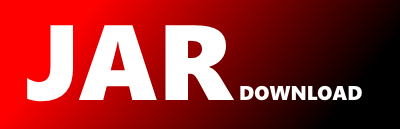
br.com.objectos.schema.CreateTableDslPojo Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2016 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.schema;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import br.com.objectos.core.util.MoreCollectors;
import br.com.objectos.schema.info.IntColumnKind;
import br.com.objectos.schema.info.LocalDateTimeColumnKind;
import br.com.objectos.schema.info.StringColumnKind;
/**
* @author [email protected] (Marcio Endo)
*/
class CreateTableDslPojo
implements
CreateColumnDsl,
CreatePrimaryKey,
CreateTableDsl {
private final String tableName;
private final List columnTypeList = new ArrayList<>();
private final List primaryKeyColumnRefList = new ArrayList<>();
private ColumnName columnName;
public CreateTableDslPojo(String tableName) {
this.tableName = tableName;
}
@Override
public CreateTable compile() {
return CreateTable.thisBuilder()
.tableName(tableName)
.columnDdlList(columnTypeList.stream()
.map(AbstractCreateColumnDsl::toColumnDdl)
.collect(MoreCollectors.toImmutableList()))
.primaryKeyDef(PrimaryKeyDef.of(primaryKeyColumnRefList))
.build();
}
@Override
public CreateColumnDsl column(ColumnName columnName) {
this.columnName = Objects.requireNonNull(columnName);
return this;
}
@Override
public CreateColumnDsl column(String columnName) {
this.columnName = Columns.named(columnName);
return this;
}
@Override
public LocalDateTimeColumnDsl timestamp() {
return localDateTimeColumnType(LocalDateTimeColumnKind.TIMESTAMP);
}
@Override
public IntegerColumnDsl tinyint() {
return intTypeColumn(IntColumnKind.TINYINT);
}
@Override
public StringCreateColumnDsl varchar(int length) {
return stringColumnType(StringColumnKind.VARCHAR, length);
}
CreatePrimaryKey primaryKey(ColumnName columnRef) {
primaryKeyColumnRefList.add(columnRef);
return this;
}
CreatePrimaryKey primaryKey(String columnName) {
AbstractCreateColumnDsl column = columnTypeList.stream()
.filter(dsl -> dsl.matches(columnName))
.findFirst()
.orElseThrow(IllegalArgumentException::new);
return primaryKey(column.columnName);
}
private IntegerColumnDslPojo intTypeColumn(IntColumnKind kind) {
IntegerColumnDslPojo pojo = new IntegerColumnDslPojo(this, columnName, kind);
columnTypeList.add(pojo);
return pojo;
}
private LocalDateTimeColumnDslPojo localDateTimeColumnType(LocalDateTimeColumnKind kind) {
LocalDateTimeColumnDslPojo pojo = new LocalDateTimeColumnDslPojo(this, columnName, kind);
columnTypeList.add(pojo);
return pojo;
}
private StringCreateColumnDslPojo stringColumnType(StringColumnKind kind, int length) {
StringCreateColumnDslPojo pojo = new StringCreateColumnDslPojo(this, columnName, kind, length);
columnTypeList.add(pojo);
return pojo;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy