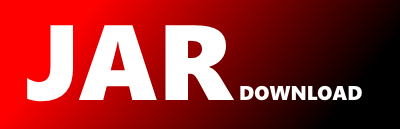
br.com.objectos.code.AbstractTestingProcessor Maven / Gradle / Ivy
/*
* Copyright 2015 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.code;
import java.lang.annotation.Annotation;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Function;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import javax.annotation.processing.AbstractProcessor;
import javax.annotation.processing.RoundEnvironment;
import javax.lang.model.SourceVersion;
import javax.lang.model.element.Element;
import javax.lang.model.element.TypeElement;
import javax.lang.model.util.ElementFilter;
import javax.tools.JavaFileObject;
import com.google.common.collect.ImmutableSet;
import com.google.common.truth.Truth;
import com.google.testing.compile.JavaFileObjects;
import com.google.testing.compile.JavaSourcesSubjectFactory;
/**
* @author [email protected] (Marcio Endo)
*/
public abstract class AbstractTestingProcessor extends AbstractProcessor {
private Map qualifiedNameMap;
public AbstractTestingProcessor() {
}
public static Map loadAll(
Supplier supplier,
String basePath,
String... paths) {
List objectList = Stream.of(paths)
.map((String input) -> JavaFileObjects.forResource(basePath + "/" + input))
.collect(Collectors.toList());
T processor = supplier.get();
Truth.assertAbout(JavaSourcesSubjectFactory.javaSources())
.that(objectList)
.processedWith(processor)
.compilesWithoutError();
return processor.qualifiedNameMap();
}
@Override
public Set getSupportedAnnotationTypes() {
return ImmutableSet.of(annotationType().getName());
}
@Override
public SourceVersion getSupportedSourceVersion() {
return SourceVersion.latestSupported();
}
@Override
public boolean process(Set extends TypeElement> annotations, RoundEnvironment roundEnv) {
if (annotations.isEmpty()) {
return true;
}
Set extends Element> roundSet = roundEnv.getElementsAnnotatedWith(annotationType());
qualifiedNameMap = ElementFilter.typesIn(roundSet)
.stream()
.map(typeElementMapper())
.filter(this::filter)
.collect(Collectors.toMap(TypeInfo::qualifiedName, Function.identity()));
return true;
}
protected abstract Class extends Annotation> annotationType();
protected boolean filter(TypeInfo typeInfo) {
return true;
}
protected abstract Function typeElementMapper();
public Stream typesAnnotatedWith(RoundEnvironment roundEnv) {
Set extends Element> elementSet;
elementSet = roundEnv.getElementsAnnotatedWith(annotationType());
Set typeSet;
typeSet = ElementFilter.typesIn(elementSet);
return typeSet.stream();
}
Map qualifiedNameMap() {
return qualifiedNameMap;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy