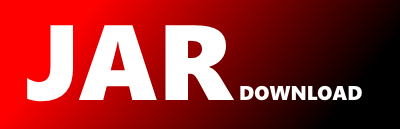
br.com.objectos.lang.ToString Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of objectos-lang Show documentation
Show all versions of objectos-lang Show documentation
A set of utilities for classes of the java.lang and the java.util packages.
The newest version!
/*
* Copyright (C) 2011-2021 Objectos Software LTDA.
*
* This file is part of the ObjectosComuns project.
*
* ObjectosComuns is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* ObjectosComuns is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with ObjectosComuns. If not, see .
*/
package br.com.objectos.lang;
import static br.com.objectos.lang.Lang.checkNotNull;
import java.util.Arrays;
import java.util.Map.Entry;
final class ToString {
private static final char[][] INDENTATION = new char[][] {
" ".toCharArray(),
" ".toCharArray(),
" ".toCharArray(),
" ".toCharArray()
};
private ToString() {}
public static void formatToString(
StringBuilder sb, int depth, Object source) {
checkNotNull(sb, "sb == null");
checkNotNull(source, "source == null");
formatStart(sb, source);
char[] indentation;
indentation = i(depth);
formatEnd(sb, indentation);
}
public static void formatToString(
StringBuilder sb, int depth, Object source,
String name, Object value) {
checkNotNull(sb, "sb == null");
checkNotNull(source, "source == null");
checkNotNull(name, "name == null");
formatStart(sb, source);
char[] indentation;
indentation = i(depth);
formatFirstValue(sb, depth, indentation, name, value);
formatEnd(sb, indentation);
}
public static void formatToString(
StringBuilder sb, int depth, Object source,
String name1, Object value1,
String name2, Object value2) {
checkNotNull(sb, "sb == null");
checkNotNull(source, "source == null");
checkNotNull(name1, "name1 == null");
checkNotNull(name2, "name2 == null");
formatStart(sb, source);
char[] indentation;
indentation = i(depth);
formatFirstValue(sb, depth, indentation, name1, value1);
formatNextValue(sb, depth, indentation, name2, value2);
formatEnd(sb, indentation);
}
public static void formatToString(
StringBuilder sb, int depth, Object source,
String name1, Object value1,
String name2, Object value2,
String name3, Object value3) {
checkNotNull(sb, "sb == null");
checkNotNull(source, "source == null");
checkNotNull(name1, "name1 == null");
checkNotNull(name2, "name2 == null");
checkNotNull(name3, "name3 == null");
formatStart(sb, source);
char[] indentation;
indentation = i(depth);
formatFirstValue(sb, depth, indentation, name1, value1);
formatNextValue(sb, depth, indentation, name2, value2);
formatNextValue(sb, depth, indentation, name3, value3);
formatEnd(sb, indentation);
}
public static void formatToString(
StringBuilder sb, int depth, Object source,
String name1, Object value1,
String name2, Object value2,
String name3, Object value3,
String name4, Object value4) {
checkNotNull(sb, "sb == null");
checkNotNull(source, "source == null");
checkNotNull(name1, "name1 == null");
checkNotNull(name2, "name2 == null");
checkNotNull(name3, "name3 == null");
checkNotNull(name4, "name4 == null");
formatStart(sb, source);
char[] indentation;
indentation = i(depth);
formatFirstValue(sb, depth, indentation, name1, value1);
formatNextValue(sb, depth, indentation, name2, value2);
formatNextValue(sb, depth, indentation, name3, value3);
formatNextValue(sb, depth, indentation, name4, value4);
formatEnd(sb, indentation);
}
public static void formatToString(
StringBuilder sb, int depth, Object source,
String name1, Object value1,
String name2, Object value2,
String name3, Object value3,
String name4, Object value4,
String name5, Object value5) {
checkNotNull(sb, "sb == null");
checkNotNull(source, "source == null");
checkNotNull(name1, "name1 == null");
checkNotNull(name2, "name2 == null");
checkNotNull(name3, "name3 == null");
checkNotNull(name4, "name4 == null");
checkNotNull(name5, "name5 == null");
formatStart(sb, source);
char[] indentation;
indentation = i(depth);
formatFirstValue(sb, depth, indentation, name1, value1);
formatNextValue(sb, depth, indentation, name2, value2);
formatNextValue(sb, depth, indentation, name3, value3);
formatNextValue(sb, depth, indentation, name4, value4);
formatNextValue(sb, depth, indentation, name5, value5);
formatEnd(sb, indentation);
}
public static void formatToString(
StringBuilder sb, int depth, Object source,
String name1, Object value1,
String name2, Object value2,
String name3, Object value3,
String name4, Object value4,
String name5, Object value5,
String name6, Object value6) {
checkNotNull(sb, "sb == null");
checkNotNull(source, "source == null");
checkNotNull(name1, "name1 == null");
checkNotNull(name2, "name2 == null");
checkNotNull(name3, "name3 == null");
checkNotNull(name4, "name4 == null");
checkNotNull(name5, "name5 == null");
checkNotNull(name6, "name6 == null");
formatStart(sb, source);
char[] indentation;
indentation = i(depth);
formatFirstValue(sb, depth, indentation, name1, value1);
formatNextValue(sb, depth, indentation, name2, value2);
formatNextValue(sb, depth, indentation, name3, value3);
formatNextValue(sb, depth, indentation, name4, value4);
formatNextValue(sb, depth, indentation, name5, value5);
formatNextValue(sb, depth, indentation, name6, value6);
formatEnd(sb, indentation);
}
public static void formatToStringList(
StringBuilder sb, int depth, Object source, ToStringList list) {
checkNotNull(source, "source == null");
checkNotNull(list, "list == null");
formatStart(sb, source);
char[] indentation;
indentation = i(depth);
int size;
size = list.size();
if (size > 0) {
int length;
length = computeLength(size);
String name;
name = getName(length, 0);
Object value;
value = list.getValue(0);
formatFirstValue(sb, depth, indentation, name, value);
for (int i = 1; i < size; i++) {
name = getName(length, i);
value = list.getValue(i);
formatNextValue(sb, depth, indentation, name, value);
}
}
formatEnd(sb, indentation);
}
public static void formatToStringMap(
StringBuilder sb, int depth, Object source, ToStringMap map) {
checkNotNull(source, "source == null");
checkNotNull(map, "map == null");
formatStart(sb, source);
char[] indentation;
indentation = i(depth);
if (map.hasNext()) {
Entry, ?> entry;
entry = map.next();
Object key;
key = entry.getKey();
Object value;
value = entry.getValue();
formatFirstValue(sb, depth, indentation, key, value);
while (map.hasNext()) {
entry = map.next();
key = entry.getKey();
value = entry.getValue();
formatNextValue(sb, depth, indentation, key, value);
}
}
formatEnd(sb, indentation);
}
public static String toString(
Object source) {
StringBuilder sb;
sb = new StringBuilder();
formatToString(
sb, 0, source
);
return sb.toString();
}
public static String toString(
Object source,
String name, Object value) {
StringBuilder sb;
sb = new StringBuilder();
formatToString(
sb, 0, source,
name, value
);
return sb.toString();
}
public static String toString(
Object source,
String name1, Object value1,
String name2, Object value2) {
StringBuilder sb;
sb = new StringBuilder();
formatToString(
sb, 0, source,
name1, value1,
name2, value2
);
return sb.toString();
}
public static String toString(
Object source,
String name1, Object value1,
String name2, Object value2,
String name3, Object value3) {
StringBuilder sb;
sb = new StringBuilder();
formatToString(
sb, 0, source,
name1, value1,
name2, value2,
name3, value3
);
return sb.toString();
}
public static String toString(
Object source,
String name1, Object value1,
String name2, Object value2,
String name3, Object value3,
String name4, Object value4) {
StringBuilder sb;
sb = new StringBuilder();
formatToString(
sb, 0, source,
name1, value1,
name2, value2,
name3, value3,
name4, value4
);
return sb.toString();
}
public static String toString(
Object source,
String name1, Object value1,
String name2, Object value2,
String name3, Object value3,
String name4, Object value4,
String name5, Object value5) {
StringBuilder sb;
sb = new StringBuilder();
formatToString(
sb, 0, source,
name1, value1,
name2, value2,
name3, value3,
name4, value4,
name5, value5
);
return sb.toString();
}
public static String toString(
Object source,
String name1, Object value1,
String name2, Object value2,
String name3, Object value3,
String name4, Object value4,
String name5, Object value5,
String name6, Object value6) {
StringBuilder sb;
sb = new StringBuilder();
formatToString(
sb, 0, source,
name1, value1,
name2, value2,
name3, value3,
name4, value4,
name5, value5,
name6, value6
);
return sb.toString();
}
public static String toString(ToStringObject object) {
checkNotNull(object, "object == null");
StringBuilder out;
out = new StringBuilder();
object.formatToString(out, 0);
return out.toString();
}
private static int computeLength(int size) {
if (size < 10) {
return 1;
}
else if (size < 100) {
return 2;
}
else {
double l;
l = Math.log10(size);
double f;
f = Math.floor(l);
return (int) f;
}
}
private static void formatEnd(StringBuilder sb, char[] indentation) {
sb.append(indentation, 0, indentation.length - 2);
sb.append(']');
}
private static void formatFirstValue(
StringBuilder sb, int depth, char[] indentation, Object key, Object value) {
sb.append('\n');
formatNextValue(sb, depth, indentation, key, value);
}
private static void formatNextValue(
StringBuilder sb, int depth, char[] indentation, Object key, Object value) {
sb.append(indentation);
// key
if (key instanceof String) {
String s;
s = (String) key;
if (!s.isEmpty()) {
sb.append(s);
sb.append('=');
}
}
else if (key instanceof ToStringObject) {
ToStringObject o;
o = (ToStringObject) key;
o.formatToString(sb, depth + 1);
sb.append('=');
}
// value
if (value == null) {
sb.append("null");
}
else if (value instanceof String) {
String s;
s = (String) value;
sb.append(s);
}
else if (value instanceof ToStringObject) {
ToStringObject o;
o = (ToStringObject) value;
o.formatToString(sb, depth + 1);
}
else {
String s;
s = value.toString();
sb.append(s);
}
sb.append('\n');
}
private static void formatStart(StringBuilder sb, Object source) {
if (source instanceof String) {
String s;
s = (String) source;
sb.append(s);
}
else {
Class extends Object> type;
type = source.getClass();
sb.append(type.getSimpleName());
}
sb.append('[');
}
private static String getName(int length, int index) {
StringBuilder sb;
sb = new StringBuilder();
String s;
s = Integer.toString(index);
int indexLength;
indexLength = s.length();
int diff;
diff = length - indexLength;
for (int i = 0; i < diff; i++) {
sb.append(' ');
}
sb.append(s);
return sb.toString();
}
private static char[] i(int depth) {
if (depth < 0) {
throw new IllegalArgumentException("depth=" + depth + " < 0");
}
if (depth < INDENTATION.length) {
return INDENTATION[depth];
}
char[] r;
r = new char[2 * depth + 2];
Arrays.fill(r, ' ');
return r;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy