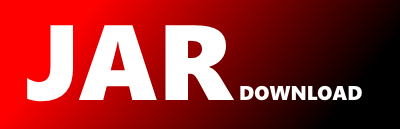
br.com.objectos.way.sql.AbstractSelectNode Maven / Gradle / Ivy
/*
* Copyright 2014-2015 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.sql;
import java.lang.reflect.Type;
import java.util.Arrays;
import java.util.List;
import java.util.function.Consumer;
import javax.lang.model.element.Modifier;
import br.com.objectos.way.db.Dialect;
import br.com.objectos.way.db.IntTypeColumn;
import br.com.objectos.way.db.LocalDateTypeColumn;
import br.com.objectos.way.db.Order;
import br.com.objectos.way.db.Orderable;
import br.com.objectos.way.db.ParameterBinder;
import br.com.objectos.way.db.SelectBuilder;
import br.com.objectos.way.db.StringTypeColumn;
import com.squareup.javapoet.ArrayTypeName;
import com.squareup.javapoet.ClassName;
import com.squareup.javapoet.MethodSpec;
import com.squareup.javapoet.TypeVariableName;
/**
* @author [email protected] (Marcio Endo)
*/
abstract class AbstractSelectNode extends AbstractHasLevel {
public AbstractSelectNode(Level level) {
super(level);
}
MethodSpec constructorSelectWhere() {
return MethodSpec.constructorBuilder()
.addParameter(selectNodeTypeName(), "previous")
.addParameter(Naming.Keyword, "keyword")
.addParameter(Naming.V, "first")
.addStatement("super(previous)")
.addStatement("this.keyword = keyword")
.addStatement("this.first = first")
.build();
}
MethodSpec andInt(Consumer statement) {
return andType(statement, IntTypeColumn.class, simpleSelectWhereIntClassName());
}
MethodSpec andLocalDate(Consumer statement) {
return andType(statement, LocalDateTypeColumn.class, simpleSelectWhereLocalDateClassName());
}
MethodSpec andString(Consumer statement) {
return andType(statement, StringTypeColumn.class, simpleSelectWhereStringClassName());
}
MethodSpec bind0Condition() {
return MethodSpec.methodBuilder("bind0")
.addAnnotation(Override.class)
.returns(ParameterBinder.class)
.addParameter(ParameterBinder.class, "binder")
.beginControlFlow("if (condition != null)")
.addStatement("condition.bind(binder)")
.endControlFlow()
.addStatement("return binder")
.build();
}
MethodSpec compile() {
return compile(builder -> {
});
}
MethodSpec compile(Consumer statement) {
MethodSpec.Builder compile = MethodSpec.methodBuilder("compile")
.addAnnotation(Override.class)
.addModifiers(Modifier.PUBLIC)
.addParameter(Dialect.class, "dialect")
.returns(parameterizedTypeNameLevel("SimpleSelectQuery"));
statement.accept(compile);
return compile
.addStatement("return new $T<>(dialect, this)", classNameLevel("SimpleSelectQuery"))
.build();
}
MethodSpec orderBy0() {
return MethodSpec.methodBuilder("orderBy0")
.addModifiers(Modifier.PRIVATE)
.addParameter(ArrayTypeName.of(Order.class), "columns")
.varargs()
.returns(simpleSelectOrderByTypeName())
.addStatement("return new $T<>(this, columns)", classNameLevel("SimpleSelectOrderBy"))
.build();
}
MethodSpec orderBy0(Consumer statement) {
MethodSpec.Builder orderBy0 = MethodSpec.methodBuilder("orderBy0")
.addModifiers(Modifier.PRIVATE)
.addParameter(ArrayTypeName.of(Order.class), "columns")
.varargs()
.returns(simpleSelectOrderByTypeName());
statement.accept(orderBy0);
return orderBy0
.addStatement("return new $T<>(this, columns)", classNameLevel("SimpleSelectOrderBy"))
.build();
}
List orderBy() {
return Arrays.asList(
orderBy(Orderable.class, "orderBy(o1.asc())"),
orderBy(Order.class, "orderBy0(o1)"));
}
MethodSpec write0Condition() {
return MethodSpec.methodBuilder("write0")
.addAnnotation(Override.class)
.returns(SelectBuilder.class)
.addParameter(SelectBuilder.class, "sql")
.addStatement("return condition != null ? sql.where(condition) : sql")
.build();
}
private MethodSpec andType(Consumer statement, Class> column, ClassName className) {
MethodSpec.Builder method = MethodSpec.methodBuilder("and")
.addAnnotation(Override.class)
.addModifiers(Modifier.PUBLIC)
.addTypeVariable(TypeVariableName.get("X", column))
.returns(parameterizedTypeName(className, Naming.X))
.addParameter(Naming.X, "column");
statement.accept(method);
return method
.addStatement("return new $T<>(this, $T.AND, column)", className, Naming.Keyword)
.build();
}
private MethodSpec orderBy(Type type, String statement) {
return MethodSpec.methodBuilder("orderBy")
.addAnnotation(Override.class)
.addModifiers(Modifier.PUBLIC)
.addParameter(type, "o1")
.returns(simpleSelectOrderByTypeName())
.addStatement("return $L", statement)
.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy