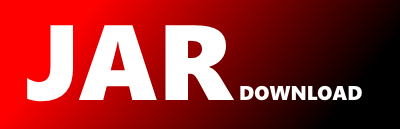
br.com.objectos.code.Apt Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2014 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.code;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
import javax.lang.model.element.Element;
import javax.lang.model.element.ElementKind;
import javax.lang.model.element.Modifier;
import javax.lang.model.element.PackageElement;
import javax.lang.model.element.TypeElement;
import javax.lang.model.type.TypeKind;
import javax.lang.model.type.TypeMirror;
/**
* @author [email protected] (Marcio Endo)
*/
class Apt {
private Apt() {
}
public static boolean isClass(Element element) {
return ElementKind.CLASS.equals(element.getKind());
}
public static boolean isType(Element element) {
return ElementIsType.TYPE_SET.contains(element.getKind());
}
public static AccessInfo accessInfoOf(Element element) {
Set modifiers = element.getModifiers();
return AccessInfo.fromJdk(modifiers)
.orElse(AccessInfo.DEFAULT);
}
public static InterfaceInfoMap interfaceInfoMapOf(ProcessingEnvironmentWrapper processingEnv, TypeElement element) {
List list = element.getInterfaces().stream()
.map(type -> TypeInfoTypeMirror.wrap(processingEnv, type))
.map(TypeInfo::toInterfaceInfo)
.filter(Optional::isPresent)
.map(Optional::get)
.collect(Collectors.toList());
return InterfaceInfoMap.mapOf(list);
}
public static InterfaceInfoMap interfaceInfoMapOf(ProcessingEnvironmentWrapper processingEnv, TypeMirror type) {
InterfaceInfoMap map = InterfaceInfoMap.mapOf();
Element element = processingEnv.asElement(type);
if (isType(element)) {
TypeElement typeElement = TypeElement.class.cast(element);
map = interfaceInfoMapOf(processingEnv, typeElement);
}
return map;
}
public static List methodInfoListOf(
ProcessingEnvironmentWrapper processingEnv, TypeElement element, TypeParameterInfoMap typeParameterInfoMap) {
List extends Element> elements = processingEnv.getEnclosedElements(element);
return elements.stream()
.filter(ElementIsKind.get(ElementKind.METHOD))
.map(ElementToMethodInfo.get(processingEnv, typeParameterInfoMap))
.collect(Collectors.toList());
}
public static List methodInfoListOf(ProcessingEnvironmentWrapper processingEnv, TypeMirror type) {
List list = Collections.emptyList();
Element element = processingEnv.asElement(type);
if (isType(element)) {
TypeElement typeElement = TypeElement.class.cast(element);
TypeParameterInfoMap typeParameterInfoMap = processingEnv.getTypeParameterInfoMapOf(typeElement);
list = methodInfoListOf(processingEnv, typeElement, typeParameterInfoMap);
}
return list;
}
public static PackageInfo packageInfoOf(ProcessingEnvironmentWrapper processingEnv, Element element) {
PackageElement packageElement = processingEnv.getPackageOf(element);
return PackageInfoPackageElement.wrap(processingEnv, packageElement);
}
public static Optional superTypeInfoOf(ProcessingEnvironmentWrapper processingEnv, TypeElement element) {
ElementKind kind = element.getKind();
if (!ElementKind.CLASS.equals(kind)) {
return Optional.empty();
}
TypeMirror superTypeMirror = element.getSuperclass();
TypeKind superKind = superTypeMirror.getKind();
if (TypeKind.NONE.equals(superKind)) {
return Optional.empty();
}
String qualifiedName = processingEnv.getQualifiedName(superTypeMirror);
if ("java.lang.Object".equals(qualifiedName)) {
return Optional.empty();
}
SuperTypeInfo superTypeInfo = SuperTypeInfoTypeMirror.wrap(processingEnv, superTypeMirror);
return Optional.ofNullable(superTypeInfo);
}
public static Optional superTypeInfoOf(ProcessingEnvironmentWrapper processingEnv, TypeMirror type) {
Element element = processingEnv.asElement(type);
return superTypeInfoOf(processingEnv, element);
}
private static Optional superTypeInfoOf(ProcessingEnvironmentWrapper processingEnv, Element element) {
Optional res = Optional.empty();
if (isClass(element)) {
TypeElement typeElement = TypeElement.class.cast(element);
res = superTypeInfoOf(processingEnv, typeElement);
}
return res;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy