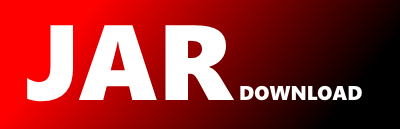
br.com.objectos.comuns.assincrono.SecretariaAbstrata Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2011 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.comuns.assincrono;
/**
* @author [email protected] (Marcio Endo)
*/
public abstract class SecretariaAbstrata implements Secretaria {
private final Agenda agenda;
private final AnotadorDeProgresso anotadorDeProgresso;
private final ServicoAssincrono servicoAssincrono;
protected SecretariaAbstrata(Agenda agenda,
AnotadorDeProgresso anotadorDeProgresso,
ServicoAssincrono servicoAssincrono) {
this.agenda = agenda;
this.anotadorDeProgresso = anotadorDeProgresso;
this.servicoAssincrono = servicoAssincrono;
}
@Override
public Agendamento agendar(Identificador identificador,
Configuracao configuracao) {
T objeto = buscarPor(identificador);
if (objeto == null || configuracao.isForcar()) {
return agendarSeNecessario(identificador, configuracao);
} else {
return new AgendamentoImediato(identificador, objeto, configuracao);
}
}
protected abstract T buscarPor(Identificador identificador);
protected abstract Agendamento novoAgendamento(
Identificador identificador, Configuracao configuracao);
private Agendamento agendarSeNecessario(Identificador identificador,
Configuracao configuracao) {
Agendamento novoAgendamento = novoAgendamento(identificador,
configuracao);
Agendamento existente = agenda.obterAgendamentoDe(identificador);
if (existente != null) {
if (existente.limparErroDeExecucao()) {
// TODO isto provavelmente deveria ser uma op. atômica
anotadorDeProgresso.iniciarProgresso(identificador);
anotadorDeProgresso.anotarProgresso(identificador, "Agendamento feito");
existente = servicoAssincrono.enviar(existente);
}
return existente;
} else {
Agendamento agendamento = agenda.agendarSeNecessario(novoAgendamento);
if (agendamento == null) {
// TODO isto provavelmente deveria ser uma op. atômica
anotadorDeProgresso.iniciarProgresso(identificador);
anotadorDeProgresso.anotarProgresso(identificador, "Agendamento feito");
agendamento = servicoAssincrono.enviar(novoAgendamento);
}
return agendamento;
}
}
private static class AgendamentoImediato implements Agendamento {
private final Identificador identificador;
private final T objeto;
private final Configuracao configuracao;
public AgendamentoImediato(Identificador identificador, T objeto,
Configuracao configuracao) {
this.identificador = identificador;
this.objeto = objeto;
this.configuracao = configuracao;
}
@Override
public Agendamento atualizar(Atualizacoes atualizacoes) {
return this;
}
@Override
public Identificador getIdentificador() {
return identificador;
}
@SuppressWarnings("unchecked")
@Override
public C getConfiguracao() {
return (C) configuracao;
}
@Override
public Erro getErro() {
return null;
}
@Override
public boolean isErroDuranteExecucao() {
return false;
}
@Override
public boolean limparErroDeExecucao() {
return false;
}
@Override
public boolean isResultadoDisponivel() throws ExcecaoDeAgendamento {
return true;
}
@Override
public T obterOuEsperar() throws ExcecaoDeAgendamento {
return objeto;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy