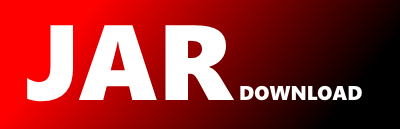
br.com.objectos.lang.MoreArrays Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of objectos-lang Show documentation
Show all versions of objectos-lang Show documentation
A set of utilities for classes of the java.lang and the java.util packages.
The newest version!
/*
* Copyright (C) 2011-2021 Objectos Software LTDA.
*
* This file is part of the ObjectosComuns project.
*
* ObjectosComuns is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* ObjectosComuns is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with ObjectosComuns. If not, see .
*/
package br.com.objectos.lang;
import static br.com.objectos.lang.Lang.checkArgument;
import static br.com.objectos.lang.Lang.checkNotNull;
import java.lang.reflect.Array;
final class MoreArrays {
static final byte[] EMPTY_BYTE_ARRAY = new byte[0];
static final char[] EMPTY_CHAR_ARRAY = new char[0];
static final int[] EMPTY_INT_ARRAY = new int[0];
static final Object[] EMPTY_OBJECT_ARRAY = new Object[0];
private static final int BYTE_MASK = 0xff;
private static final char[] HEX_CHARS = "0123456789abcdef".toCharArray();
private static final int HEX_MASK = 0xf;
private MoreArrays() {}
public static char[] append(char[] array, int offset, char[] value) {
int length;
length = value.length;
int requiredIndex;
requiredIndex = offset + length - 1;
char[] result;
result = growIfNecessary(array, requiredIndex);
System.arraycopy(value, 0, result, offset, length);
return result;
}
public static char[] append(char[] array, int offset, String value) {
char[] valueChars;
valueChars = value.toCharArray();
return append(array, offset, valueChars);
}
public static byte[] growIfNecessary(byte[] array, int requiredIndex) {
checkArgument(requiredIndex >= 0, "failed test: 0 <= requiredIndex < Integer.MAX_VALUE");
int length;
length = array.length;
if (requiredIndex < length) {
return array;
}
int newLength;
newLength = growArrayLength(length, requiredIndex);
byte[] result;
result = new byte[newLength];
System.arraycopy(array, 0, result, 0, length);
return result;
}
public static char[] growIfNecessary(char[] array, int requiredIndex) {
checkArgument(requiredIndex >= 0, "failed test: 0 <= requiredIndex < Integer.MAX_VALUE");
int length;
length = array.length;
if (requiredIndex < length) {
return array;
}
int newLength;
newLength = growArrayLength(length, requiredIndex);
char[] result;
result = new char[newLength];
System.arraycopy(array, 0, result, 0, length);
return result;
}
public static double[] growIfNecessary(double[] array, int requiredIndex) {
checkArgument(requiredIndex >= 0, "failed test: 0 <= requiredIndex < Integer.MAX_VALUE");
int length;
length = array.length;
if (requiredIndex < length) {
return array;
}
int newLength;
newLength = growArrayLength(length, requiredIndex);
double[] result;
result = new double[newLength];
System.arraycopy(array, 0, result, 0, length);
return result;
}
@SuppressWarnings("unchecked")
public static E[] growIfNecessary(E[] array, int requiredIndex) {
checkArgument(requiredIndex >= 0, "failed test: 0 <= requiredIndex < Integer.MAX_VALUE");
int length;
length = array.length;
if (requiredIndex < length) {
return array;
}
int newLength;
newLength = growArrayLength(length, requiredIndex);
E[] result;
Class extends Object[]> arrayType;
arrayType = array.getClass();
if (arrayType == Object[].class) {
result = (E[]) new Object[newLength];
} else {
Class> componentType;
componentType = arrayType.getComponentType();
result = (E[]) Array.newInstance(componentType, newLength);
}
System.arraycopy(array, 0, result, 0, length);
return result;
}
public static int[] growIfNecessary(int[] array, int requiredIndex) {
checkArgument(requiredIndex >= 0, "failed test: 0 <= requiredIndex < Integer.MAX_VALUE");
int length;
length = array.length;
if (requiredIndex < length) {
return array;
}
int newLength;
newLength = growArrayLength(length, requiredIndex);
int[] result;
result = new int[newLength];
System.arraycopy(array, 0, result, 0, length);
return result;
}
public static int[][] growIfNecessary(int[][] array, int requiredIndex) {
checkArgument(requiredIndex >= 0, "failed test: 0 <= requiredIndex < Integer.MAX_VALUE");
int length;
length = array.length;
if (requiredIndex < length) {
return array;
}
int newLength;
newLength = growArrayLength(length, requiredIndex);
int[][] result;
result = new int[newLength][];
System.arraycopy(array, 0, result, 0, length);
return result;
}
public static long longValueUnchecked(byte[] bytes) {
long result = bytes[0] & BYTE_MASK;
result = (result << 8) | (bytes[1] & BYTE_MASK);
result = (result << 8) | (bytes[2] & BYTE_MASK);
result = (result << 8) | (bytes[3] & BYTE_MASK);
result = (result << 8) | (bytes[4] & BYTE_MASK);
result = (result << 8) | (bytes[5] & BYTE_MASK);
result = (result << 8) | (bytes[6] & BYTE_MASK);
result = (result << 8) | (bytes[7] & BYTE_MASK);
return result;
}
public static String toHexStringNonEmpty(byte[] bytes, int offset, int length) {
char[] result;
result = new char[length * 2];
for (int i = 0, j = 0; i < length; i++) {
int b;
b = bytes[offset + i] & BYTE_MASK;
result[j++] = HEX_CHARS[b >>> 4];
result[j++] = HEX_CHARS[b & HEX_MASK];
}
return new String(result);
}
static int growArrayLength(int currentLength, int requiredIndex) {
int newLength;
newLength = currentLength + (currentLength >> 1);
if (requiredIndex < newLength) {
return newLength;
}
int requiredLength;
requiredLength = requiredIndex + 1;
newLength = Integer.highestOneBit(requiredLength) << 1;
if (newLength > 0) {
return newLength;
}
return Integer.MAX_VALUE;
}
static long longValue(byte[] bytes) {
checkNotNull(bytes, "bytes == null");
checkArgument(bytes.length == 8);
return longValueUnchecked(bytes);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy