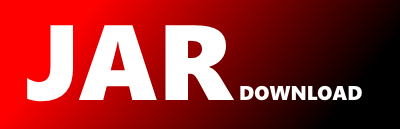
br.com.objectos.lang.Strings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of objectos-lang Show documentation
Show all versions of objectos-lang Show documentation
A set of utilities for classes of the java.lang and the java.util packages.
The newest version!
/*
* Copyright (C) 2010 The Guava Authors
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*
* Modifications:
*
* Copyright (C) 2011-2021 Objectos Software LTDA.
*
* This file is part of the ObjectosComuns project.
*
* ObjectosComuns is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* ObjectosComuns is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with ObjectosComuns. If not, see .
*/
package br.com.objectos.lang;
import java.text.Normalizer;
import java.text.Normalizer.Form;
/**
* A streamlined version of Google Guava's Strings.
*
* @author Kevin Bourrillion (original Guava version)
*/
final class Strings {
private static final StringConverter STRIP_WHITESPACE = StringConverter.builder()
.stripWhitespace()
.build();
private static final StringConverter TO_ALPHANUM = StringConverter.builder()
.toAlphanum()
.build();
private Strings() {}
public static String emptyToNull(String string) {
return isNullOrEmpty(string) ? null : string;
}
public static boolean isNullOrEmpty(String string) {
return string == null || string.isEmpty();
}
public static String nullToEmpty(String string) {
return string == null ? "" : string;
}
public static String stripDiacritics(String value) {
String normalized;
normalized = Normalizer.normalize(value, Form.NFD);
char[] charArray;
charArray = normalized.toCharArray();
int length;
length = charArray.length;
StringBuilder sb;
sb = new StringBuilder(length);
for (char c : charArray) {
// https://unicode.org/charts/PDF/U0300.pdf
if (c >= '\u0300' && c <= '\u036F') {
continue;
}
sb.append(c);
}
return sb.toString();
}
public static String stripWhitespace(String value) {
return STRIP_WHITESPACE.convert(value);
}
public static String toAlphanum(String value) {
return TO_ALPHANUM.convert(value);
}
public static String toCamelCase(String value) {
boolean wasWhitespace;
wasWhitespace = true;
char[] charArray;
charArray = value.toCharArray();
StringBuilder sb;
sb = new StringBuilder(charArray.length);
for (char c : charArray) {
char theChar = c;
if (Character.isWhitespace(c)) {
wasWhitespace = true;
}
else if (wasWhitespace) {
theChar = Character.toUpperCase(c);
wasWhitespace = false;
}
sb.append(theChar);
}
return sb.toString();
}
public static int[] toCodePoints(String string) {
int stringLength;
stringLength = string.length();
if (stringLength == 0) {
return MoreArrays.EMPTY_INT_ARRAY;
}
char[] charArray;
charArray = string.toCharArray();
if (stringLength == 1) {
char onlyChar = charArray[0];
return new int[] {onlyChar};
}
int[] intArray;
intArray = new int[stringLength];
int intIndex;
intIndex = 0;
for (int charIndex = 0; charIndex < charArray.length; charIndex++) {
char highOrBmp;
highOrBmp = charArray[charIndex];
intArray[intIndex] = highOrBmp;
if (!Character.isHighSurrogate(highOrBmp)) {
intIndex++;
continue;
}
char low;
low = charArray[charIndex + 1];
if (!Character.isLowSurrogate(low)) {
intIndex++;
continue;
}
charIndex++;
int codePoint;
codePoint = Character.toCodePoint(highOrBmp, low);
intArray[intIndex] = codePoint;
intIndex++;
}
if (intIndex == intArray.length) {
return intArray;
} else {
int[] result;
result = new int[intIndex];
System.arraycopy(intArray, 0, result, 0, intIndex);
return result;
}
}
public static String whitespaceTo(String text, char replacement) {
return StringConverter.builder()
.whitespaceTo(replacement)
.build()
.convert(text);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy