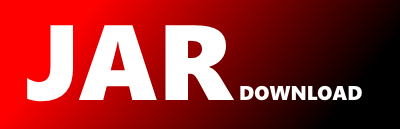
br.com.objectos.way.base.log.AbstractMethodLogger Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2014 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.way.base.log;
import static com.google.common.collect.Lists.newArrayListWithCapacity;
import static com.google.common.collect.Lists.transform;
import java.util.ArrayList;
import java.util.List;
import org.aopalliance.intercept.MethodInterceptor;
import org.aopalliance.intercept.MethodInvocation;
import com.google.common.base.Stopwatch;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.Iterables;
import com.google.inject.Provider;
/**
* @author [email protected] (Marcio Endo)
*/
public abstract class AbstractMethodLogger implements MethodInterceptor {
private final Provider processors;
public AbstractMethodLogger(Provider processors) {
this.processors = processors;
}
@Override
public final Object invoke(MethodInvocation invocation) throws Throwable {
return new Invoker(invocation).invoke();
}
protected void onInvoke(OnInvoke onInvoke) {
onInvoke.debug()
.addClassName()
.add(".")
.addMethodName()
.add("() :>> ")
.addArgs();
}
protected void onReturn(OnReturn onReturn) {
}
protected void onThrowable(OnThrowable onThrowable) {
onThrowable.error()
.addClassName()
.add(".")
.addMethodName()
.add("() :threw an exception")
.addStrackTrace();
}
private void onEnd(OnEnd onEnd) {
onEnd.debug()
.addClassName()
.add(".")
.addMethodName()
.add("() :<< in ")
.addStopwatch();
}
private class Invoker {
private final MethodInvocation invocation;
private final Stopwatch stopwatch;
private final ArrayList phases;
public Invoker(MethodInvocation invocation) {
this.invocation = invocation;
stopwatch = Stopwatch.createStarted();
phases = newArrayListWithCapacity(3);
}
public Object invoke() throws Throwable {
try {
return tryToInvoke();
} catch (Throwable t) {
throw processAndRethrow(t);
} finally {
try {
OnEnd onEnd = new OnEnd(invocation, stopwatch);
phases.add(onEnd);
onEnd(onEnd);
} finally {
process();
}
}
}
private Object tryToInvoke() throws Throwable {
OnInvoke onInvoke = new OnInvoke(invocation);
phases.add(onInvoke);
onInvoke(onInvoke);
Object returned = invocation.proceed();
OnReturn onReturn = new OnReturn(invocation, returned);
phases.add(onReturn);
onReturn(onReturn);
return returned;
}
private Throwable processAndRethrow(Throwable t) {
OnThrowable onThrowable = new OnThrowable(invocation, t);
phases.add(onThrowable);
onThrowable(onThrowable);
return t;
}
private void process() {
LogProcessor processor;
processor = processors.get();
List>> listzes;
listzes = transform(phases, AbstractPhase.TO_LOG_BUILDER);
Iterable> builders;
builders = Iterables.concat(listzes);
Iterable _logs;
_logs = Iterables.transform(builders, LogBuilder.TO_LOG);
List logs;
logs = ImmutableList.copyOf(_logs);
for (Log log : logs) {
processor.process(log);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy