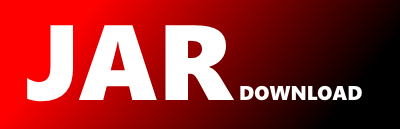
br.com.objectos.lang.MoreObjects Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of objectos-lang Show documentation
Show all versions of objectos-lang Show documentation
A set of utilities for classes of the java.lang and the java.util packages.
The newest version!
/*
* Copyright (C) 2011-2021 Objectos Software LTDA.
*
* This file is part of the ObjectosComuns project.
*
* ObjectosComuns is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* ObjectosComuns is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with ObjectosComuns. If not, see .
*/
package br.com.objectos.lang;
import static br.com.objectos.lang.Lang.checkNotNull;
final class MoreObjects {
static final int HASH_CODE_MULTIPLIER = 31;
static final int HASH_CODE_START = 17;
private MoreObjects() {}
public static boolean equals(Object first, Object second) {
if (first == second) {
return true;
}
if (first != null) {
return first.equals(second);
}
return false;
}
public static int hashCode(int hc1, int hc2) {
int result = HASH_CODE_START;
result = hashCode0(result, hc1);
result = hashCode0(result, hc2);
return result;
}
public static int hashCode(int hc1, int hc2, int hc3) {
int result = HASH_CODE_START;
result = hashCode0(result, hc1);
result = hashCode0(result, hc2);
result = hashCode0(result, hc3);
return result;
}
public static int hashCode(int hc1, int hc2, int hc3, int hc4) {
int result = HASH_CODE_START;
result = hashCode0(result, hc1);
result = hashCode0(result, hc2);
result = hashCode0(result, hc3);
result = hashCode0(result, hc4);
return result;
}
public static int hashCode(int hc1, int hc2, int hc3, int hc4, int hc5) {
int result = HASH_CODE_START;
result = hashCode0(result, hc1);
result = hashCode0(result, hc2);
result = hashCode0(result, hc3);
result = hashCode0(result, hc4);
result = hashCode0(result, hc5);
return result;
}
public static int hashCode(int hc1, int hc2, int hc3, int hc4, int hc5, int... rest) {
checkNotNull(rest, "rest == null");
int result = HASH_CODE_START;
result = hashCode0(result, hc1);
result = hashCode0(result, hc2);
result = hashCode0(result, hc3);
result = hashCode0(result, hc4);
result = hashCode0(result, hc5);
for (int hc : rest) {
result = hashCode0(result, hc);
}
return result;
}
public static int hashCode(Object o) {
if (o == null) {
return 0;
}
return o.hashCode();
}
public static int hashCode(Object o1, Object o2) {
return hashCode(
hashCode(o1),
hashCode(o2)
);
}
public static int hashCode(Object o1, Object o2, Object o3) {
return hashCode(
hashCode(o1),
hashCode(o2),
hashCode(o3)
);
}
public static int hashCode(Object o1, Object o2, Object o3, Object o4) {
return hashCode(
hashCode(o1),
hashCode(o2),
hashCode(o3),
hashCode(o4)
);
}
public static int hashCode(Object o1, Object o2, Object o3, Object o4, Object o5) {
return hashCode(
hashCode(o1),
hashCode(o2),
hashCode(o3),
hashCode(o4),
hashCode(o5)
);
}
public static int hashCode(
Object o1, Object o2, Object o3, Object o4, Object o5, Object... rest) {
checkNotNull(rest, "rest == null");
int result = HASH_CODE_START;
result = hashCode0(result, hashCode(o1));
result = hashCode0(result, hashCode(o2));
result = hashCode0(result, hashCode(o3));
result = hashCode0(result, hashCode(o4));
result = hashCode0(result, hashCode(o5));
for (Object o : rest) {
result = hashCode0(result, hashCode(o));
}
return result;
}
static int hashCode0(int current, int hashCode) {
return HASH_CODE_MULTIPLIER * current + hashCode;
}
static int hashCode0(int partial, Object o) {
return hashCode0(partial, hashCode(o));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy