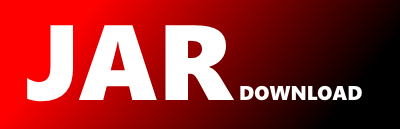
br.com.objectos.lang.Ints Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of objectos-lang Show documentation
Show all versions of objectos-lang Show documentation
A set of utilities for classes of the java.lang and the java.util packages.
The newest version!
/*
* Copyright (C) 2008 The Guava Authors
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*
* Modifications:
*
* Copyright (C) 2011-2021 Objectos Software LTDA.
*
* This file is part of the ObjectosComuns project.
*
* ObjectosComuns is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* ObjectosComuns is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with ObjectosComuns. If not, see .
*/
package br.com.objectos.lang;
import static br.com.objectos.lang.Lang.checkNotNull;
import static br.com.objectos.lang.Lang.isDigit;
import br.com.objectos.lang.Lang.Utf8Bytes;
/**
* A streamlined version of Google Guava's Ints.
*
* Contains only the methods that are currently being used at Objectos Software.
*
* @author Kevin Bourrillion (original Guava version)
*/
final class Ints {
private Ints() {}
/**
* Returns the values from each provided array combined into a single array.
* For example, {@code concat(new int[] {a, b}, new int[] {}, new int[] {c}}
* returns the array {@code {a, b, c}}.
*
* @param arrays
* zero or more {@code int} arrays
* @return a single array containing all the values from the source arrays, in
* order
*/
public static int[] concat(int[]... arrays) {
int length = 0;
for (int[] array : arrays) {
length += array.length;
}
int[] result = new int[length];
int pos = 0;
for (int[] array : arrays) {
System.arraycopy(array, 0, result, pos, array.length);
pos += array.length;
}
return result;
}
/**
* Returns a string containing the supplied {@code int} values separated by
* {@code separator}. For example, {@code join("-", 1, 2, 3)} returns the
* string {@code "1-2-3"}.
*
* @param separator
* the text that should appear between consecutive values in the
* resulting string (but not at the start or end)
* @param array
* an array of {@code int} values, possibly empty
* @return a string containing the supplied {@code int} values separated by
* {@code separator}.
*/
public static String join(String separator, int... array) {
checkNotNull(separator);
if (array.length == 0) {
return "";
}
// For pre-sizing a builder, just get the right order of magnitude
StringBuilder builder = new StringBuilder(array.length * 5);
builder.append(array[0]);
for (int i = 1; i < array.length; i++) {
builder.append(separator).append(array[i]);
}
return builder.toString();
}
public static int parseInt(byte utf8) {
if (!isDigit(utf8)) {
throw new NumberFormatException();
}
return utf8 - Utf8Bytes.DIGIT_0;
}
public static int parseInt(byte[] utf8) {
return parseInt(utf8, 0, utf8.length);
}
public static int parseInt(byte[] utf8, int offset, int length) {
byte b;
int result;
result = 0;
int multiplier;
multiplier = 1;
for (int i = offset + length - 1; i >= offset; i--) {
b = utf8[i];
int digit;
digit = parseInt(b);
digit *= multiplier;
result += digit;
multiplier *= 10;
}
if (result < 0) {
throw new NumberFormatException();
}
return result;
}
public static int parseInt(char c) {
switch (c) {
case '0':
return 0;
case '1':
return 1;
case '2':
return 2;
case '3':
return 3;
case '4':
return 4;
case '5':
return 5;
case '6':
return 6;
case '7':
return 7;
case '8':
return 8;
case '9':
return 9;
default:
throw new NumberFormatException(c + " is not a digit");
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy