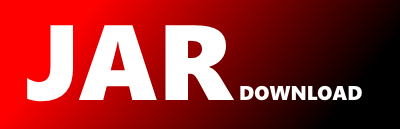
br.com.objectos.code.ParameterInfoFake Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2014 Objectos, Fábrica de Software LTDA.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package br.com.objectos.code;
/**
* @author [email protected] (Marcio Endo)
*/
class ParameterInfoFake {
public static final ParameterInfo INT_ARRAY_I = builder()
.simpleTypeInfo(SimpleTypeInfoFake.INT_ARRAY)
.name("i")
.build();
public static final ParameterInfo BOOLEAN_STEP1 = builder()
.simpleTypeInfo(SimpleTypeInfoFake.BOOLEAN)
.name("step1")
.build();
public static final ParameterInfo INT_AMMOUNT = builder()
.simpleTypeInfo(SimpleTypeInfoFake.INT)
.name("ammount")
.build();
public static final ParameterInfo INT_AMMOUNT_1_2 = builder()
.index(1, 2)
.simpleTypeInfo(SimpleTypeInfoFake.INT)
.name("ammount")
.build();
public static final ParameterInfo INT_ID = builder()
.simpleTypeInfo(SimpleTypeInfoFake.INT)
.name("id")
.build();
public static final ParameterInfo INT_ID_0_2 = builder()
.index(0, 2)
.simpleTypeInfo(SimpleTypeInfoFake.INT)
.name("id")
.build();
public static final ParameterInfo INT_ID_1_2 = builder()
.index(1, 2)
.simpleTypeInfo(SimpleTypeInfoFake.INT)
.name("id")
.build();
public static final ParameterInfo ITERABLE_ANY_EXTENDS_SIMPLE_ITERABLE = builder()
.simpleTypeInfo(SimpleTypeInfoFake.ITERABLE_ANY_EXTENDS_SIMPLE)
.name("iterable")
.build();
public static final ParameterInfo LOCAL_DATE_DATE = builder()
.simpleTypeInfo(SimpleTypeInfoFake.LOCAL_DATE__)
.name("date")
.build();
public static final ParameterInfo LOCAL_DATE_DATE_0_2 = builder()
.index(0, 2)
.simpleTypeInfo(SimpleTypeInfoFake.LOCAL_DATE__)
.name("date")
.build();
public static final ParameterInfo OBJECT_OBJ = builder()
.simpleTypeInfo(SimpleTypeInfoFake.OBJECT)
.name("obj")
.build();
public static final ParameterInfo OBJECT_ARG = builder()
.simpleTypeInfo(SimpleTypeInfoFake.OBJECT)
.name("arg0")
.build();
public static final ParameterInfo OBJECT_ARRAY_VARARGS = builder()
.simpleTypeInfo(SimpleTypeInfoFake.OBJECT_ARRAY)
.name("varargs")
.build();
public static final ParameterInfo OBJECT_ARRAY_VARARGS_1_2 = builder()
.index(1, 2)
.simpleTypeInfo(SimpleTypeInfoFake.OBJECT_ARRAY)
.name("varargs")
.build();
public static final ParameterInfo OBJECT_FIRST = builder()
.index(0, 2)
.simpleTypeInfo(SimpleTypeInfoFake.OBJECT)
.name("first")
.build();
public static final ParameterInfo OBJECT_NOT_NULL = builder()
.simpleTypeInfo(SimpleTypeInfoFake.OBJECT)
.name("notNull")
.annotationInfo(AnnotationInfoFake.NOT_NULL)
.build();
public static final ParameterInfo OPTIONAL_ANY_EXTENDS_GENERIC = builder()
.simpleTypeInfo(SimpleTypeInfoFake.OPTIONAL_ANY_EXTENDS_GENERIC)
.name("maybeGeneric")
.build();
public static final ParameterInfo POJO_THAT = builder()
.simpleTypeInfo(SimpleTypeInfoFake.POJO)
.name("that")
.build();
public static final ParameterInfo POJO_INNER_INNER = builder()
.simpleTypeInfo(SimpleTypeInfoFake.POJO_INNER)
.name("inner")
.build();
public static final ParameterInfo POJO_GENERIC_RAW_ARG = builder()
.simpleTypeInfo(SimpleTypeInfoFake.POJO_GENERIC_RAW)
.name("arg0")
.build();
public static final ParameterInfo SET_STRING_NAMESET = builder()
.simpleTypeInfo(SimpleTypeInfoFake.SET_STRING)
.name("nameSet")
.build();
public static final ParameterInfo SOURCE_FILE_ARG = builder()
.simpleTypeInfo(SimpleTypeInfoFake.SOURCE_FILE)
.name("arg0")
.build();
public static final ParameterInfo SOURCE_FILE_THAT = builder()
.simpleTypeInfo(SimpleTypeInfoFake.SOURCE_FILE)
.name("that")
.build();
public static final ParameterInfo STRING_ARRAY = builder()
.simpleTypeInfo(SimpleTypeInfoFake.STRING_ARRAY)
.name("stringArray")
.build();;
public static final ParameterInfo STRING_ARRAY_S = builder()
.simpleTypeInfo(SimpleTypeInfoFake.STRING_ARRAY)
.name("s")
.build();
public static final ParameterInfo STRING_FIRST = builder()
.simpleTypeInfo(SimpleTypeInfoFake.STRING)
.name("first")
.build();
public static final ParameterInfo STRING_NAME = builder()
.simpleTypeInfo(SimpleTypeInfoFake.STRING)
.name("name")
.build();
public static final ParameterInfo STRING_NAME_0_2 = builder()
.index(0, 2)
.simpleTypeInfo(SimpleTypeInfoFake.STRING)
.name("name")
.build();
public static final ParameterInfo STRING_NAME_ANNOTATED = builder()
.index(1, 2)
.simpleTypeInfo(SimpleTypeInfoFake.STRING)
.name("name")
.annotationInfo(AnnotationInfoFake.PARAMETER_ANNOTATION_LEVEL_2)
.build();
public static final ParameterInfo STRING_STEP0 = builder()
.simpleTypeInfo(SimpleTypeInfoFake.STRING)
.name("step0")
.build();
private ParameterInfoFake() {
}
private static ParameterInfoFakeBuilder builder() {
return new ParameterInfoFakeBuilder();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy