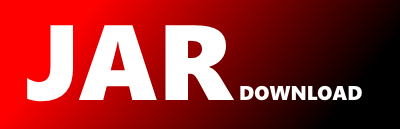
br.com.objectos.core.testing.SimpleTestable Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2015 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.core.testing;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
import javax.lang.model.element.Modifier;
import br.com.objectos.pojo.plugin.Naming;
import br.com.objectos.pojo.plugin.Property;
import br.com.objectos.testable.NotTestable;
import com.squareup.javapoet.CodeBlock;
import com.squareup.javapoet.MethodSpec;
import com.squareup.javapoet.ParameterSpec;
import com.squareup.javapoet.TypeName;
/**
* @author [email protected] (Marcio Endo)
*/
class SimpleTestable implements IsTestable {
final Naming naming;
final TypeName testableOf;
public SimpleTestable(Naming naming, TypeName testableOf) {
this.naming = naming;
this.testableOf = testableOf;
}
@Override
public final Optional get(List propertyList) {
List header = header();
List body = body(propertyList);
return body.isEmpty()
? Optional. empty()
: Optional.of(isEqual(header, body));
}
List header() {
return Collections.emptyList();
}
List body(List propertyList) {
return propertyList.stream()
.filter(property -> !property.hasAnnotation(NotTestable.class))
.map(property -> CodeBlock.builder()
.add(" .equal($L, that.$L())\n", property.name(), property.accessorName())
.build())
.collect(Collectors.toList());
}
String parameterName() {
return "that";
}
private MethodSpec isEqual(List header, List body) {
MethodSpec.Builder b = MethodSpec.methodBuilder("isEqual")
.addModifiers(Modifier.PUBLIC)
.addAnnotation(Override.class)
.returns(boolean.class)
.addParameter(parameterSpec());
for (CodeBlock code : header) {
b.addCode(code);
}
b.addCode("return $T.isEqualHelper()\n", testablesClass());
for (CodeBlock code : body) {
b.addCode(code);
}
b.addStatement(" .result()");
return b.build();
}
Class> testablesClass() {
return Testables.class;
}
private ParameterSpec parameterSpec() {
return ParameterSpec.builder(testableOf, parameterName()).build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy