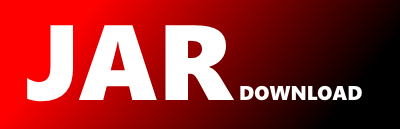
br.com.objectos.lang.ByteUnit Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of objectos-lang Show documentation
Show all versions of objectos-lang Show documentation
A set of utilities for classes of the java.lang and the java.util packages.
The newest version!
/*
* Copyright (C) 2011-2021 Objectos Software LTDA.
*
* This file is part of the ObjectosComuns project.
*
* ObjectosComuns is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* ObjectosComuns is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with ObjectosComuns. If not, see .
*/
package br.com.objectos.lang;
import static br.com.objectos.lang.Lang.checkArgument;
public final class ByteUnit {
public static final ByteUnit BINARY = new ByteUnit(
1024L, "KiB", "MiB", "GiB", "TiB", "PiB", "EiB"
);
public static final ByteUnit DECIMAL = new ByteUnit(
1000L, "kB", "MB", "GB", "TB", "PB", "EB"
);
private static final char DECIMAL_SEPARATOR = '.';
private final long kilo;
private final long mega;
private final long giga;
private final long tera;
private final long peta;
private final long exa;
private final String[] units;
private ByteUnit(long kilo, String... units) {
this.kilo = kilo;
mega = kilo * kilo;
giga = mega * kilo;
tera = giga * kilo;
peta = tera * kilo;
exa = peta * kilo;
this.units = units;
}
final String format(long bytes) {
checkArgument(bytes >= 0, "bytes must be >= 0");
StringBuilder result;
result = new StringBuilder();
if (bytes < kilo) {
result.append(bytes);
result.append(' ');
result.append('B');
return result.toString();
}
if (bytes < mega) {
return format0(result, bytes, kilo, units[0]);
}
if (bytes < giga) {
return format0(result, bytes, mega, units[1]);
}
if (bytes < tera) {
return format0(result, bytes, giga, units[2]);
}
if (bytes < peta) {
return format0(result, bytes, tera, units[3]);
}
if (bytes < exa) {
return format0(result, bytes, peta, units[4]);
}
return format0(result, bytes, exa, units[5]);
}
private String format0(StringBuilder result, long bytes, long base, String unit) {
double doubleValue;
doubleValue = (double) bytes / base;
int integerPart;
integerPart = (int) doubleValue;
result.append(integerPart);
result.append(DECIMAL_SEPARATOR);
double fractionalPart0;
fractionalPart0 = doubleValue - (long) doubleValue;
int fractionalPart;
fractionalPart = (int) (fractionalPart0 * 10);
result.append(fractionalPart);
result.append(' ');
result.append(unit);
return result.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy