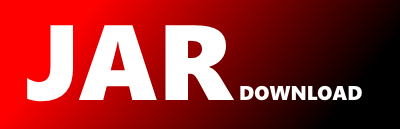
br.com.objectos.code.MethodInfoExecutableElement Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2015 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.code;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import javax.lang.model.element.Element;
import javax.lang.model.element.ExecutableElement;
import javax.lang.model.element.Modifier;
import javax.lang.model.element.TypeElement;
import javax.lang.model.type.TypeMirror;
import br.com.objectos.lazy.Lazy;
/**
* @author [email protected] (Marcio Endo)
*/
class MethodInfoExecutableElement extends MethodInfo {
private final ProcessingEnvironmentWrapper processingEnv;
private final ExecutableElement element;
private final MethodInfoExecutableElementCore core;
private final Lazy> annotationInfoList = new LazyAnnotationInfoList();
private final Lazy> enclosingTypeInfo = new LazyEnclosingTypeInfo();
private final Lazy> parameterInfoList = new LazyParameterInfoList();
private final Lazy returnTypeInfo = new LazyReturnTypeInfo();
private MethodInfoExecutableElement(ProcessingEnvironmentWrapper processingEnv,
ExecutableElement element,
MethodInfoExecutableElementCore core) {
this.processingEnv = processingEnv;
this.element = element;
this.core = core;
}
public static MethodInfoExecutableElement wrap(
ProcessingEnvironmentWrapper processingEnv,
ExecutableElement element,
TypeParameterInfoMap typeParameterInfoMap) {
MethodInfoExecutableElementCore core = new CoreBuilder(element, typeParameterInfoMap).build();
return new MethodInfoExecutableElement(processingEnv, element, core);
}
@Override
public AccessInfo accessInfo() {
return core.accessInfo;
}
@Override
public List annotationInfoList() {
return annotationInfoList.get();
}
@Override
public void compilationError(String message) {
processingEnv.compilationError(message, element);
}
@Override
public String name() {
return core.name;
}
@Override
public SimpleTypeInfo returnTypeInfo() {
return returnTypeInfo.get();
}
@Override
public boolean varargs() {
return core.varargs;
}
@Override
Optional enclosingTypeInfo() {
return enclosingTypeInfo.get();
}
@Override
Set modifierInfoSet() {
return core.modifierInfoSet;
}
@Override
List parameterInfoList() {
return parameterInfoList.get();
}
private TypeParameterInfoMap typeParameterInfoMap() {
return core.typeParameterInfoMap;
}
private static class CoreBuilder {
private final ExecutableElement element;
private final TypeParameterInfoMap typeParameterInfoMap;
public CoreBuilder(ExecutableElement element, TypeParameterInfoMap typeParameterInfoMap) {
this.element = element;
this.typeParameterInfoMap = typeParameterInfoMap;
}
public MethodInfoExecutableElementCore build() {
return new MethodInfoExecutableElementCore(
typeParameterInfoMap,
accessInfo(),
modifierInfoSet(),
name(),
element.isVarArgs());
}
private String name() {
return element.getSimpleName()
.toString();
}
private AccessInfo accessInfo() {
return Apt.accessInfoOf(element);
}
private Set modifierInfoSet() {
Set modifiers = element.getModifiers();
return ModifierInfo.fromJdk(modifiers);
}
}
private class LazyAnnotationInfoList extends Lazy> {
@Override
protected List compute() {
return AnnotationMirrorWrapper.wrapAllAndList(processingEnv, element);
}
}
private class LazyEnclosingTypeInfo extends Lazy> {
@Override
protected Optional compute() {
Optional res = Optional.empty();
Element enclosingElement = element.getEnclosingElement();
if (TypeElement.class.isInstance(enclosingElement)) {
TypeElement typeElement = TypeElement.class.cast(enclosingElement);
TypeInfoTypeElement typeInfo = TypeInfoTypeElement.wrap(processingEnv, typeElement);
res = Optional.of(typeInfo);
}
return res;
}
}
private class LazyReturnTypeInfo extends Lazy {
@Override
protected SimpleTypeInfo compute() {
TypeMirror returnType = element.getReturnType();
return SimpleTypeInfoTypeMirror.wrap(processingEnv, returnType);
}
}
private class LazyParameterInfoList extends Lazy> {
@Override
protected List compute() {
return ParameterInfoVariableElement.wrapAll(processingEnv, element, typeParameterInfoMap());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy