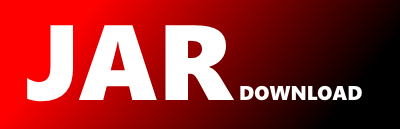
br.com.objectos.lang.StringConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of objectos-lang Show documentation
Show all versions of objectos-lang Show documentation
A set of utilities for classes of the java.lang and the java.util packages.
The newest version!
/*
* Copyright (C) 2011-2021 Objectos Software LTDA.
*
* This file is part of the ObjectosComuns project.
*
* ObjectosComuns is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* ObjectosComuns is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with ObjectosComuns. If not, see .
*/
package br.com.objectos.lang;
import java.util.ArrayList;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
public abstract class StringConverter {
protected StringConverter() {}
public static Builder builder() {
return new Builder();
}
public abstract String convert(String text);
public final static class Builder {
private final Set factories = new LinkedHashSet(4);
Builder() {}
public final StringConverter build() {
int size = factories.size();
switch (size) {
case 0:
return Noop.INSTANCE;
case 1:
CharConverterFactory format = factories.iterator().next();
return new SingleFormat(format);
default:
return new ManyFormats(factories);
}
}
public final Builder stripWhitespace() {
factories.add(StripWhitespace.INSTANCE);
return this;
}
public final Builder toAlphanum() {
factories.add(Alphanum.INSTANCE);
return this;
}
public final Builder whitespaceTo(char replacement) {
factories.add(new WhitespaceTo(replacement));
return this;
}
public final Builder withCustomConverter(CharConverterFactory factory) {
factories.add(factory);
return this;
}
}
private static class ManyFormats extends StringConverter {
private final Set factorySet;
private ManyFormats(Set factorySet) {
this.factorySet = factorySet;
}
@Override
public final String convert(String text) {
StringBuilder s = new StringBuilder();
List formatList;
formatList = new ArrayList(factorySet.size());
for (CharConverterFactory factory : factorySet) {
formatList.add(factory.get());
}
char[] chars = text.toCharArray();
for (char c : chars) {
boolean apply = false;
char theChar = c;
for (CharConverter f : formatList) {
if (apply = f.apply(theChar)) {
theChar = f.convert(theChar);
} else {
break;
}
}
if (apply) {
s.append(theChar);
}
}
return s.toString();
}
}
private static class Noop extends StringConverter {
static final StringConverter INSTANCE = new Noop();
@Override
public final String convert(String text) {
return text;
}
}
private static class SingleFormat extends StringConverter {
private final CharConverterFactory factory;
public SingleFormat(CharConverterFactory factory) {
this.factory = factory;
}
@Override
public final String convert(String text) {
StringBuilder s = new StringBuilder();
char[] chars = text.toCharArray();
CharConverter format = factory.get();
for (char c : chars) {
if (format.apply(c)) {
s.append(format.convert(c));
}
}
return s.toString();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy