(bytes, "bytes == null");
int requiredLength;
requiredLength = offset + length;
checkArgument(
requiredLength <= bytes.length,
"requiredLength exceeds array length: %d > %d", requiredLength, length
);
switch (length) {
case 0:
return "";
default:
return MoreArrays.toHexStringNonEmpty(bytes, offset, length);
}
}
public static String toHexString(UUID uuid) {
return UUIDs.toHexString(uuid);
}
public static String toJavaIdentifier(String string) {
return ToJava.TO_JAVA_IDENTIFIER.apply(string);
}
public static String toJavaLowerName(String string) {
return ToJava.TO_JAVA_VARIABLE_NAME.apply(string);
}
public static String toJavaUpperName(String string) {
return ToJava.TO_JAVA_UPPER_NAME.apply(string);
}
public static String toString(int value) {
return Integer.toString(value);
}
public static String toString(
Object source) {
return ToString.toString(
source
);
}
public static String toString(
Object source,
String name, Object value) {
return ToString.toString(
source,
name, value
);
}
public static String toString(
Object source,
String name1, Object value1,
String name2, Object value2) {
return ToString.toString(
source,
name1, value1,
name2, value2
);
}
public static String toString(
Object source,
String name1, Object value1,
String name2, Object value2,
String name3, Object value3) {
return ToString.toString(
source,
name1, value1,
name2, value2,
name3, value3
);
}
public static String toString(
Object source,
String name1, Object value1,
String name2, Object value2,
String name3, Object value3,
String name4, Object value4) {
return ToString.toString(
source,
name1, value1,
name2, value2,
name3, value3,
name4, value4
);
}
public static String toString(
Object source,
String name1, Object value1,
String name2, Object value2,
String name3, Object value3,
String name4, Object value4,
String name5, Object value5) {
return ToString.toString(
source,
name1, value1,
name2, value2,
name3, value3,
name4, value4,
name5, value5
);
}
public static String toString(
Object source,
String name1, Object value1,
String name2, Object value2,
String name3, Object value3,
String name4, Object value4,
String name5, Object value5,
String name6, Object value6) {
return ToString.toString(
source,
name1, value1,
name2, value2,
name3, value3,
name4, value4,
name5, value5,
name6, value6
);
}
public static String toString(
ToStringObject object) {
return ToString.toString(
object
);
}
public static Charset usAscii() {
return CharsetUsAscii.INSTANCE;
}
public static ThreadMode userThread() {
return ThreadModeImpl.USER;
}
public static Charset utf8() {
return CharsetUtf8.INSTANCE;
}
public final static class UsAsciiBytes {
public static final byte DIGIT_0 = 0x30;
public static final byte DIGIT_1 = 0x31;
public static final byte DIGIT_2 = 0x32;
public static final byte DIGIT_3 = 0x33;
public static final byte DIGIT_4 = 0x34;
public static final byte DIGIT_5 = 0x35;
public static final byte DIGIT_6 = 0x36;
public static final byte DIGIT_7 = 0x37;
public static final byte DIGIT_8 = 0x38;
public static final byte DIGIT_9 = 0x39;
private UsAsciiBytes() {}
}
public final static class Utf8Bytes {
public static final byte DIGIT_0 = UsAsciiBytes.DIGIT_0;
public static final byte DIGIT_1 = UsAsciiBytes.DIGIT_1;
public static final byte DIGIT_2 = UsAsciiBytes.DIGIT_2;
public static final byte DIGIT_3 = UsAsciiBytes.DIGIT_3;
public static final byte DIGIT_4 = UsAsciiBytes.DIGIT_4;
public static final byte DIGIT_5 = UsAsciiBytes.DIGIT_5;
public static final byte DIGIT_6 = UsAsciiBytes.DIGIT_6;
public static final byte DIGIT_7 = UsAsciiBytes.DIGIT_7;
public static final byte DIGIT_8 = UsAsciiBytes.DIGIT_8;
public static final byte DIGIT_9 = UsAsciiBytes.DIGIT_9;
private Utf8Bytes() {}
}
private static class CharsetIsoLatin1 {
static Charset INSTANCE = Charset.forName("ISO-8859-1");
}
private static class CharsetUsAscii {
static Charset INSTANCE = Charset.forName("US-ASCII");
}
private static class CharsetUtf8 {
static Charset INSTANCE = Charset.forName("UTF-8");
}
private static class ShutdownHookLazy {
static ShutdownHook INSTANCE = create();
private static ShutdownHook create() {
ShutdownHook shutdownHook;
shutdownHook = new ShutdownHook();
shutdownHook.register();
return shutdownHook;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy