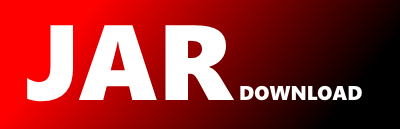
br.com.objectos.ui.html.ElementMethod Maven / Gradle / Ivy
/*
* Copyright 2015 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.ui.html;
import java.util.List;
import java.util.stream.Collectors;
import javax.lang.model.element.Modifier;
import br.com.objectos.way.pojo.Pojo;
import br.com.objectos.way.testable.Testable;
import br.com.objectos.way.code.MethodInfo;
import com.squareup.javapoet.ClassName;
import com.squareup.javapoet.JavaFile;
import com.squareup.javapoet.MethodSpec;
import com.squareup.javapoet.TypeName;
import com.squareup.javapoet.TypeSpec;
/**
* @author [email protected] (Marcio Endo)
*/
@Pojo
abstract class ElementMethod implements Testable {
abstract String tagName();
abstract ClassName className();
abstract TypeName protoTypeName();
abstract List childList();
ElementMethod() {
}
public static ElementMethodBuilder builder() {
return new ElementMethodBuilderPojo();
}
public static ElementMethod of(Naming naming, MethodInfo methodInfo) {
ClassName className = naming.elementMethodClassName(methodInfo);
return ElementMethod.builder()
.tagName(methodInfo.name())
.className(className)
.protoTypeName(naming.protoTypeName(className))
.childList(Child.listOf(className, methodInfo))
.build();
}
public JavaFile generate() {
String packageName = className().packageName();
TypeSpec typeSpec = type();
return JavaFile.builder(packageName, typeSpec)
.skipJavaLangImports(true)
.build();
}
public TypeSpec type() {
return TypeSpec.classBuilder(className().simpleName())
.addAnnotation(SpecProcessor.ANNOTATION_SPEC)
.addModifiers(Modifier.PUBLIC)
.superclass(protoTypeName())
.addMethods(childrenMethods())
.addMethod(self())
.addTypes(childrenInnerClass())
.build();
}
MethodSpec self() {
return MethodSpec.methodBuilder("self")
.addAnnotation(Override.class)
.returns(className())
.addStatement("return this")
.build();
}
MethodSpec specOverride() {
return MethodSpec.methodBuilder(tagName())
.addAnnotation(Override.class)
.addModifiers(Modifier.PUBLIC)
.returns(className())
.addStatement("return new $T()", className())
.build();
}
private List childrenInnerClass() {
return childList().stream()
.map(Child::innerClass)
.collect(Collectors.toList());
}
private List childrenMethods() {
return childList().stream()
.map(Child::method)
.flatMap(List::stream)
.collect(Collectors.toList());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy