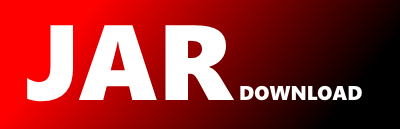
br.com.objectos.lang.RandomImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of objectos-lang Show documentation
Show all versions of objectos-lang Show documentation
A set of utilities for classes of the java.lang and the java.util packages.
The newest version!
/*
* Copyright (C) 2011-2021 Objectos Software LTDA.
*
* This file is part of the ObjectosComuns project.
*
* ObjectosComuns is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* ObjectosComuns is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with ObjectosComuns. If not, see .
*/
package br.com.objectos.lang;
import static br.com.objectos.lang.Lang.checkArgument;
import static br.com.objectos.lang.Lang.checkNotNull;
import java.security.SecureRandom;
import java.util.Random;
final class RandomImpl {
static final String DEFAULT_DICTIONARY
= "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz";
static final RandomImpl INSTANCE = new RandomImpl();
private static final char[] DEFAULT_DICTIONARY_CHARS = DEFAULT_DICTIONARY.toCharArray();
private final Random delegate = new SecureRandom();
private RandomImpl() {}
public final byte[] randomByteArray(int length) {
checkArgument(length >= 0, "length has to be >= 0");
switch (length) {
case 0:
return Lang.emptyByteArray();
default:
byte[] array;
array = new byte[length];
randomBytes(array);
return array;
}
}
public final void randomBytes(byte[] bytes) {
checkNotNull(bytes, "bytes == null");
delegate.nextBytes(bytes);
}
public final double randomDouble() {
return delegate.nextDouble();
}
public final int randomInt() {
return delegate.nextInt();
}
public final int randomInt(int bound) {
return delegate.nextInt(bound);
}
public final String randomString(int length) {
checkArgument(length > 0, "length has to be > 0");
return randomString0(DEFAULT_DICTIONARY_CHARS, length);
}
private String randomString0(char[] chars, int length) {
char[] result;
result = new char[length];
int index;
index = 0;
int fullIntCount;
fullIntCount = length / 4;
for (int i = 0; i < fullIntCount; i++) {
int value;
value = delegate.nextInt();
result[index++] = chars[((value >> 12) & 0xFF) % chars.length];
result[index++] = chars[((value >> 8) & 0xFF) % chars.length];
result[index++] = chars[((value >> 4) & 0xFF) % chars.length];
result[index++] = chars[(value & 0xFF) % chars.length];
}
int lastIntCount;
lastIntCount = length % 4;
if (lastIntCount > 0) {
int value;
value = delegate.nextInt();
switch (lastIntCount) {
case 3:
result[index + 2] = chars[((value >> 4) & 0xFF) % chars.length];
// fall through
case 2:
result[index + 1] = chars[((value >> 8) & 0xFF) % chars.length];
// fall through
case 1:
result[index + 0] = chars[((value >> 12) & 0xFF) % chars.length];
break;
default:
throw new AssertionError("Should not happen");
}
}
return new String(result);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy