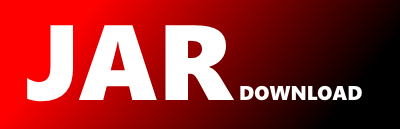
br.com.objectos.way.base.io.DataFile Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2013 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.base.io;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.nio.channels.Channels;
import java.nio.channels.ReadableByteChannel;
import java.util.List;
import org.joda.time.DateTime;
import br.com.objectos.way.base.zip.WayZip;
import br.com.objectos.way.base.zip.WayZipEntry;
import br.com.objectos.way.base.zip.WayZipException;
import br.com.objectos.way.base.zip.WayZips;
import br.com.objectos.core.io.Directory;
import br.com.objectos.core.io.DirectoryFind.Exec;
import com.google.common.collect.ImmutableList;
/**
* @author [email protected] (Marcio Endo)
*/
public class DataFile extends DataFileMeta {
private DataFile(Directory directory, String name) {
super(directory, name);
}
private DataFile(File file) {
super(file);
}
public static DataFile at(Directory directory, String name) {
return new DataFile(directory, name);
}
public static DataFile of(File file) {
return new DataFile(file);
}
public static DataFile withExt(Directory directory, String ext) {
DateTime now = new DateTime();
String filename = String.format("%s.%s", now.toString("yyyy-MM-dd-hhmmssSSS"), ext);
return new DataFile(directory, filename);
}
public static Exec toDataFileExec() {
return ToDataFileExec.INSTANCE;
}
public DataFileMeta metaOf(String ext) {
String filename = String.format("%s.%s", name, ext);
return new DataFileMeta(directory, filename);
}
public List unzip() {
DataFileLog log = DataFileLog.of(this);
try {
WayZip zip = WayZips.unzip(file);
for (WayZipEntry error : zip.getErrors()) {
log.error("Could not unzip entry %s", error.getIOException(), error.getName());
}
return zip.open();
} catch (WayZipException e) {
log.error("Could not open as a ZipFile: %s", e, getPath());
return ImmutableList.of();
}
}
void copyFrom(InputStream is) {
ReadableByteChannel rbc = Channels.newChannel(is);
DataFileOutputStream os = toDataFileOutputStream();
os.transferFrom(rbc);
}
private DataFileOutputStream toDataFileOutputStream() {
try {
FileOutputStream os = new FileOutputStream(file);
return DataFileOutputStream.valid(this, os);
} catch (FileNotFoundException e) {
return DataFileOutputStream.invalid(this, e);
}
}
private enum ToDataFileExec implements Exec {
INSTANCE;
@Override
public DataFile apply(File file) {
return new DataFile(file);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy