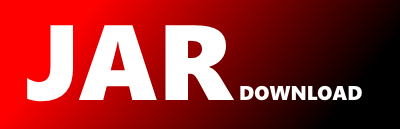
br.com.objectos.way.schema.info.ColumnInfoAnnotationInfo Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2015 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.schema.info;
import java.lang.annotation.Annotation;
import javax.lang.model.element.Modifier;
import br.com.objectos.way.code.AnnotationInfo;
import br.com.objectos.way.code.TypeInfo;
import br.com.objectos.way.db.SortOrder;
import br.com.objectos.way.lazy.Lazy;
import br.com.objectos.way.schema.meta.ColumnClass;
import br.com.objectos.way.schema.meta.ColumnName;
import br.com.objectos.way.schema.meta.MigrationPrefix;
import br.com.objectos.way.schema.meta.Nullable;
import br.com.objectos.way.schema.meta.TableClassName;
import br.com.objectos.way.testable.Equality;
import br.com.objectos.way.testable.Tester;
import com.squareup.javapoet.ClassName;
import com.squareup.javapoet.MethodSpec;
import com.squareup.javapoet.TypeName;
/**
* @author [email protected] (Marcio Endo)
*/
class ColumnInfoAnnotationInfo implements ColumnInfo {
private static final Tester TESTER = Tester.of(ColumnInfo.class)
.add("tableName", o -> o.tableName())
.add("simpleName", o -> o.simpleName())
.add("nullable", o -> o.nullable())
.build();
private final AnnotationInfo annotationInfo;
private final Lazy tableName = new LazyTableName();
private ColumnInfoAnnotationInfo(AnnotationInfo annotationInfo) {
this.annotationInfo = annotationInfo;
}
public static ColumnInfoAnnotationInfo of(AnnotationInfo annotationInfo) {
return new ColumnInfoAnnotationInfo(annotationInfo);
}
public TypeName annotationTypeName() {
return annotationInfo.enclosingTypeInfo().get()
.annotationInfo(TableClassName.class)
.flatMap(ann -> ann.stringValue("value"))
.map(s -> ClassName.bestGuess(s))
.map(className -> className.nestedClass(annotationInfo.simpleName()))
.get();
}
public MethodSpec columnClassMethod() {
TypeInfo columnClass = annotationInfo.annotationInfo(ColumnClass.class)
.flatMap(ann -> ann.simpleTypeInfoValue("value"))
.flatMap(simpleTypeInfo -> simpleTypeInfo.typeInfo())
.get();
ClassName tableClassName = columnClass.enclosingTypeInfo()
.flatMap(typeInfo -> typeInfo.annotationInfo(TableClassName.class))
.map(ann -> ann.stringValue("value", ""))
.map(s -> ClassName.bestGuess(s))
.get();
ClassName columnClassName = tableClassName.nestedClass(columnClass.simpleName());
return MethodSpec.methodBuilder(columnClass.simpleName())
.addModifiers(Modifier.PUBLIC)
.returns(columnClassName)
.addStatement("$T table = $T.get()", tableClassName, tableClassName)
.addStatement("return table.$L(get())", annotationInfo.simpleName())
.build();
}
@Override
public Equality isEqualTo(Object o) {
return TESTER.test(this, o);
}
@Override
public GenerationInfo generationInfo() {
return ThisNoGenerationInfo.get();
}
@Override
public boolean nullable() {
return annotationInfo.hasAnnotation(Nullable.class);
}
public String orderBy(SortOrder sortOrder) {
String tableVarName = annotationInfo.enclosingTypeInfo().get().simpleName();
return String.format("%s.%s().%s()", tableVarName, annotationInfo.simpleName(), sortOrder.lowerCase());
}
@Override
public String simpleName() {
return stringValue(ColumnName.class);
}
@Override
public TableName tableName() {
return tableName.get();
}
private String stringValue(Class extends Annotation> type) {
return annotationInfo.annotationInfo(type)
.flatMap(annotationInfo -> annotationInfo.stringValue("value"))
.get();
}
private class LazyTableName extends Lazy {
@Override
protected TableName compute() {
return TableName.builder()
.schemaName(schemaName())
.simpleName(stringValue(br.com.objectos.way.schema.meta.TableName.class))
.migrationVersion(migrationVersion())
.build();
}
private MigrationVersion migrationVersion() {
return MigrationVersion.builder()
.prefix(annotationInfo.enclosingTypeInfo()
.flatMap(typeInfo -> typeInfo.annotationInfo(MigrationPrefix.class))
.flatMap(annotationInfo -> annotationInfo.stringValue("value"))
.get())
.build();
}
private SchemaName schemaName() {
return SchemaName.builder()
.simpleName(stringValue(br.com.objectos.way.schema.meta.SchemaName.class))
.build();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy