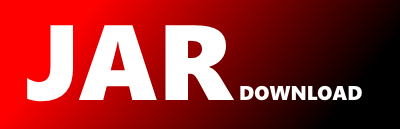
br.com.objectos.ui.html.ProtoMethod Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2015 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.ui.html;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
import javax.lang.model.element.Modifier;
import br.com.objectos.way.pojo.Pojo;
import br.com.objectos.way.testable.Testable;
import br.com.objectos.ui.html.annotation.Attributes;
import br.com.objectos.ui.html.annotation.Void;
import br.com.objectos.way.code.AnnotationInfo;
import br.com.objectos.way.code.Code;
import br.com.objectos.way.code.MethodInfo;
import com.squareup.javapoet.AnnotationSpec;
import com.squareup.javapoet.ClassName;
import com.squareup.javapoet.JavaFile;
import com.squareup.javapoet.MethodSpec;
import com.squareup.javapoet.TypeSpec;
/**
* @author [email protected] (Marcio Endo)
*/
@Pojo
abstract class ProtoMethod implements Testable {
abstract ProtoNaming naming();
abstract String tagName();
abstract String classSimpleName();
abstract ContentModel contentModel();
abstract List attributeAnnotationList();
ProtoMethod() {
}
public static ProtoMethodBuilder builder() {
return new ProtoMethodBuilderPojo();
}
public static ProtoMethod of(ProtoNaming naming, MethodInfo methodInfo) {
String tagName = methodInfo.name();
String name = methodInfo.annotationInfo(br.com.objectos.ui.html.annotation.ClassName.class)
.flatMap(annotationInfo -> annotationInfo.stringValue("value"))
.orElse(Code.upperCaseFirstChar(tagName));
Optional maybeAttributes = methodInfo.annotationInfo(Attributes.class);
return ProtoMethod.builder()
.naming(naming)
.tagName(tagName)
.classSimpleName(name + "Proto")
.contentModel(methodInfo.hasAnnotation(Void.class)
? ContentModel.VOID
: ContentModel.NON_VOID)
.attributeAnnotationList(AttributeAnnotation.list(maybeAttributes))
.build();
}
public JavaFile generate() {
return naming().generate(type());
}
public TypeSpec type() {
return TypeSpec.classBuilder(classSimpleName())
.addAnnotation(ProtoSpecProcessor.ANNOTATION_SPEC)
.addAnnotation(tagNameAnnotation())
.addModifiers(Modifier.ABSTRACT)
.addTypeVariable(naming().protoTypeVariableName())
.superclass(naming().superclassTypeName())
.addMethod(constructor())
.addMethods(attributes())
.build();
}
private List attributes() {
return attributeAnnotationList().stream()
.map(annotation -> annotation.setter(naming().typeVariableName()))
.collect(Collectors.toList());
}
private MethodSpec constructor() {
return MethodSpec
.constructorBuilder()
.addModifiers(Modifier.PUBLIC)
.addStatement("super($S, $T.$L)", tagName(), ClassName.get(ContentModel.class), contentModel().name())
.build();
}
private AnnotationSpec tagNameAnnotation() {
return AnnotationSpec.builder(TagName.class)
.addMember("value", "$S", tagName())
.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy