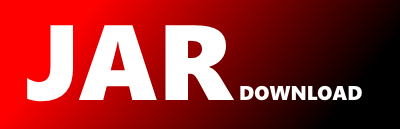
br.com.objectos.code.AnnotationInfoAnnotationMirror Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2015 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.code;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
import javax.lang.model.element.AnnotationMirror;
import javax.lang.model.element.Element;
import javax.lang.model.element.TypeElement;
import javax.lang.model.type.DeclaredType;
import javax.lang.model.type.TypeKind;
import br.com.objectos.lazy.Lazy;
/**
* @author [email protected] (Marcio Endo)
*/
class AnnotationInfoAnnotationMirror extends AnnotationInfo {
private final ProcessingEnvironmentWrapper processingEnv;
private final AnnotationMirror annotation;
private final PackageInfo packageInfo;
private final AccessInfo accessInfo;
private final String name;
private final Lazy annotationValueInfoMap = new LazyAnnotationValueInfoMap();
private final Lazy> enclosingTypeInfo = new LazyEnclosingTypeInfo();
private final Lazy> annotationInfoList = new LazyAnnotationInfoList();
private final Lazy simpleTypeInfo = new LazySimpleTypeInfo();
AnnotationInfoAnnotationMirror(ProcessingEnvironmentWrapper processingEnv,
AnnotationMirror annotation,
PackageInfo packageInfo,
AccessInfo accessInfo,
String name) {
this.processingEnv = processingEnv;
this.annotation = annotation;
this.packageInfo = packageInfo;
this.accessInfo = accessInfo;
this.name = name;
}
@Override
public List annotationInfoList() {
return annotationInfoList.get();
}
@Override
public Optional enclosingSimpleTypeInfo() {
return enclosingTypeInfo.get();
}
@Override
public PackageInfo packageInfo() {
return packageInfo;
}
@Override
public SimpleTypeInfo simpleTypeInfo() {
return simpleTypeInfo.get();
}
@Override
AccessInfo accessInfo() {
return accessInfo;
}
@Override
AnnotationValueInfoMap annotationValueInfoMap() {
return annotationValueInfoMap.get();
}
@Override
String name() {
return name;
}
private Element element() {
DeclaredType annotationType = annotation.getAnnotationType();
return annotationType.asElement();
}
private class LazyAnnotationValueInfoMap extends Lazy {
@Override
protected AnnotationValueInfoMap compute() {
List list = AnnotationValueWrapper.wrapAll(processingEnv, annotation)
.map(AnnotationValueWrapper::toAnnotationValueInfo)
.collect(Collectors.toList());
return AnnotationValueInfoMap.mapOf(list);
}
}
private class LazyEnclosingTypeInfo extends Lazy> {
@Override
protected Optional compute() {
Optional res = Optional.empty();
Element enclosingElement = element().getEnclosingElement();
if (Apt.isType(enclosingElement)) {
TypeElement typeElement = TypeElement.class.cast(enclosingElement);
SimpleTypeInfo simpleTypeInfo = SimpleTypeInfoTypeElement.wrap(processingEnv, typeElement);
res = Optional.of(simpleTypeInfo);
}
return res;
}
}
private class LazyAnnotationInfoList extends Lazy> {
@Override
protected List compute() {
List list = Collections.emptyList();
if (!packageInfo().isJavaLang() && !packageInfo().hasName("java.lang.annotation")) {
list = AnnotationMirrorWrapper.wrapAllAndList(processingEnv, element());
}
return list;
}
}
private class LazySimpleTypeInfo extends Lazy {
@Override
protected SimpleTypeInfo compute() {
DeclaredType type = annotation.getAnnotationType();
if (TypeKind.ERROR.equals(type.getKind())) {
throw new CodeGenerationIncompleteException();
}
return SimpleTypeInfoTypeMirror.wrap(processingEnv, type);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy